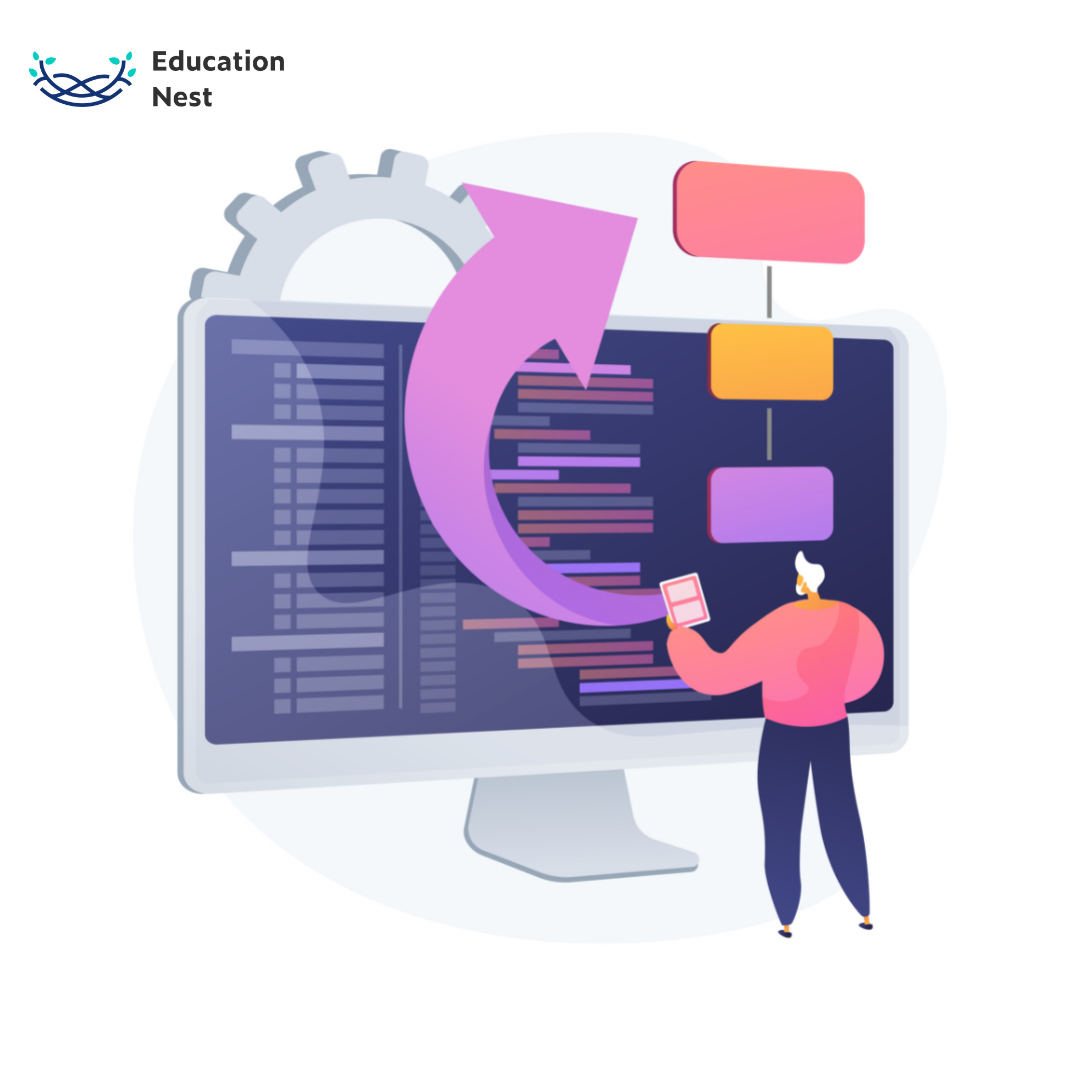
The idea of “objects” is central to object oriented programming (OOP), a way of writing code that divides data and procedures into separate pieces. It’s also a way to keep code from repeating itself unnecessarily while creating clean, reusable code.
Python is a flexible programming language that can be used in several ways, including object-oriented programming (OOP) with objects and classes.
Object-oriented programming courses are a way to model abstract ideas, like cars, and real-world relationships, like those between businesses and their employees or students and teachers. In OOP, software objects are like real-world things. They have their data and can-do things that are already set up.
This article will discuss object-oriented programming (OOP) in Python types and concepts supporting OOP. We have also covered some Python object-oriented programming exercises, which will help at every step of your programming career.
What Exactly is Python Object Oriented Programming?
Object-oriented programming is a way to organize code around the properties and actions of objects.
An object can stand in for a person, giving them a name, age, address, and even the ability to walk, talk, breathe, and run. It could also be an email with a sender, recipient, topic, body, and attachments.
To be precise, object-oriented programming models both abstract ideas like cars and real-world interactions like those between companies and their employees or between students and teachers. OOP turns things in the real world into software objects that have data and abilities.
Procedural programming is another standard paradigm. Like a cookbook, it lists the functions and code blocks that must be run to finish the program.
Object-oriented programming in Python uses objects to store data and organize how the program works.
Let’s start by understanding an example of OOP and how it makes our work easier.
Python’s object oriented programming (OOP) example
This piece of code shows how OOP can be used to make classes with traits that can be inherited and methods that can be changed.
Class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def greeting(self):
print(“Hello, my name is”, self. name, “and I am”, self. age, “years old.”)
class Student(Person):
def __init__(self, name, age, student_id):
super().__init__(name, age)
self.student_id = student_id
def study(self):
print(self.name, “is studying.”)
person1 = Person(“John”, 25)
person1.greeting()
student1 = Student(“Jane”, 20, “1234”)
student1.greeting()
student1.study()
In this example, the classes “person” and “student” are the ones that are used. The name and age of a person define them, and the greeting function makes a message that fits them. The subclass of Person called “Student” now has a new property called “student id” and a new method called “study.” The fact that the object is a student is just written down.
Then, we create an instance of Person and call its greeting method to print a greeting. We also make a student object and give it information like its name, age, and student ID. After that, we call methods like “hello” and “study.”
You Must Watch: 21 Essential DBMS Interview Questions You Need to Know
The basics of object oriented programming with Python is:
A class is a template for making objects with standard properties and operations.
A single instance of a class is called an “object.”
A piece of information that describes some part of an object is called an attribute.
A method is an internal function of a class that can be called an object.
These are used in Python to make reliable and easy-to-maintain apps. OOP makes it possible for programmers to write modular, reusable, and scalable code. This makes it possible for programmers to use the power of modularity, reusability, and scalability.
Object Oriented Programming Concepts
The following are covered by object-oriented programming in Python:
- Inheritance
- Polymorphism
- Encapsulation
- Abstraction
These four are the basis of OOP and are used to create complex and useful programs. With these methods, programmers can make more flexible software, add it, and update it more easily.
Let’s talk about the basic principles of all these methods of OOP:
- Using a concept called “inheritance,” one class can take on the actions and features of another. With this feature, you can make a new class based on a modified version of an existing course.
- Polymorphism means that something can change into many different shapes. In OOP, this means that two objects can have the same name for a method but use different ways to do that method’s work.
- This method, called “encapsulation,” hides the details of how an object works while letting outsiders use its interface. It helps keep sensitive information from getting into the wrong hands.
- The term “abstraction” refers to hiding a system’s complexity while giving the user only the most essential information. Object-oriented programming means making classes that hide the code underneath and have a simple interface.
Object Oriented Programming in Python Tutorial
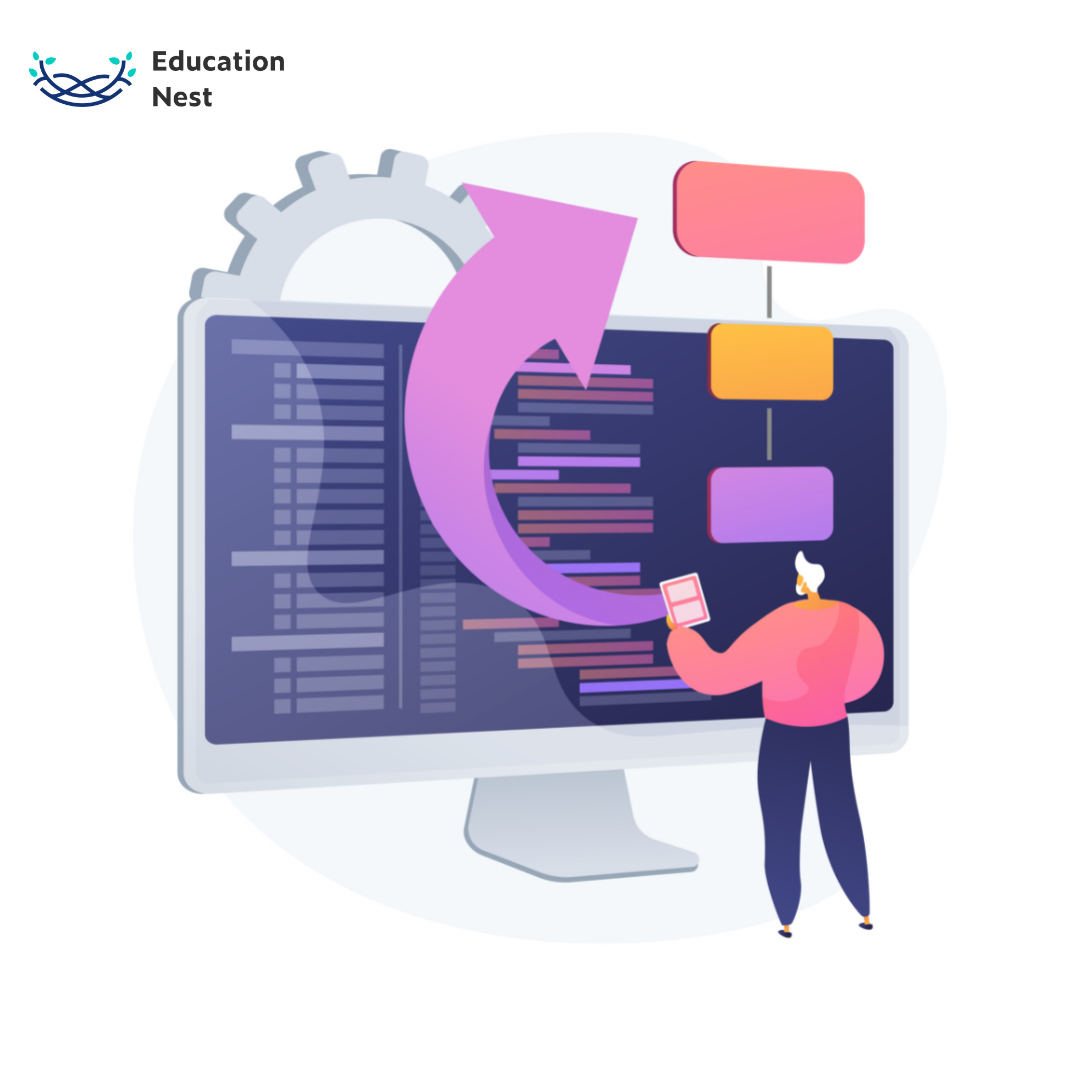
In object-oriented programming, each object has its own traits and ways of acting, and the code is built around these objects.
A person, for example, can be represented by an object with a name, age, and address, as well as by actions like walking, talking, breathing, and sprinting. On the other hand, it can be used in place of an email, with sender, recipient, subject, body, and attachment operations.
Procedural programming is another standard paradigm. It organizes a program, like a recipe, with a series of steps (usually functions and code blocks) that must be done to finish the task.
In Python’s object-oriented programming paradigm, one of the most important things to remember is that objects are at the center of how data is represented and how a program or student program is put together.
Few examples of python object oriented programming exercises
- Write a Python program that imports the built-in array module and shows the module’s namespace.
- Write a Python script to generate a class and show off its associated namespace.
- Write a Python script to instantiate and show the namespace of a given class.
- Write a Student class in Python and give it two attributes: student_name and grades. Change the values of the characteristics of the course and show both the old and new values.
- Define a Python function (). Show the names of the arguments to the process by using their attributes.
Best Way to Learn Object Oriented Programming Python
Python’s OOP is not easy to pick up, but it has many applications and is worth the effort.
Listed below are some suggested methods for learning OOP in Python:
- Get a foundational understanding of Python. Be sure you have a firm knowledge of data types, functions, and control structures before delving into OOP.
- Take the time to learn OOP’s most important ideas, such as encapsulation, inheritance, and polymorphism. Only move forward if you have a firm grasp on what each of these terms implies and how they function.
- Exercise, exercise, and more exercise OOP is a talent that requires practice. Programming with OOP can be intimidating, so starting with the basics and working your way up to the more advanced levels is best.
- Learn Python by perusing its documentation: Python documentation is excellent and gives much information about object-oriented programming. Be sure to get your hands on Python’s official OOP documentation and put what you learn into practice as you go along.
- If you want to learn OOP in Python, you can do it by watching videos or enrolling in online courses. A lot of information on the web can help you learn how to use OOP in Python. Video guides, online courses, scholarly publications, and weblogs are all great resources for learning more.
- Project work is an excellent method to gain experience with Python’s object-oriented programming language. Start implementing some OOP concepts into the code of a project that interests you.
Keep in mind that mastering OOP in Python requires time and effort. If you don’t understand everything at first, don’t give up. You will always get better if you keep pushing yourself and improving your skills.
Conclusion
Lastly, Python is a flexible programming language that supports object-oriented programming (OOP), a basic idea in software development. This article covered the basics of how Python works with the core OOP ideas of encapsulation, inheritance, and polymorphism.
When programmers learn OOP in Python, they can make code that is more stable, reusable, easy to keep up to date, and easy to add features to.
This article helped you clear your doubts about object-oriented programming in Python.
I would also encourage you to go through the exercises on object-oriented programming in Python to understand the subject better.