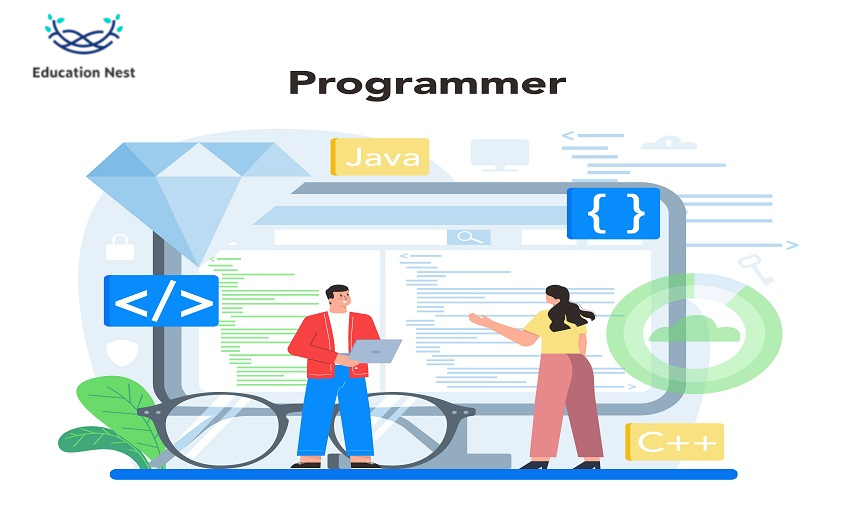
What does “object-oriented programming” mean?
Object-oriented programming, or OOP is a method of writing computer code that centers on data or objects rather than functions and logic. An object is a piece of data with its characteristics and behaviors.
OOP focuses on the objects that programmers want to work with rather than the logic required to do so.This development method works well for large, complex, and frequently updated or maintained programs. This includes mobile apps as well as software for designing and creating things. OOP can be used to develop simulation software for manufacturing systems also.
Because of how an object-oriented program is set up, the method is proper when projects are divided into groups for collaborative development. OOP also has the advantages of being fast, scalable, and capable of reusing code.
Data modelling is the process of assembling everything a programmer wants to work with and determining how those pieces fit together.
In terms of structure, how does object-oriented programming appear?
Object-oriented programming is composed of several components, or building blocks, such as those listed below:
- Classes, which are composed of user-defined data types, serve as the foundation for objects, attributes, and methods.
- Objects are specific instances of a class created from well-defined data. Objects can be either ideas or physical objects in the real world. The only thing that is set when a class is created for the first time is the description.
- Methods are internal class functions that describe how an object behaves. The first line of code in each method in a class declaration is a reference to an instance object. Instance methods are also used to refer to an object’s internal functions. Programmers can write code that can be used multiple times or keep functionality within a single object at a time.
- Attributes The class template creates attributes, which are similar to an object’s state. The information will be saved in the attributes field of the object. A class’s characteristics belong to the class.
What are the main concepts underlying OOP?
The following are the basic concepts of object-oriented programming:
- Encapsulation. According to this concept, only some information is displayed, and all important information is kept within an item. Inside a class, the implementation and state of each object are kept private. Other objects can’t get to this class, and they can’t change it. They can only invoke a limited set of public functions or methods. This feature to hide data improves application security and prevents accidental data damage.
- Abstraction. Objects hide any unnecessary implementation code and only display the internal parts required for other objects to function. More features can be added to the derived class. This concept may make it easier for developers to add or change things in the future.
- Inheritance. Classes can use code from other classes. By assigning relationships and subclasses, developers can reuse similar functionality while maintaining a clear hierarchy. Because it necessitates a more in-depth examination of the data, this aspect of OOP speeds up and improves accuracy.
- Polymorphism. Objects can take many different shapes and are intended to behave consistently in all of them. There will be less need for duplicate code because the program will determine which use or meaning is required for each execution of that object from a parent class. The parent class’s functions are then extended by creating a child class. Polymorphism allows various types of objects to pass through the same interface.
What are the advantages of OOP?
Some advantages of OOP include:
- Modularity. Encapsulation allows things to stand on their own, making it easier to collaborate on projects and figure out what’s wrong.
- Reusability. Because inheritance allows code to be reused, a team does not have to keep writing the same code.
- Productivity. Programmers can create new programmes more quickly by reusing previously written code and a variety of libraries.
It has the potential to grow and be easily updated. Programmers can create their own system features.
- Security. Encapsulation and abstraction allow complicated code to be hidden, software maintenance to be simplified, and internet protocols to be protected.
- Flexibility. Polymorphism allows a single function to change depending on its class. Various objects can also coexist on the same interface.
You Must Like: The Ultimate JavaScript Interview Questions Guide: Preparing for Success
The most widely used OOP programming languages
In this blog, we will discuss the six most popular object-oriented programming languages today: Java, C#, Ruby, Python, TypeScript, and PHP. Despite the fact that each of these languages has its own set of advantages and disadvantages, they are all based on objects.
There will be discussion of the six OOP languages.
- Java
Java is a language used to create Android apps because it was designed to work on any platform and be simple to learn.
Java is also popular in the business world due to its dependability and security features. Because it is simple to learn, this is an excellent first programming language. It can also be used for important projects that require dependability.
Java has been extremely popular since its initial release in 1995. It powers most websites that use content management systems such as WordPress or Drupal. It is also a component of the Mac OS X CPU and is used to program everything from video game consoles to iPhone apps.
2.C#
Microsoft created the C# programming language, which can be used in a variety of ways. This object-oriented language supports imperative, functional, and generic programming styles. C# was created in the early 2000s. Although it is similar to Java in many ways, it was created with the.NET framework, making it easier to build for Microsoft platforms such as ASP.
Because it works with the.NET Framework, C# has grown in popularity in the OOP community. It can also be used to create Android and Windows desktop apps.
C# is an excellent choice for beginners because it is simple to learn and has widespread support in the business world. However, because this OOP language is so widely used, it is easier to learn than other languages because there are so many resources available on the internet to assist you.
3.Python
This high-level, object-oriented programming language is simple to learn, allowing programmers to work faster and integrate systems more effectively. It is a dynamic, interpreted language that emphasizes readability and supports a variety of programming styles, including imperative, procedural, object-oriented, and functional.
Guido van Rossum created Python, a programming language that can be used in a variety of ways. Python is an excellent choice for beginners because it is simple to grasp and learn. It also has a vibrant community that creates a wealth of useful resources. It is a versatile programming language that can be used to create websites, perform scientific computations, write scripts, and perform other tasks.
4.Ruby
Ruby is a dynamic, open-source programming language that emphasizes ease of use and speed of execution. Ruby was created in the 1990s by Yukihiro Matsumoto. It has a lovely syntax that is simple to read and write. Because of its simple design and large standard library, Ruby is very reliable and easy to move around.
One of the best aspects of this OOP language is its community. There are numerous excellent resources available online, and the Ruby community is very active. Ruby is a powerful scripting language that is both safe and expressive. It is also simple to programme.
5.PHP
PHP is a popular programming language in general, and it is also one of the most commonly used object-oriented languages for creating dynamic web pages. Rasmus Lerdorf created PHP in 1995 with the intention of using it in conjunction with HTML and CSS to build websites.
PHP is an excellent choice for beginners because it is simple to learn, has a large community, and produces excellent resources.
6.TypeScript
TypeScript is one of the object-oriented programming languages. Because it has classes, interfaces, and optional static typing, it is a superset of JavaScript. JavaScript’s syntactical features can be useful when writing complex programmes that must function correctly. TypeScript files are also converted to standard JavaScript files that can be used with any browser or execution engine (like Node.js).
TypeScript also has type inference, which makes coding faster and less likely to contain errors. It also uses a different syntax for constructors and destroyers. This syntax makes it easier to recognise objects by name because the names of the objects are always written first in the literal object. Furthermore, because it is open source, it can be easily added to existing JavaScript projects or created from scratch.
Object oriented versus functional programming
Before knowing the difference between object oriented programming and functional program ming ,lets get a brief idea about what is functional programming .
Functional Programming
Mathematical functions are used in all functional programming relationships. Declarative programming is a type of programming. This approach focuses on “what to solve” rather than an imperative style. Statements are replaced by expressions. When variables are given values, a statement is executed, whereas an expression evaluates to a value. The following distinctions exist between these functions.
Functional programming is based on Lambda calculus:
Alonzo Church developed lambda calculus to investigate how functions are used in calculations. It is the most basic programming language. It lists items that can be counted. Lambda calculus can solve any problem. In terms of capability, it is comparable to a Turing machine. It defines and assesses functions. It is the foundation of the majority of functional programming languages used today.
Haskell, JavaScript, Python, Scala, Erlang, Lisp, ML, Clojure, OCaml, Common Lisp, Racket, and Racket ML are all examples of programming languages that allow functional programming.
Difference between OOP and Functional programming
Below is a list of the ways that functional programming is different from OOP:
- Functional programming is used to do a wide range of things when the data is fixed. Object-oriented programming is used to do a few tasks that have different but similar ways of working.
- Functional programming has a feature called programming without states. Object-oriented programming has a part called “stateful programming.”
- In functional programming, there is no such thing as a state. In object-oriented programming, the state is not just a metaphor.
- In functional programming, a function is the main thing that can be changed. In object-oriented programming, an object is the main thing that can be moved or changed.
- Functional programming doesn’t have side effects, so code running on more than one processor doesn’t change. The ways object-oriented programming is done may have effects that were not intended and may slow down a processor.
- In functional programming, what we are doing is the most important thing. In object-oriented programming, the main question is “How are we doing?”
- Functional programming is the best way to support abstraction over data and abstraction over behaviour. Most of the time, object-oriented programming helps with abstraction over data alone.
- Functional programming is a way to process a lot of data quickly and well for applications. Object-oriented programming is not a good fit for processing big amounts of data.
- In functional programming, you can’t use if-then statements. Conditional statements, like if-else and switch statements, can be used in object-oriented programming.
Conclusion-
The concepts of objects and classes are central to object-oriented programmings. This article discusses object-oriented programming in brief yet highlighting the important aspects of it.This page explains what OOP is and why you should use it. It also defines objects, classes, methods and attributes. It ends by Highlighting he differences between functional programming and OOP.