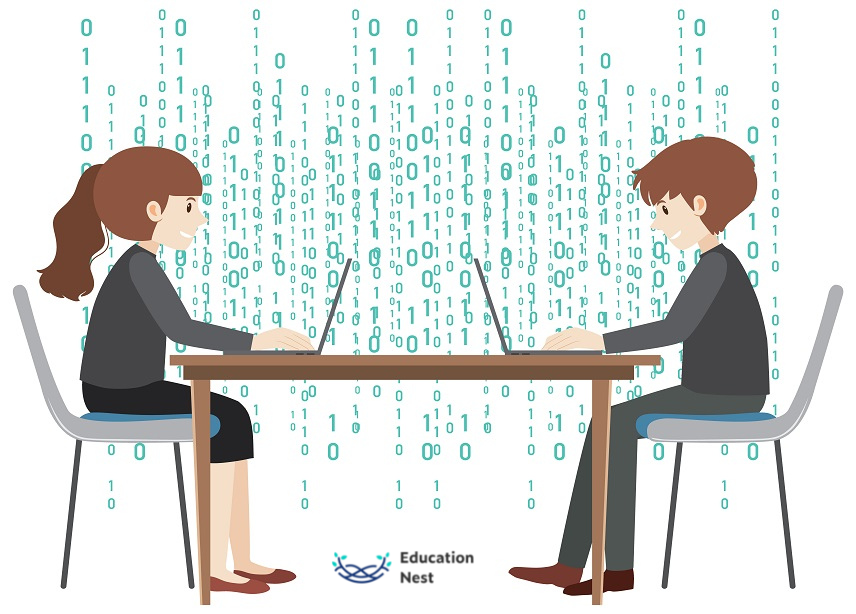
In this blog post, we’ve compiled a list of 36 commonly asked Python interview questions to help you prepare for your next job interview. From basic programming concepts to more advanced topics, our list covers a wide range of Python knowledge. Each question also comes with a detailed answer to help you understand the underlying concepts and improve your Python skills. Whether you’re a beginner or an experienced developer, this guide is a valuable resource to help you succeed in your Python interviews.
Python was made by Guido van Rossum and put out for the first time on February 20, 1991. It is one of the most popular programming languages. After all, it can use dynamic semantics because it is an interpreted language. The language is open source and free, and its syntax is easy to understand. This is a step that will help developers learn Python. Python is a popular programming language that can be used for many tasks. It can also be used for object-oriented programming.
Python is becoming increasingly popular because it is easy to use and can do more with fewer lines of code. Python is used in many fields because it can handle complex calculations and has strong libraries. These include Machine Learning, Artificial Intelligence, Web Development, and Web Scraping. This has led to a need for Python developers never like before in places like India and all over the world. Companies that want to hire these engineers are ready to offer them great bonuses and perks.
If you read this post and study the most frequently asked questions and answers, you’ll be able to ace in your Python Interviews
We split them up into the following groups:
- Python Interview Questions for Freshers
- Python Interview Questions for Experienced
- Python interview Questions for Data Analyst
- Python Interview Questions for Coding
How Freshers should prepare for Interview in Python
1. What is Python? What are the good things about Python?
Python is a high-level, general-purpose language that is read out loud. A language like this can make almost any program with the right frameworks and libraries. Also, Python’s support for objects, modules, threads, handling exceptions, and intelligent memory management makes it easier to model real-world problems and make apps to solve them.
Using Python has a lot of good points:
Python is a general-purpose programming language with an easy-to-understand syntax that emphasizes readability and, as a result, lowers the cost of maintaining programs. The language can also be scripted, is free and open-source, and can use third-party packages, which all add to its modularity and ability to be used in different ways.
2. What is dynamic typing?
Because it has high-level data structures, dynamic typing, and dynamic binding, developers worldwide use it for Rapid Application Development and deployment.
First, let’s talk about what a dynamically typed language is.
To understand a dynamically typed language, you must first learn how to order. In computer languages, “typing” is a short form for “type-checking.” When you add “1” and “2,” you’ll get a type error in a strongly-typed language like Python, which doesn’t support “type-coercion” (implicit conversion of data types). On the other hand, a weakly typed language like JavaScript will return “12” as the answer.
There are two different times when type checks can be done:
Before any code is run, the types of data are checked statically.
Different data types are checked in real-time during processing, which makes this method dynamic.
Python is an interpreted language, meaning each statement is run one at a time, and type-checking happens as the program runs. Python is called a Dynamically Typed Language because of this.
3. What is an interpreted language?
A language that is interpreted runs its statements line by line. Interpreted languages include Python, JavaScript, R, PHP, and Ruby. Programs written in an interpreted language don’t need to be compiled first. Instead, they run right from the source code.
4. What Is PEP 8 and Why Is It Important?
This is referred to as a Python Enhancement Proposal. A PEP, or Python Enhancement Proposal, is a recognized design specification for the Python community. PEPs can outline new Python features or underlying operations. PEP 8 is important since it outlines the approved Python programming practices. You must strictly follow these style guidelines to contribute significantly to the Python open-source community.
5. What is the scope of Python?
Each Python object functions within a specific scope. An object in Python has a limited range of applications. Every piece of code in a program is given a label in the namespace. These namespaces have a defined scope, though, in which their objects can be used without a prefix. Several examples of Python scope that were created when the code was being run are shown below:
The objects produced by the current function are regarded as ” local. “
Suppose the objects created at the start of a program’s execution are still accessible after the program has ended. In that case, they are said to have a global scope.
The objects that the current module can access are declared at the module level.
The entire collection of usable programmatic names is called the “outermost scope.” This scope is searched once more after all other possibilities have been explored to find the required word.
Using keywords like global, an object’s local and international scopes can be synchronized.
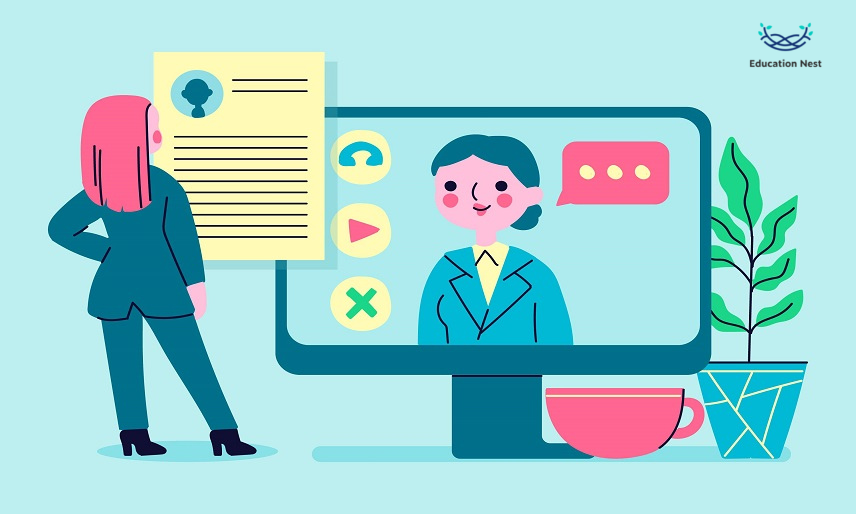
5. How about we talk about lists and tuples? What precisely separates the two, then?
Lists and tuples are two sequence data structures used in Python to hold groups of items. Both sequences can grasp objects with various types of data. A list is indicated by square brackets [“Sara,” 6, 0.19], whereas a tuple is indicated by parentheses (“ansh,” 5, 0.97).
Tuples and lists differ primarily because tuples are immutable, whereas lists are not. This indicates that tuples are immutable and cannot be modified in any way. In contrast, lists can be dynamically updated by adding or splitting them up. Running the following code in Python IDLE is one approach to confirm the distinction. Even though Python doesn’t require explicit data type definitions when declaring variables, type errors may still occur if one is unaware of data types and how they interact.
7. What are the typical Python built-in data types?
Python comes with several built-in data types. Although Python does not require explicit data type definitions during variable declarations, type mistakes are possible if data type knowledge and interoperability are not considered. Python offers the type() and instance () methods to determine the type of these variables. The following categories can be used to classify these data types:
You Must Like: Hadoop Features and Advantages You Must Know
None Type: The Python language‘s “none” keyword designates null values. These NoneType objects can be used to conduct boolean equality operations.
NoneType is a class that represents Python’s NULL values.
Integers, floating-point numbers, and complex numbers are numeric types. Additionally, a subtype of integers is booleans.
Class Name Description Integer literals, such as hexadecimal, octal, and binary values, are stored as integers in the int class.
Stores literals with exponent signs and decimal values as floating-point numbers.
Complex stores complex numbers in the form (A + Bj) and has the following properties: real, image, and bool (True or False).
Notably, the standard library also contains decimals to store floating-point integers with user-defined precision and fractions to store rational values.
According to Python Docs, lists, tuples, and range objects are the three fundamental sequence types. The in and not in operators are defined for traversing the elements of sequence types. The priority of these operators is the same as that of the comparison operations.
Hashable values can be mapped to random objects in Python using mapping types. There is currently only one common mapping type, the dictionary, and mapping objects are mutable.
The two built-in set types in Python right now are set and frozenset. The set type is mutable and is compatible with add() and delete() methods (). The frozen set type is immutable and cannot be changed after it has been created.
Because the set is mutable, it cannot serve as the dictionary’s key. On the other hand, a frozen set can be used as a dictionary key or as a component of another set because it is immutable and thus hashable.
Modules: The Python interpreter also supports an additional built-in type called modules. Mymod.Myobj, where mymod is a module and myobj refers to a name defined in m’s symbol table, is the only special operation it supports. Although direct assignment to this module is not possible nor advised, the module’s symbol table is stored in a unique module attribute called __dict__.
Called-For Types:
The kinds to which a function call can be applied are calledable types. They can be built-in functions, methods, classes, instance methods, generator functions, and user-defined functions.
8. What in Python is pass?
In Python, a null operation is represented by the term pass. It is typically used to fill in blank blocks of code that may run during runtime but still need to be written. The following code may encounter specific issues if the pass statement is absent.
9. What are modules?
In general, modules are just Python files with a.py extension that include a defined and implemented set of functions, classes, or variables. The import statement can be used to import and initialize them once. Use from foo import bar to import the required courses or functions if just partial functionality is needed.
The module namespace can be hierarchically organized using packages and dot notation.
10. What do the Python terms global, protected, and private mean?
Public variables with a worldwide reach are referred to as global variables. The global keyword is used to reference a variable in the global scope inside a function.
Protected characteristics include an underscore before their identifier, for example, _sara. Although a good developer should refrain from doing so, they can be accessed and updated from outside the specified class.
Personal attributes have a double underscore before their name, for example, __ansh. They are not immediately accessible or adjustable from the outside, and any effort to do so will cause an attribute error.
11. In Python, what purpose does self-serve?
The self represents the class instance. This keyword can access the class’s attributes and methods in Python. It connects the given arguments and the attributes. Self is a word used frequently and frequently mistaken for a keyword. But in Python, self is not a keyword, unlike in C++.
12.What does __init__ mean?
When a new object or instance is formed, the function Object() { [native code] } method __init__ is immediately invoked to allocate memory. Every class has a related __init__ method. It aids in separating local variables from a class’s methods and properties.
13. What do the Python terms break, continue, and pass mean?
Break
The break statement abruptly ends the loop, passing control to the statement that follows the loop’s main body.
Continue
The continue statement ends the current iteration of the statement, skips the remainder of the current iteration’s code, and transfers control to the loop’s subsequent iteration.
Pass
As previously mentioned, the pass keyword in Python is typically used to fill up empty blocks. It is comparable to an empty statement in languages like Java, C++, Javascript, etc., denoted by a semicolon.
pat = [1, 3, 2, 1, 2, 3, 1, 0, 1, 3]
in part for p:
pass
If (p == 0), then current = p; if (p% 2 == 0), then continue printing (p) # output => 0 # output => 1 3 1 3 1 print(current).
14. What do Python unit tests entail?
Python’s unit test framework is used for unit testing.
Separate testing of each software component is known as unit testing. Describe the importance of unit testing in your own words. Consider a situation where you are developing software that utilizes the following three components: A, B, and C. Now imagine that, at some point, your software malfunctions. How will you identify the component that caused the software to malfunction? Perhaps part A failed, causing component B to fail, and this ultimately caused the software to fail. These combinations are numerous.
Because of this, it’s essential to thoroughly test each component to identify any that may be particularly at fault for the software’s failure.
15. In Python, what is docstring?
A multiline string known as a documentation string or docstring is used to describe a particular code segment.
What the function or method does should be explained in the docstring.
16. What in Python is slicing?
Slicing, as the name implies, is the taking of pieces.
Slice syntax is [start: stop: step].
The starting index from which to cut a list or tuple is called the start.
The stopping index, or where to stop, is sop.
The amount of steps needed to jump is one.
Start defaults to 0, stop to a number of items, and step to 1.
Strings, arrays, lists, and tuples can all be sliced.
17. What makes Python lists different from arrays?
In Python, an array can only have items with the same data type. This is called homogeneous data type. It takes up much less memory than lists and is just a thin layer on top of C language arrays.
In Python, lists can have different types of data, meaning they can have various items. The bad thing about it is that it takes up a lot of memory.
Python interview questions for experienced
18. How does Python handle memory?
The Python Memory Manager is in charge of memory management in Python. Python is given memory in the form of a private heap area by the manager. This private heap stores all Python objects and is inaccessible to programmers. However, Python provides some essential API functions for working with the private heap space.
Python also has a garbage collector built in to reuse any unused memory for the private heap area.
19. What exactly are Python namespaces? Why are they employed?
In Python, a namespace ensures that object names in a program are unique and can be used without conflict. Python implements namespaces as dictionaries, with a ‘name as key’ mapping to a ‘object as value.’ This enables multiple namespaces to share the same name and map it to a different object. Here are some examples of namespaces:
Local namespace refers to names found within a function. The namespace is created temporarily for a function call and cleared when the function returns.
Global Namespace contains names from various imported packages/modules used in the current project. This namespace is created when the package is imported into the script and persists until the script is executed.
The built-in namespace contains built-in core Python functions as well as built-in names for various types of exceptions.
The lifecycle of a namespace is determined by the scope of objects to which it is mapped. When the scope of an object expires, so does the lifecycle of that namespace. As a result, accessing inner namespace objects from an outer namespace is impossible.
20. What exactly does Python’s Scope Resolution imply?
Objects with the same name but different functions may sometimes exist in the same scope. Python engages scope resolution automatically in certain situations. Here are a few examples of similar behavior:
Many functions, such as log10(), acos(), and exp(), can be found in both the math and cmath Python modules (). To resolve this issue, you must prefix them with their corresponding module, such as math.exp() and cmath.exp ().
Consider the following code, which initializes a temporary object to 10 globally and 20 when a function is called. The function call, however, did not change the value of temp globally. In this case, we can see that Python clearly distinguishes between global and local variables by treating their namespaces as separate entities.
21. What are Python decorators?
Decorators in Python are functions that extend the capabilities of a pre-existing function without changing its structure. The @decorator name denotes bottom-up decorators in Python. The beauty of decorators lies in their ability to accept method arguments and change them before passing them to the function itself, in addition to providing functionality to the method’s output. In this case, the “wrapper” function, which is an inner nested function, is crucial. It is intended to maintain encapsulation and, thus, privacy from the global scope.
22. What are dicta and list comprehensions?
Python comprehensions, like decorators, are syntactic sugar constructions that aid in creating modified and filtered versions of lists, dictionaries, or sets from a given list, glossary, or set. Using comprehensions saves time and sometimes results in more verbose code (containing more lines of code)
23. What is lambda in Python? Why is it in use?
The lambda function in Python is anonymous with any number of parameters but only one expression. It is typically used when an unknown temporary position is required.
24 .Explain how to delete a file in Python?
Use command os. remove(file_name)
25 .Explain split() and join() functions in Python?
- You can use the split() function to split a string based on a delimiter to a list of strings.
- You can use the join() function to join a list of strings based on a delimiter to give a single string.
Python Interview Questions for Data Sciences
Python interview questions are widely used in technical data science interviews. You’ll be asked about fundamental Python coding principles in a typical interview. As a starting point for your preparation, use these recently revised Python data science interview questions on statistics, probability, string parsing, NumPy/matrices, and Pandas.
The following topics are typically covered in Python data science interview questions:
questions concerning string manipulation in Python, questions about probability and statistics
Pandas in Python problems
Matrix questions and NumPy Python questions concerning Python machine learning
Why are Python questions asked in data science interviews?
Python has dominated data science recently, dethroning previous giants such as R, Julia, Spark, and Scala. This is partly due to its extensive collection of data science libraries (modules), supported by a thriving and developing data science community.
The versatility of Python libraries to be utilized throughout the entire data science stack is one of the key reasons it is the language of choice today. Each data science language specializes in a unique area of expertise; for example, R excels at academic data analysis and modeling, whereas Spark and Scala excel at big data ETLs and production. On the other hand, Python has created an ecosystem of libraries that operate nicely together. Finally, when you don’t have to switch languages, programming and full-stack data science become much more accessible. This includes using a single language to conduct exploratory data analysis, develop the model, deploy it, and generate graphs and visualizations.
Who Is Asked Python Questions?
Data scientists, machine learning engineers, and data analysts are given Python interview questions. The work, on the other hand, decides how complicated the question is.
Here are some examples of how Python inquiries from data scientists and data analysts differ:
Python queries for data analysts are typically more straightforward and scripting-oriented. The majority of the questions will be straightforward Pandas and Python tasks.
Engineers and scientists that work with data More than two-thirds of data scientists use Python daily. The questions include fundamental concepts such as conditions, branching, loops, functions, and object-oriented programming. Along with sophisticated concepts like regression, K-means clustering, and classification, data engineer job questions usually cover standard libraries like Pandas, SciPy, and NumPy.
26. Which Python data types are used?
Python makes use of several built-in data types, including:
Number (integer, float, or complex) (int, float, and complex)
str (string) (str)
The tuple (tuple) (tuple)
The range (range) (range)
a list (a list) (list)
Set up (set up) (set)
Thesaurus (dict) (dict)
In Python, each value has a data type used to classify or categorize the data.
27. What is the operation of Python data analysis libraries? What are some of the most popular libraries?
One of the critical reasons Python is such a popular data science programming language is the enormous number of data analysis packages available. These libraries include data organization and processing tools, strategies, and functions. Python libraries are public for many data science activities, including text and image data processing, mining, and visualization. The following are the most popular Python data analysis libraries:
Pandas
NumPy\sSciPy\sTensorFlow
SciKit\sSeaborn\sMatplotlib\s3. Python uses a negative index in what way?
Python evaluates and indexes lists and arrays using negative indexes, beginning at the end of a string and moving backward to the first value. For example, n-1 shows the final item in a list, whereas n-2 shows the next-to-last. Here’s an example of a Python negative index:
28. Matplotlib or Seaborn as your favorite plotting library?
Seaborn and Matplotlib are two of the most popular Python visualization libraries. It is worth noting that Seaborn is built on Matplotlib. Seaborn, on the other hand, frequently provides more customization due to its integrated tools. As a result, Seaborn can accelerate the process, and Matplotlib can be utilized for the final touches.
Note: This question is about preferences. The library you choose may change depending on the work or your level of tool knowledge. In other words, there are no good or wrong answers; the interviewer is more interested in knowing how skilled you are at using Python to create visualizations.
29. Is Python an object-oriented programming language?
Yes and no. Python incorporates aspects of aspect-oriented and object-oriented programming (OOP). One reason Python cannot be considered a proper OOP language is that it needs to provide strong encapsulation, the only essential component of an OOP that it does not support.
30. What distinguishes a series from a data frame in Pandas?
A data frame can have one or more series, but each can only have one list with an index. These are the words:
The one-dimensional array series supports any datatype (including integers, strings, floats, etc.). The axis labels provide the index of a series.
A data frame is a two-dimensional data structure with columns that can hold many data types. It is similar to a series object dictionary or a SQL table.
31. How would you look for duplicate values for a variable in a dataset in Python?
You can look for duplicates with Pandas’ duplicated() method. This will result in a boolean series that is TRUE only for unique components.
Python questions for coding
32. Write the output for the below Hello, Python commands.
Python
1
print(‘Hello, Python!’)
2
print(“Hello, Python!”)
3
print(”’Hello, Python!”’)
4
print(‘”Hello, Python”‘)
5
print(“‘Hello, Python'”)
Output
Hello, Python!
Hello, Python!
Hello, Python!
“Hello, Python!”
‘Hello, Python!
Explanation: In Python programming, a string can be enclosed inside single quotes, double quotes, or triple quotes.
33.What is the output of the Python add two numbers program?
Python Add Two Numbers Program
#Python Add Two Numbers Program
number1 = 5
number2 = 4
# Add two numbers
sum = number1 + number2
# Display the sum of the two numbers
print(‘The sum of the two numbers is:’ sum)
Output of the program:
File “”, line 7
print(‘The sum of the two numbers is:’ sum)
^
SyntaxError: invalid syntax
The above Python add two numbers program throws SyntaxError because, the comma(,) is missing in print statement. The below print statement will give you the sum of the two numbers.
print(‘The sum of the two numbers is:’, sum)
34. What is the output of the below sum of the two numbers Python program?
Python
1
num1 = input(‘Enter first number: ‘)
2
num2 = input(‘Enter second number: ‘)
3
sum = num1 + num2
4
print(‘The sum of the numbers is’, sum0
The output of the sum of the two numbers program
Enter first number: 15
Enter second number: 10
The sum of the numbers is 1510
Python input() function always converts the user input into a string. Whether you enter an int or float value it will consider it as a string. We need to convert it into number using int() or float() functions. See the below example.
Read the input numbers from users
num1 = input(‘Enter the first number: ‘)
Converting and adding two numbers using int() & float() functions
sum = int(num1) + int(num2)
sum2 = float(num1) + float(num2)
Displaying the sum of two numbers
print(‘The sum of {0} and {1} is {2}’.format(num1, num2, sum))
print(‘The sum of {0} and {1} is {2}’.format(num1, num2, sum2))
The output of the sum of the two numbers program
Enter the first number: 15
Enter the second number: 10
The sum of 15 and 10 is 25
The sum of 15 and 10 is 25.0
35. Write a Python program to illustrate arithmetic operations (+,-,*,/)?
Here is the Python arithmetic operators program:
Python
1
# Read the input numbers from users
2
num1 = input(‘Enter the first number: ‘)
3
num2 = input(‘Enter the second number: ‘)
4
5
# Converting and adding two numbers using int() & float() functions
6
sum = int(num1) + int(num2)
7
8
# Subtracting the two numbers
9
sub = int(num1) – int(num2)
10
11
# Multiplying two numbers
12
mul = float(num1) * float(num2)
13
14
#Dividing two numbers
15
div = float(num1) / float(num2)
16
17
18
# Displaying the results of arithmetic operations
19
print(‘The sum of {0} and {1} is {2}’.format(num1, num2, sum))
20
print(‘The subtration of {0} and {1} is {2}’.format(num1, num2, sub))
21
print(‘The multiplication of {0} and {1} is {2}’.format(num1, num2, mul))
22
print(‘The division of {0} and {1} is {2}’.format(num1, num2, div))
23
The output of the Python arithmetic operators program
Enter the first number: 25
Enter the second number: 10
The sum of 25 and 10 is 35
The subtraction of 25 and 10 is 15
The multiplication of 25 and 10 is 250.0
The division of 25 and 10 is 2.5
36.Python Program to Check if a Number is Odd or Even.
Python
1
#Python program to check if a number is odd or even
2
3
#To get the input from user
4
num1 = input(“Enter a number: “)
5
6
#Checking whether the entered number is odd or even
7
if (int(num1) % 2) == 0:
8
print(“{0} is Even number”.format(num1))
9
else:
10
print(“{0} is Odd number”.format(num1))