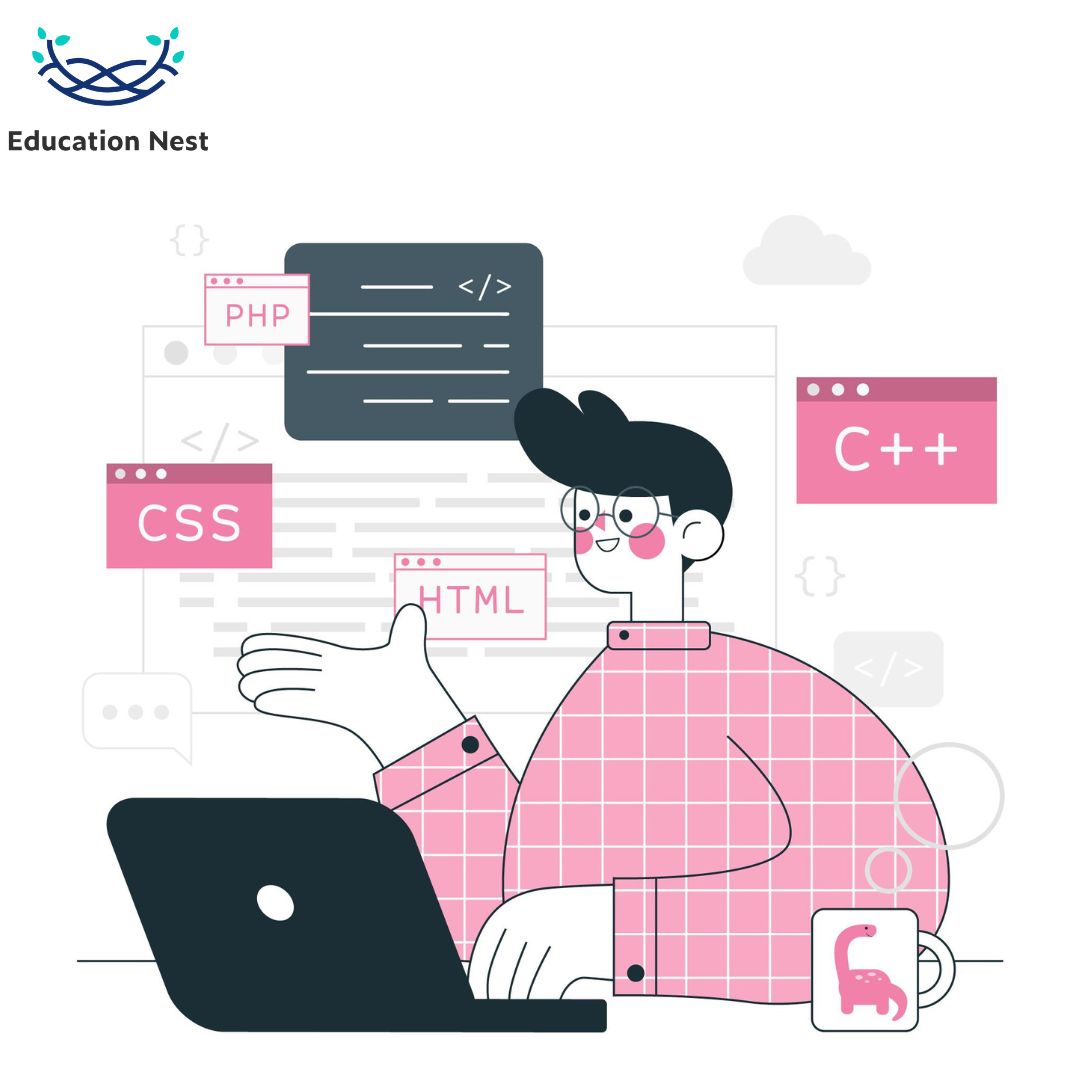
In the Python programming language, there are many kinds of data, such as lists, sets, dictionaries, etc. The “collections” package in Python is a group of data structures that can be used together. Another common way to group data in Python is with tuples. In this post, we’ll learn about the tuples in Python, the tuple in Python example, the difference between a list and a tuple in Python, and how to create a tuple in Python.
What Do You Mean By Tuples in Python?
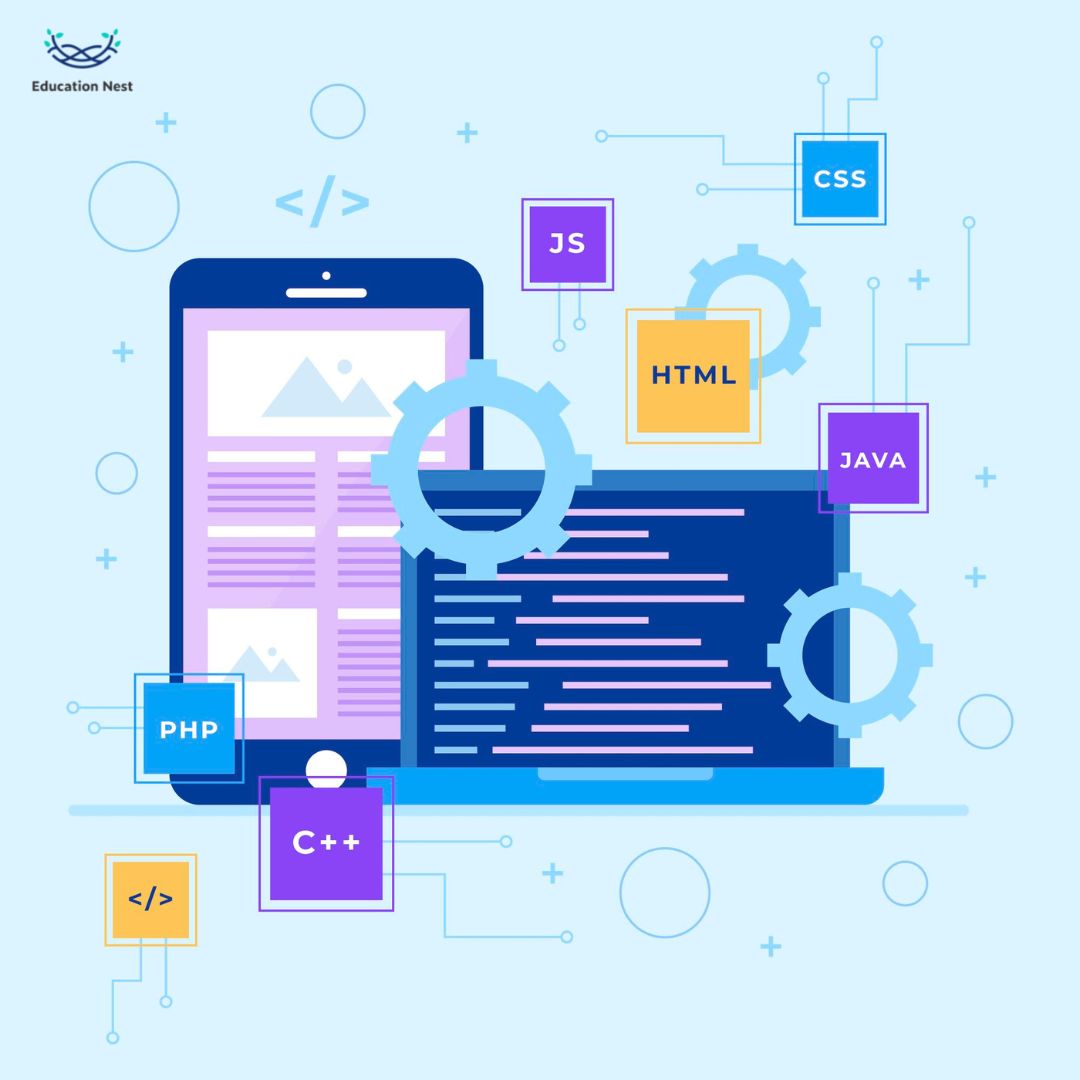
A tuple is a type of data in Python that can’t be changed. It’s almost the same as a list in regards to indexing and having duplicate members. It is a type of data called a collection containing comma-separated Python objects.
Tuples in Python Example:
making a tuple
a = (‘python’, ‘educationnest’)
#another approach
b = ‘python’ , ‘educationnest’
print(a)
print(b)
Output: (‘python’, ‘educationist’)
(‘python’ , ‘educationnest’)
How to Create Tuples in Python
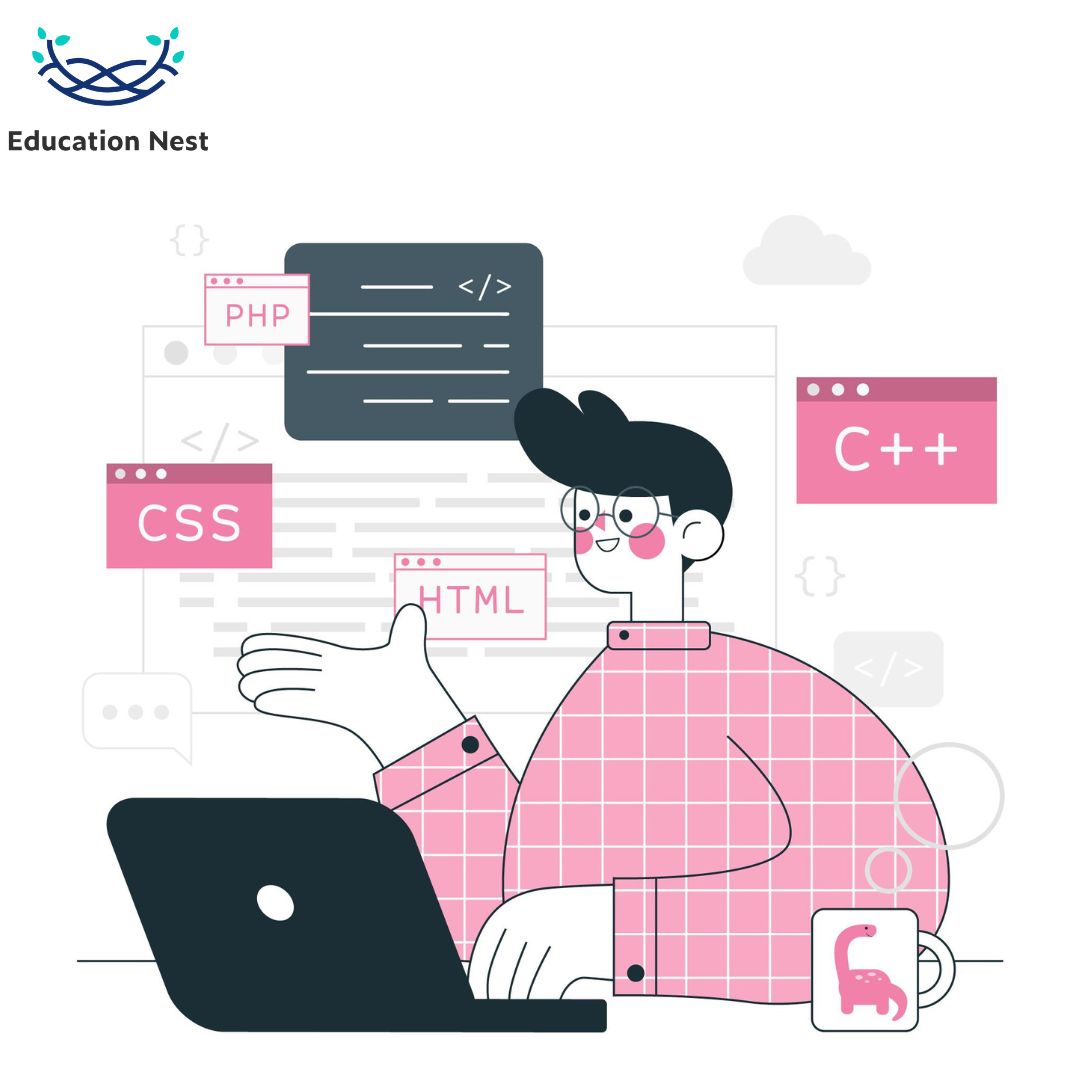
Putting all the items (elements) inside parentheses and separating them with commas makes a tuple. Even though you don’t have to use the parentheses, it’s a good idea to do so.
Any items of different kinds can be in a tuple (integer, float, list, string, etc.).
# Different types of tuples
# Empty tuple
my_tuple = ()
print(my_tuple)
# Tuple having integers
my_tuple = (1, 2, 3)
print(my_tuple)
# tuple with mixed data types
my_tuple = (1, “Hello”, 3.4)
print(my_tuple)
# nested tuple
my_tuple = (“mouse”, [8, 4, 6], (1, 2, 3))
print(my_tuple)
Output
()
(1, 2, 3)
(1, ‘Hello’, 3.4)
(‘mouse’, [8, 4, 6], (1, 2, 3))
In the example above, we made different kinds of tuples and put different data types into them.
We can also make tuples without using parentheses, as we’ve already said:
my_tuple = 1, 2, 3
my_tuple = 1, “Hello,” 3.4
Also Read: Simple Prime Numbers in Python: From User Input to Range and Functions
How to Create A Tuple in Python With One Element
It’s not easy to make a tuple with only one item in Python. It’s not enough to have just one thing in parentheses.
We’ll need a comma at the end to show that it’s a tuple,
var1 = (“Hello”) # string
var2 = (“Hello”,) # tuple
We can determine what class a variable or value belongs to using the type() function.
var1 = (“hello”)
print(type(var1)) # <class ‘str’>
# Creating a tuple having one element
var2 = (“hello”,)
print(type(var2)) # <class ‘tuple’>
# Parentheses are optional
var3 = “hello”,
print(type(var3)) # <class ‘tuple’>
What Is the Difference Between a List and Tuples in Python?
Python data structures fall into two categories: lists and tuples. The list changes over time, while the tuple remains unchanged. Tuples are static in nature and cannot be changed, so they are faster than lists, which are dynamic. Square brackets are used to indicate lists, while parentheses are used for tuples.
Comparison of Python’s List and Tuple data structures and their key differences
Sr. No. | Key | List | Tuple |
1 | Type | List is mutable. | Tuple is immutable. |
2 | Iteration | List iteration is slower and more time-consuming. | The tuple iteration is faster. |
3 | Appropriate for | Lists are useful for insertion and deletion operations. | Tuple is useful for read-only operations like accessing elements. |
4 | Memory Consumption | list consumes more memory. | Tuples consume less memory. |
5 | Methods | list provides many in-built methods. | Tuples have fewer in-built methods. |
6 | Error prone | List operations are more error-prone. | Tuples operations are safe. |
Tuple Methods in Python
There are several built-in ways to change tuples in Python. Here are some of the Python tuple methods that are used most often:
- Tuple length
The Python tuple method len() returns the number of elements in the tuple.
EX: tuple1, tuple2 = (“Java”, “Python”, “C”), (“Windows”, “linux”)
print(len(tuple1))
Output:
Tuple1, tuple2 = (“ Java” , “Python, “C”), (“Windows”), 3 “linux”)
Print (len(tuple1))
- Tuple count
The count() method tells how often a given value appears in the tuple.
Syntax
tuple.count(value)
Example:
tuple = (1, 3, 7, 8, 7, 5, 4, 5, 6, 8, 5)
Count = tuple.count(5)
print(count)
- Tuple Max
Python’s max() method finds the most oversized item in an iterable and returns it. For tuples, it gives back the most significant element based on the elements’ natural order.
Example 1 :
numbers = (1, 2, 3, 4, 5)
max = max(numbers)
print(max)
If the tuple has different data types, like integers and strings, the max() method will throw a TypeError. To handle this, you can pass the tuple to the sorted() function, which returns a sorted list, and then use the max() method on the sorted list.
Example 2:
Here’s an example of using the max() method on a tuple of integers and strings:
mixed (1, “hello,” 3; “world”).
sorted_mixed = sorted(mixed, key=lambda x: str(x))
print(sorted_mixed)
max = max(sorted_mixed, key=lambda x: str(x))
print(max)
- Tuple Min
Python’s min() method finds the smallest item in an iterable and returns it.
Here’s how to use the min() method with a list of integers:
Example 1:
numbers = (5, 3, 1, 4, 2)
min = min(numbers)
print(min)
- Tuple sorted()
The sorted() function in Python returns a sorted list of elements from a given iterable. This function can also be used on tuples to sort the elements in a natural order, from lowest to highest. The comparison operators >, =, >=, ==, and!= tell you the natural order.
Here’s an example of how to use the sorted() function on a tuple of integers:
numbers = (5, 3, 1, 4, 2)
sorted_num = sorted(numbers)
print(sorted_num)
- Tuple Index
In Python, you can get to the elements of a tuple by putting the index of the element you want to get to between square brackets. The first element of a tuple has an index of 0, the second element has an index of 1, and so on.
Example 1:
Since indexing starts at 0, in this example, the first element, application performance monitoring, has an index of 0, the second element, log monitoring, has an index of 1, and so on.
You can use the following code for accessing the tuple elements:
monitoring_tools = (
“Application performance monitoring”,
“Logs monitoring”,
“Real user monitoring”,
“Server monitoring”,
“Infrastructure monitoring”,
)
print(monitoring_tools[1])
print(monitoring_tools[2])
Conclusion
Python’s tuple data structure is a sequence of ordered, immutable lists. Using parentheses and commas to separate each component makes it easier to see. Tuples have several applications, including data grouping, function return, and more.
Tuples’ immutability is one of the main reasons why they are so popular. It is impossible to change a tuple’s components after it has been generated. This makes tuples handy when you need to ensure the values aren’t modified, whether by accident or on purpose. Tuples also support nesting, which allows one tuple to contain another as an element. This enables the use of tuples as a representation for sophisticated data structures.
In general, tuples are a robust data structure in Python that can be combined with other data structures like lists and dictionaries to solve various issues.
For this purpose, instead of using arrays, you can use tuples to unpack values into variables.
Please visit educationnest.com for more interactive topics.