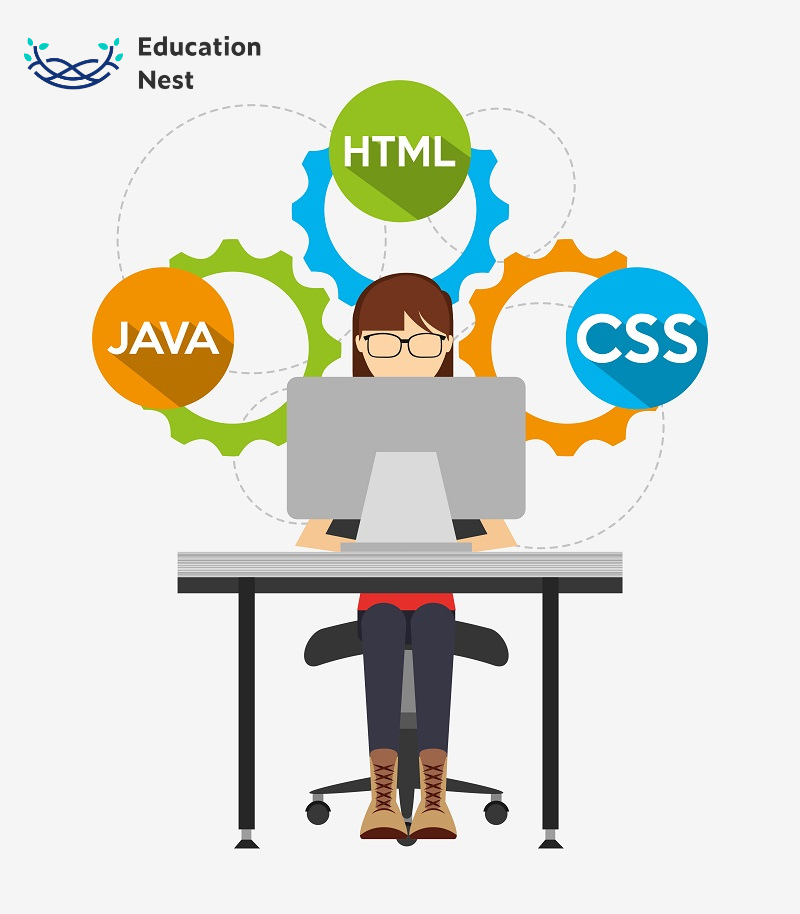
Java static keyword is frequently used to manage memory. The static keyword can be applied to variables, methods, blocks, and classes within classes. Static keywords are not a class instance. Instead, it is a class property.
This static could be:
- Variable (also known as a class variable)
- Method (also known as a class method)
- Block
- Nested class
- Java Static Variables
You declare a variable static to make it static.
The static variable can describe something that all objects share but is not unique to each object. For example, the employer’s name for employees, the college’s name for students, etc.
When the class is loaded, the static variable in the class area receives only one piece of memory.
The use of static variables has advantages.
Your program is now more memory efficient (i.e., saves memory).
Example of static variable
//Java Program to demonstrate the use of static variable
class Student{
int rollno;//instance variable
String name;
static String college =”ITS”;//static variable
//constructor
Student(int r, String n){
rollno = r;
name = n;
}
//method to display the values
void display (){System.out.println(rollno+” “+name+” “+college);}
}
//Test class to show the values of objects
public class TestStaticVariable1{
public static void main(String args[]){
Student s1 = new Student(111,”Karan”);
Student s2 = new Student(222,”Aryan”);
//we can change the college of all objects by the single line of code
//Student.college=”BBDIT”;
s1.display();
s2.display();
}
}
Output:
111 Karan ITS
111 Karan ITS
222 Aryan ITS
2. Static method in Java
Any method that begins with the keyword “static” is considered static.
- A static method is a class property, not a class object.
- A static method can be called without first creating an instance of the class.
- The values of static data members can be seen and changed by a static method.
Example of static method
//Java Program to demonstrate the use of a static method.
class Student{
int rollno;
String name;
static String college = “ITS”;
//static method to change the value of static variable
static void change(){
college = “BBDIT”;
}
//constructor to initialize the variable
Student(int r, String n){
rollno = r;
name = n;
}
//method to display values
void display(){System.out.println(rollno+” “+name+” “+college);}
}
//Test class to create and display the values of object
public class TestStaticMethod{
public static void main(String args[]){
Student.change();//calling change method
//creating objects
Student s1 = new Student(111,”Karan”);
Student s2 = new Student(222,”Aryan”);
Student s3 = new Student(333,”Sonoo”);
//calling display method
s1.display();
s2.display();
s3.display();
}
}
Output :
111 Karan BBDIT
222 Aryan BBDIT
333 Sonoo BBDIT
You Must Like: React vs. Angular: Which JavaScript Framework is Better?
3. Java static block
- Is used to initialize the static data member.
- It is executed before the main method at the time of classloading.
Example of static block
class A2{
static{System.out.println(“static block is invoked”);}
public static void main(String args[]){
System.out.println(“Hello main”);
}
}
Output : static block is invoked
Hello main
Interview Questions related to Java Static Keyword
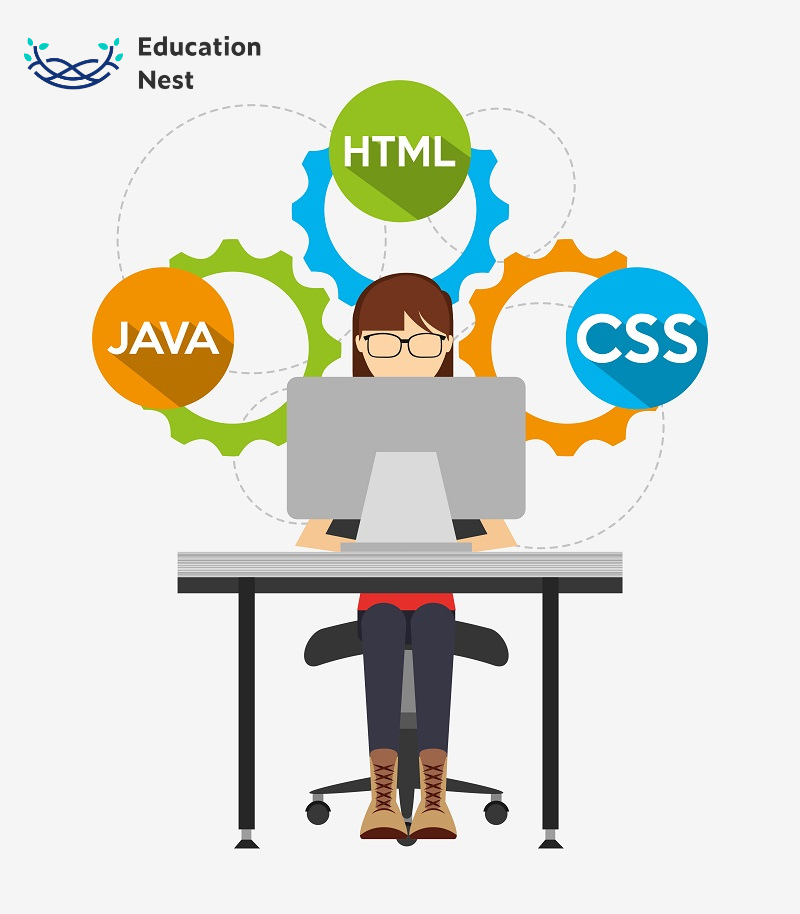
Any java technical test or interview, whether for freshers or candidates with one to three years of experience, may include these questions based on the static keyword.
1. What in Java is static?
The static keyword in Java is mostly used for memory management. Static refers to the storage of variables or procedures in a single copy.
1.Inside a class, the members that have the static keyword appended to them are referred to as static members.
2. If no instance of the class is created, can we access static members?
The static members can be accessed even in the absence of a class instance because they are not dependent on any particular instance. Each and every instance of the class shares them.
3. Is it possible to use a top-level class and the static keyword?
No, the top-level or outside classes cannot have the static keyword; however, an inner class may.
4. Will the following piece of code be correctly compiled? If so, what does the following program’s output look like?
public class Myclass
{
private int x = 10;
static int m1() {
int y = x;
return y;
}
public static void main(String[] args) {
m1();
}
}
5. Identify the error in the following code snippet. If there is no error then what will be the output of the program?
public class Myclass
{
private int x = 10;
static int m1()
{
Myclass obj = new Myclass();
int y = obj.x;
return y;
}
public static void main(String[] args) {
System.out.println(m1());
}
}
Ans: There is no error in the above code snippet. Output: 10.
6. What is the main use of static keyword in java?
Ans: The main use of java static keyword is as follows:
- The static keyword can be used when we want to access the data, method, or block of the class without any object creation.
- It can be used to make the programs more memory efficient.
7. Can we mark a local variable as static?
Ans: No, we cannot mark a local variable with a static keyword.
8. When does a static variable get memory?
Ans: When a class is loaded into the memory at runtime, the static variable is created and initialized into the common memory location only once.
9. What will be the output of the following program?
public class Myclass
{
static int a = 20;
static int b = 30;
static int c = 40;
Myclass()
{
a = 200;
}
static void m1() {
b = 300;
}
static {
c = 400;
}
public static void main(String[] args) {
System.out.println(a);
System.out.println(b);
System.out.println(c);
}
}
Ans: Output: 20, 30, 400.
10. What will be the output of the following code?
public class Myclass {
static int a = 20;
Myclass() {
a = 200;
}
public static void main(String[] args) {
new Myclass();
System.out.println(a);
}
}
Ans: Output: 200.
}
Ans: Yes, the above code will be compiled successfully. There is no problem. Output: Static method, Instance method, Constructor.
Conclusion
Java uses the “static” keyword to declare a variable or method specific to a class rather than to any particular class instance. This implies that accessing a static variable or method is possible without first establishing a class object.
When all instances of a class need to share information or functionality, static variables, and methods come in handy. As their values don’t change while the program runs, they are frequently used to define constants.
However, static variables and methods should only be used sparingly because they can make code harder to test and maintain. Moreover, if used carelessly, static variables and methods shared by all class instances might cause concurrency problems.
Ultimately, Java programming’s “static” keyword is a vital tool, but it should be utilized carefully and intelligently.