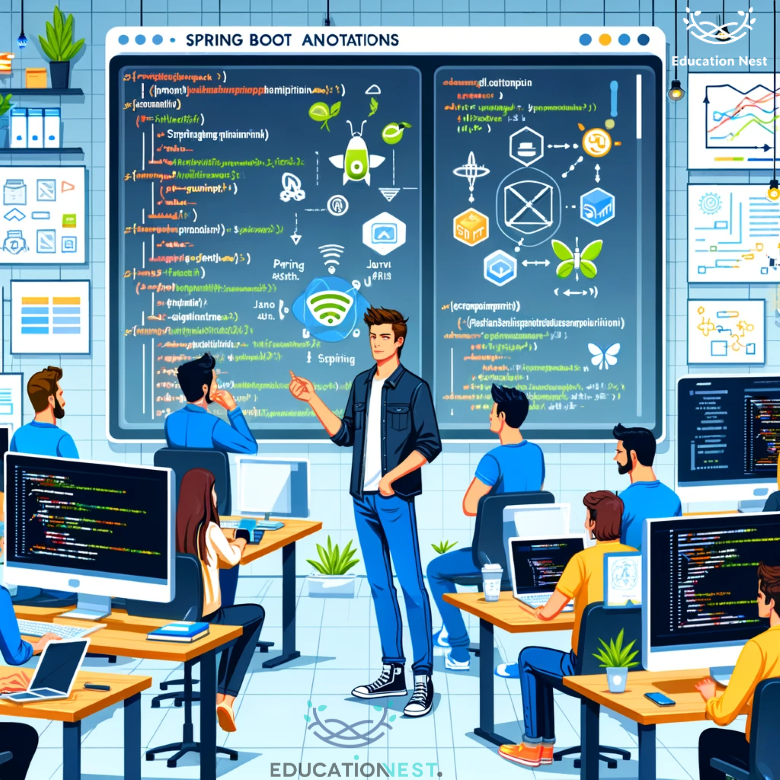
Welcome to the world of Spring Boot, a powerful and versatile framework that simplifies the bootstrapping and development of new Spring applications. In this blog, we’re going to delve deep into the realm of Spring Boot annotations, an essential aspect that makes coding in Spring so efficient and straightforward. Whether you’re a seasoned developer or new to the Spring ecosystem, understanding Spring Boot annotations is crucial. This comprehensive guide will not only provide a detailed Spring Boot annotations list but will also serve as a Spring Boot annotations cheat sheet. We’ll explore how ‘autowired in Spring Boot‘ works and prepare you to tackle Spring Boot annotations interview questions with confidence. So, buckle up, and let’s embark on this journey to mastering Spring Boot annotations.
Understanding Spring Boot Annotations
Spring Boot annotations are the backbone of the framework, acting as metadata to provide data about your code. Before diving into the extensive Spring Boot annotations list, let’s grasp why these annotations are game-changers. They streamline configuration and eliminate the need for boilerplate code, making your development process sleek and efficient. The power of ‘autowired in Spring Boot‘ lies in its ability to automatically inject dependencies, ensuring that your components are seamlessly connected without manual intervention.
Spring Boot Annotations Cheat Sheet
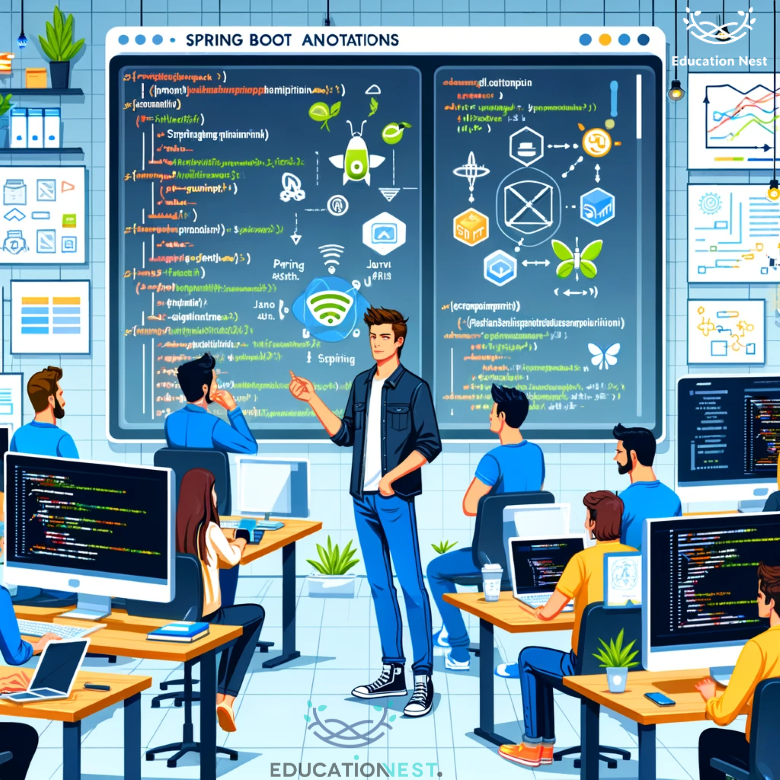
Navigating through the myriad of Spring Boot annotations can be daunting. Hence, this section serves as a cheat sheet, offering a quick reference to the most commonly used annotations in Spring Boot.
Below is a list of 40 Spring Boot annotations, each accompanied by a brief description:
@SpringBootApplication: Serves as a convenient alias for three primary annotations: @Configuration, @EnableAutoConfiguration, and @ComponentScan. It sets up a complete auto-configured Spring application.
@Autowired: Enables automatic dependency injection. It lets Spring resolve and inject collaborating beans into your bean.
@Component: Marks a java class as a bean, a Spring managed component. It’s the base annotation for stereotype annotations like @Service, @Repository, and @Controller.
@Service: Indicates that an annotated class is a service class. It’s used to mark a bean as a service provider.
@Repository: Denotes that a class is a repository, which is an abstraction of data access and storage.
@Controller: Marks a class as a web request handler, it’s typically used in combination with @RequestMapping.
@RestController: A convenience annotation that combines @Controller and @ResponseBody. It indicates that the return value of the methods should be bound to the web response body.
@RequestMapping: Maps HTTP requests to handler methods of MVC and REST controllers.
@GetMapping, @PostMapping, @PutMapping, @DeleteMapping, @PatchMapping: These annotations are specialized versions of @RequestMapping. They act as shortcuts for specifying the HTTP method in a request mapping.
@EnableAutoConfiguration: Tells Spring Boot to start adding beans based on classpath settings, other beans, and various property settings.
@ConfigurationProperties: Allows binding and validating external configurations (e.g., from application.properties) to a configuration class.
@Configuration: Indicates that a class declares one or more @Bean methods and may be processed by the Spring container to generate bean definitions and service requests for those beans at runtime.
@Bean: Indicates that a method produces a bean to be managed by the Spring container.
@Profile: Indicates that a component is eligible for registration when one or more specified profiles are active.
@Scheduled: Used for marking a method as a scheduled task, defining when it should be run.
@EnableScheduling: Enables Spring’s scheduled task execution capability.
@EnableAsync: Enables Spring’s asynchronous method execution capability.
@Async: Indicates that a method should be executed asynchronously.
@Transactional: Describes transaction attributes on a method or class.
@EnableTransactionManagement: Enables Spring’s annotation-driven transaction management capability.
@PathVariable: Used for extracting values from the URI. It’s most often used in RESTful web services, where the URL contains parameters.
@RequestParam: Used to extract query parameters from the URL in the controller methods.
@RequestBody: Indicates that a method parameter should be bound to the body of the HTTP request.
@ResponseBody: Indicates that the return type of the method should be used as the response body of the request.
@RestControllerAdvice: A convenience annotation that is itself annotated with @ControllerAdvice and @ResponseBody, used for global exception handling in RESTful services.
@ExceptionHandler: Used for handling exceptions in specific handler classes or handler methods.
@CrossOrigin: Enables cross-origin resource sharing (CORS) on the annotated method or class.
@SessionAttribute: Used for binding a method parameter or method return value to a session attribute.
@ModelAttribute: Binds a method parameter or method return value to a named model attribute and then exposes it to a web view.
@Valid: Used for validating a model attribute. It’s often used in combination with @RequestBody.
@EnableCaching: Enables Spring’s annotation-driven cache management capability.
@Cacheable: Indicates that the result of invoking a method can be cached.
@CacheEvict: Triggers a cache eviction.
@CachePut: Updates the cache without interfering with the method execution.
@Primary: Indicates that a bean should be given preference when multiple candidates are qualified to autowire a single-valued dependency.
@Qualifier: Used along with @Autowired to avoid confusion when multiple instances of a bean type are present.
@Lazy: Indicates that a bean is to be lazily initialized.
@Value: Used for injecting property values into components.
@PropertySource: Used to declare a set of properties (defined in a properties file) in Spring’s Environment.
@Import: Allows for the importation of additional configuration classes.
These annotations are integral to Spring Boot and help in effectively managing the lifecycle of your application components, managing configurations, and handling requests and transactions. Understanding these annotations and their proper usage is key to leveraging the full power of the Spring Boot framework.
This cheat sheet is just the tip of the iceberg. The Spring Boot annotations list is extensive, and understanding each one is pivotal in harnessing the full potential of Spring Boot.
‘Autowired in Spring Boot’ Explained
One cannot stress enough the importance of ‘autowired in Spring Boot.’ This section will unravel the intricacies of this powerful annotation. It eliminates the need for manual wiring, allowing Spring to resolve and inject collaborating beans into your bean. From defining controllers to services, ‘autowired in Spring Boot’ ensures that your components are effortlessly connected, fostering a cohesive and robust application.
@Autowired in Spring Boot is like a helpful assistant that connects different parts of your application together without you having to do much. Imagine you’re building a robot and you need to connect its hand to the arm. Instead of finding tools and doing it yourself, @Autowired magically brings the hand and arm together, making sure they fit perfectly. This is called dependency injection. In technical terms, @Autowired automatically provides the necessary components (like services or repositories) your code needs to work properly. You just tell Spring Boot what you need by marking it with @Autowired, and Spring Boot takes care of the rest. It’s a smart and efficient way to make sure all parts of your application are connected and working together, saving you time and making your code cleaner and easier to manage.
Also Read:
A Perspective on Spring Boot Interview Questions
Tackling Spring Boot Annotations Interview Questions
Interviews can be nerve-wracking, especially when delving into the specifics of Spring Boot annotations. However, equipped with this comprehensive guide, you’re now ready to tackle even the most challenging Spring Boot annotations interview questions. Remember, understanding the purpose and functionality of each annotation is key. Be prepared to discuss how ‘autowired in Spring Boot’ streamlines dependency injection, or how @RestController simplifies the creation of RESTful services.
Conclusion
Embarking on the Spring Boot journey can be exhilarating, with its powerful annotations acting as your compass. This comprehensive Spring Boot annotations list and cheat sheet aims to empower you, offering clarity and confidence as you navigate through your Spring Boot applications. Remember, ‘autowired in Spring Boot‘ is your ally, simplifying dependency management and propelling you towards efficient, clean, and maintainable code. So, whether you’re preparing for an interview or enhancing your Spring Boot prowess, this guide is your steadfast companion in the ever-evolving landscape of Spring Boot.
In closing, remember that each annotation in Spring Boot is a tool designed to streamline your development process, making it more intuitive and enjoyable. Keep exploring, keep learning, and let the power of Spring Boot annotations elevate your projects to new heights. Happy coding!