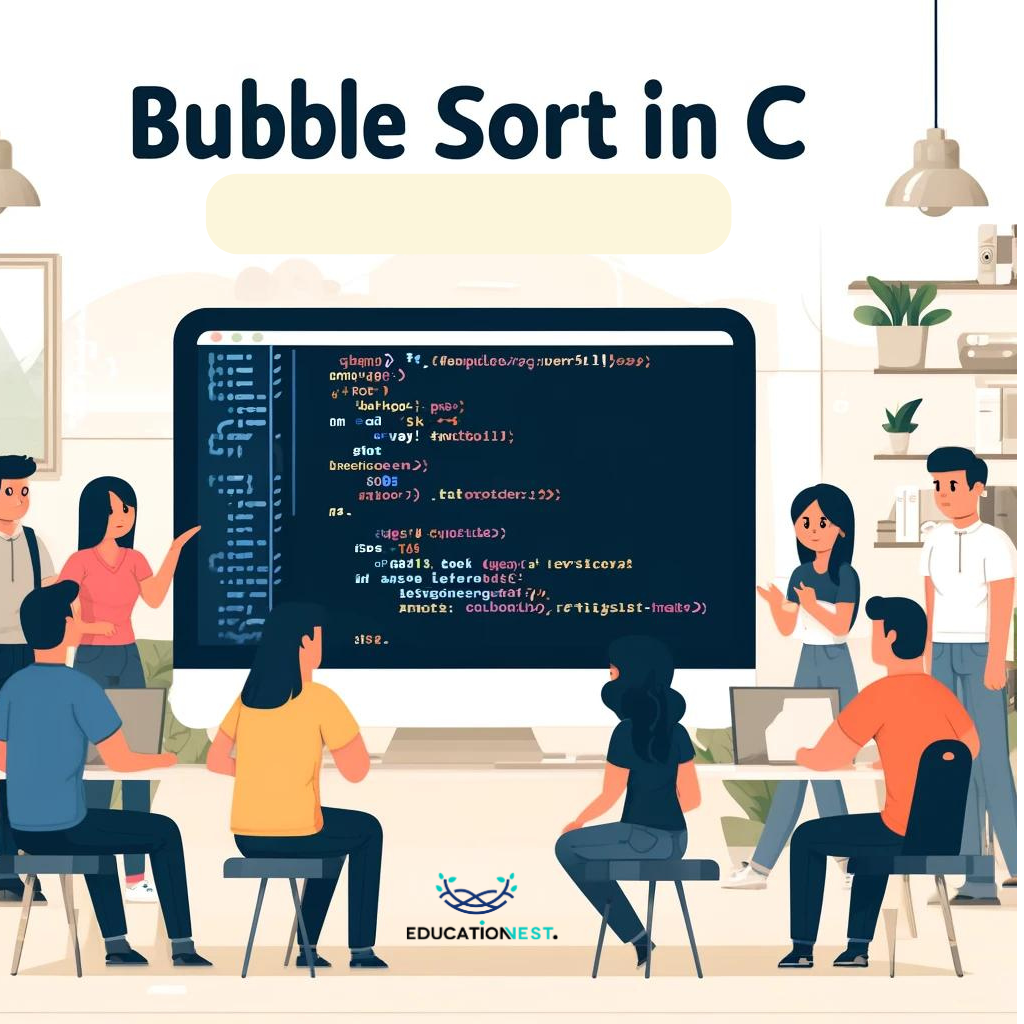
Have you ever wondered how sorting really works in programming? One of the simplest methods to understand is the bubble sort algorithm, particularly the bubble sort in C. This blog will take you through the bubble sort example step by step, so you can grasp how to implement it. We’ll explore the bubble sort algorithm, highlighting every detail in an easy-to-understand manner. You’ll learn not only the basic concept but also how to write an algorithm for bubble sort in C. By the end of this guide, the bubble sort example step by step will demystify what might seem complex at first glance and show you just how accessible coding can be.
Understanding Bubble Sort in C
Bubble sort is a straightforward sorting technique used in computer programming. It works by repeatedly stepping through a list, comparing adjacent elements and swapping them if they are in the wrong order. This process is repeated until the list is sorted. The name “bubble sort” comes from the way smaller elements “bubble” to the top of the list, while the larger ones sink to the bottom.
Let’s break it down further with a simple example. Imagine you have a row of numbers you want to arrange from smallest to largest. You start at one end and compare each pair of numbers, swapping them to put the smaller number first. You keep doing this, moving through the list until you reach the end. This completes one full pass. However, you might need to repeat this process multiple times because the largest number might not immediately end up at the end of the list on the first try.
The efficiency of bubble sort isn’t the best for large data sets, but its simplicity makes it a great educational tool for new programmers.
Also Read:
Understanding Constructor Overloading in Java: A Simple Guide
Writing the Bubble Sort Algorithm in C
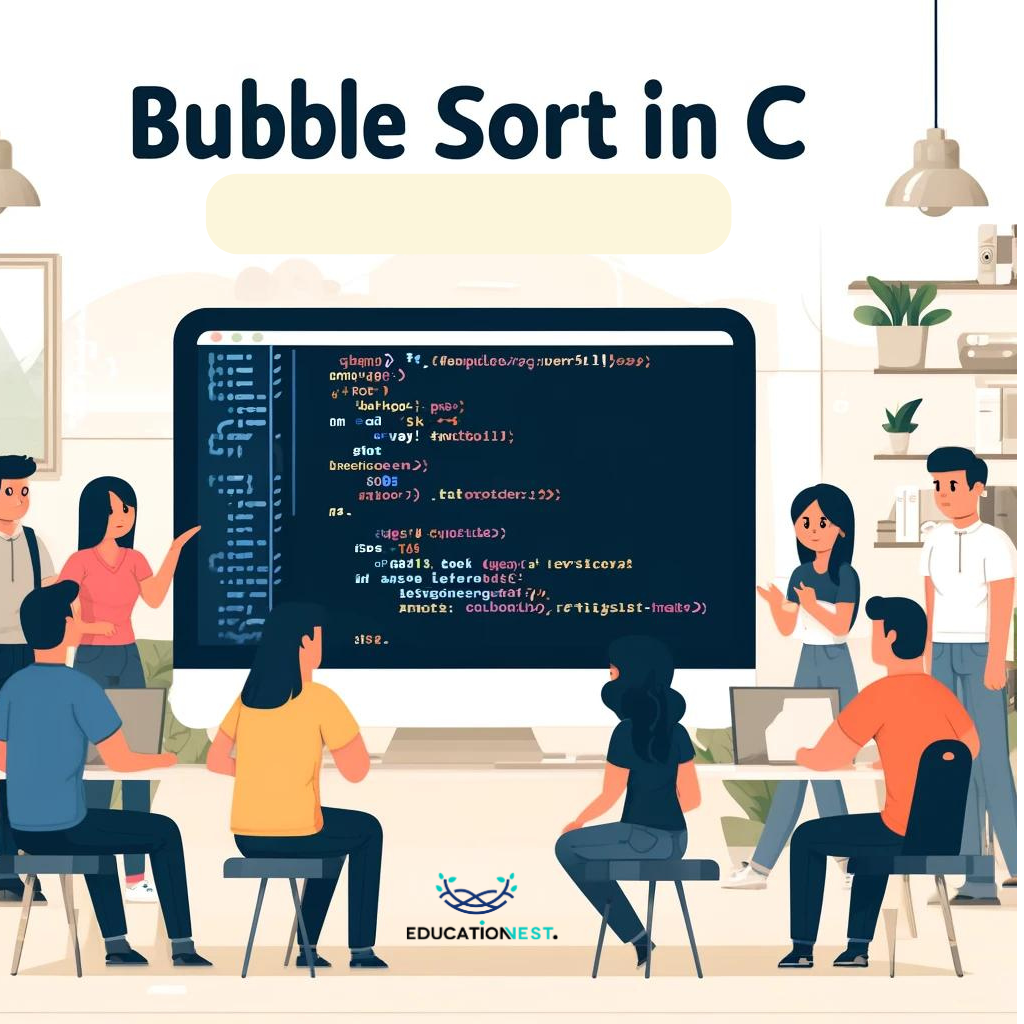
Now, let’s dive into how to code the bubble sort algorithm in C. We’ll go through it step by step to ensure it’s clear and easy to follow.
#include <stdio.h>
void bubbleSort(int array[], int size) {
for (int step = 0; step < size – 1; ++step) {
for (int i = 0; i < size – step – 1; ++i) {
if (array[i] > array[i + 1]) {
int temp = array[i];
array[i] = array[i + 1];
array[i + 1] = temp;
}
}
}
}
int main() {
int data[] = { -2, 45, 0, 11, -9 };
int size = sizeof(data) / sizeof(data[0]);
bubbleSort(data, size);
printf(“Sorted Array in Ascending Order:\n”);
for (int i = 0; i < size; i++) {
printf(“%d “, data[i]);
}
return 0;
}
In this code, bubbleSort is a function that takes an array and its size as parameters. The nested for-loops help perform the swaps necessary to sort the array. Notice how the inner loop’s range decreases with each pass through the outer loop. This is because the largest elements are progressively finding their place at the end of the array, and there’s no need to recheck them.
Conclusion
Bubble sort, while not the most efficient algorithm for large data sets, offers a perfect starting point for beginners in programming. It’s a tangible way to see how simple logic can be applied to solve problems systematically. With the bubble sort example step by step and the code provided, you should feel more confident in understanding and writing basic sorting algorithms in C. Whether you’re just starting out or brushing up on fundamentals, mastering bubble sort is a step forward in your programming journey.