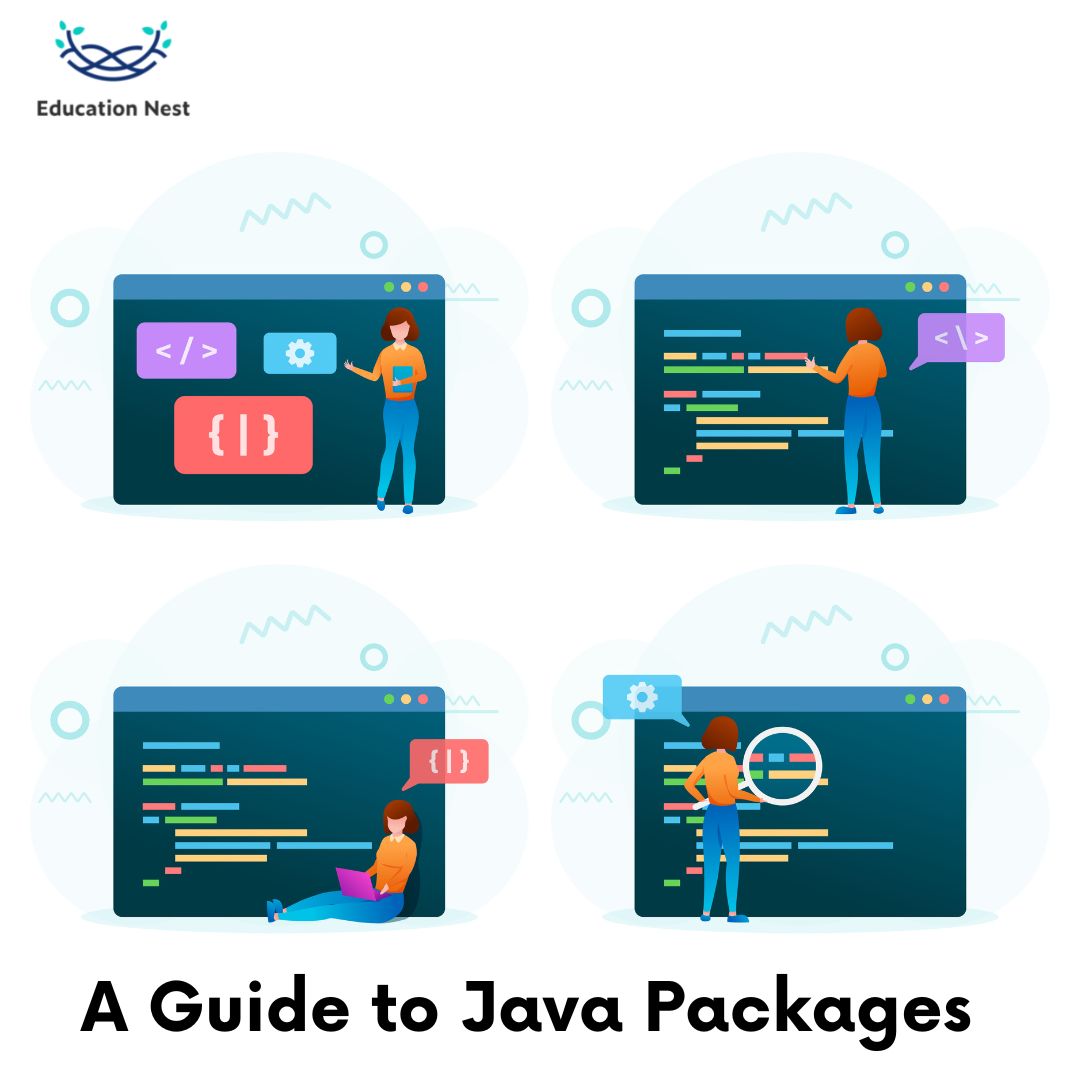
The concept of packages is one of Java’s most cutting-edge features. Java packages are a way to organize related objects like classes, interfaces, enumerations, annotations, and sub-packages. Java packages are like the file folders on your computer.
Java packages are groups of resources that work together, like classes, interfaces, and other packages.
Firstly, in Java, there are two kinds of packages:
1. In-built packages (ones made by the Java development community) and
2. User-defined packages (ones made by the end-user)
Some pre-installed packages are Java, lang, swing, net, io, util, awt, javax, SQL, etc.
This post will teach you how to work with Java packages in the most basic way.
What does a Java package mean?
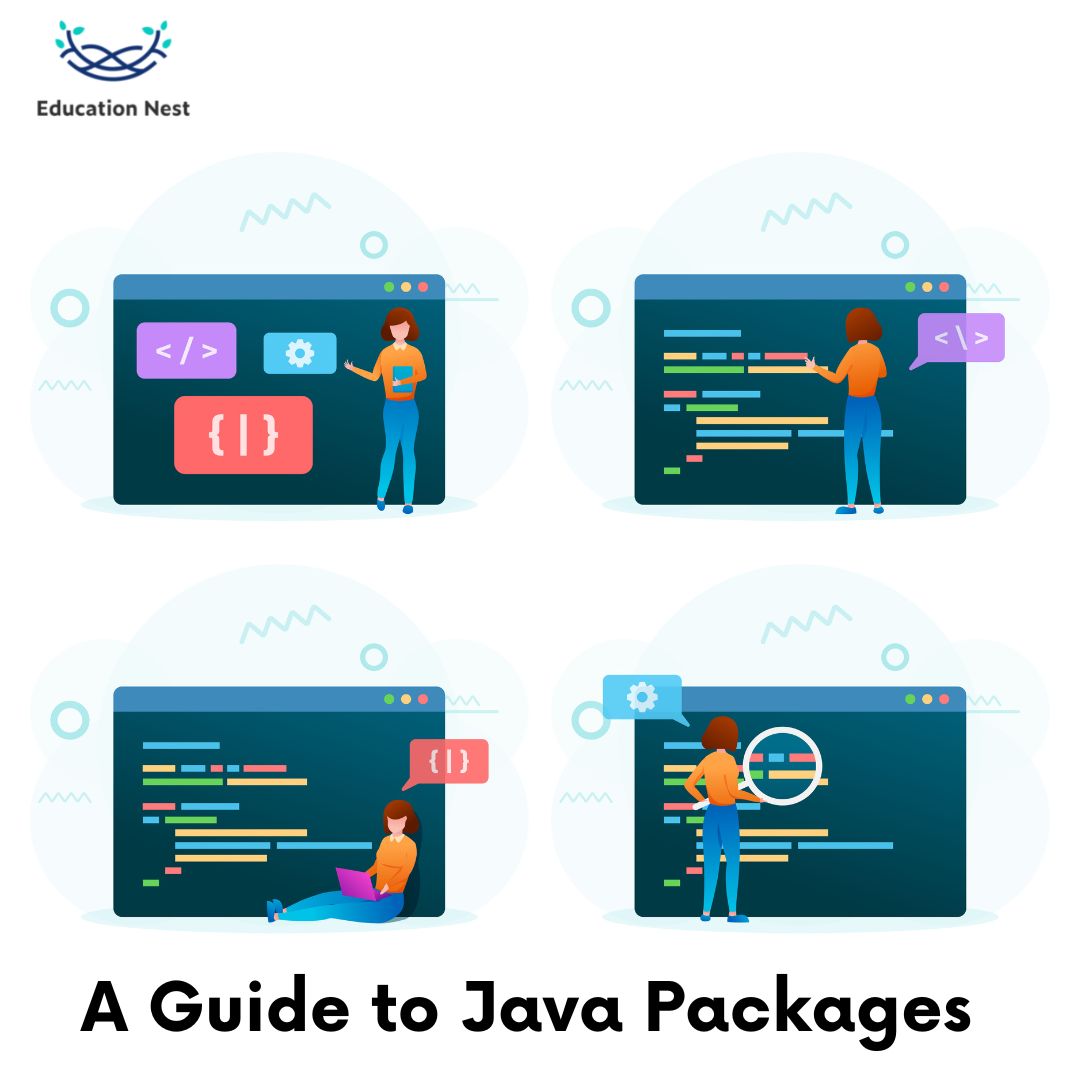
Moving forward, in Java programming, “packages” are groups of classes, sub-packages, and interfaces that share certain traits. There are two types of packages in Java: those that are already installed and those that the user makes. The likes of util, Java, IO, Net, SQL, Java, etc. are among the preinstalled packages.
This post will tell you everything you need to know about ready-made packages and the steps you need to take to make your own.
Java Package Example
For instance, we can create a Java class inside a package using the package keyword.
Package com.techvidvan.package demo; //package
class Example
{
public static void main(String args[])
{
System.out.println(“Welcome to Techvidvan’s Java Tutorial”);
}
}
Output:
Welcome to the world
Advantages of Java package
- Java packages are used to protect privacy.
- Using Java packages can help you avoid name conflicts.
- Developer-defined packages allow for the organization of related code, such as a set of classes or an interface.
- Organizing the classes, interfaces, enumerations, and annotations you build so a programmer can quickly see how they relate to one another is a good practice.
- There will be no name collisions with other packages because this package introduces a new namespace.
- Discovering similar courses is also simplified.
Java Package Types
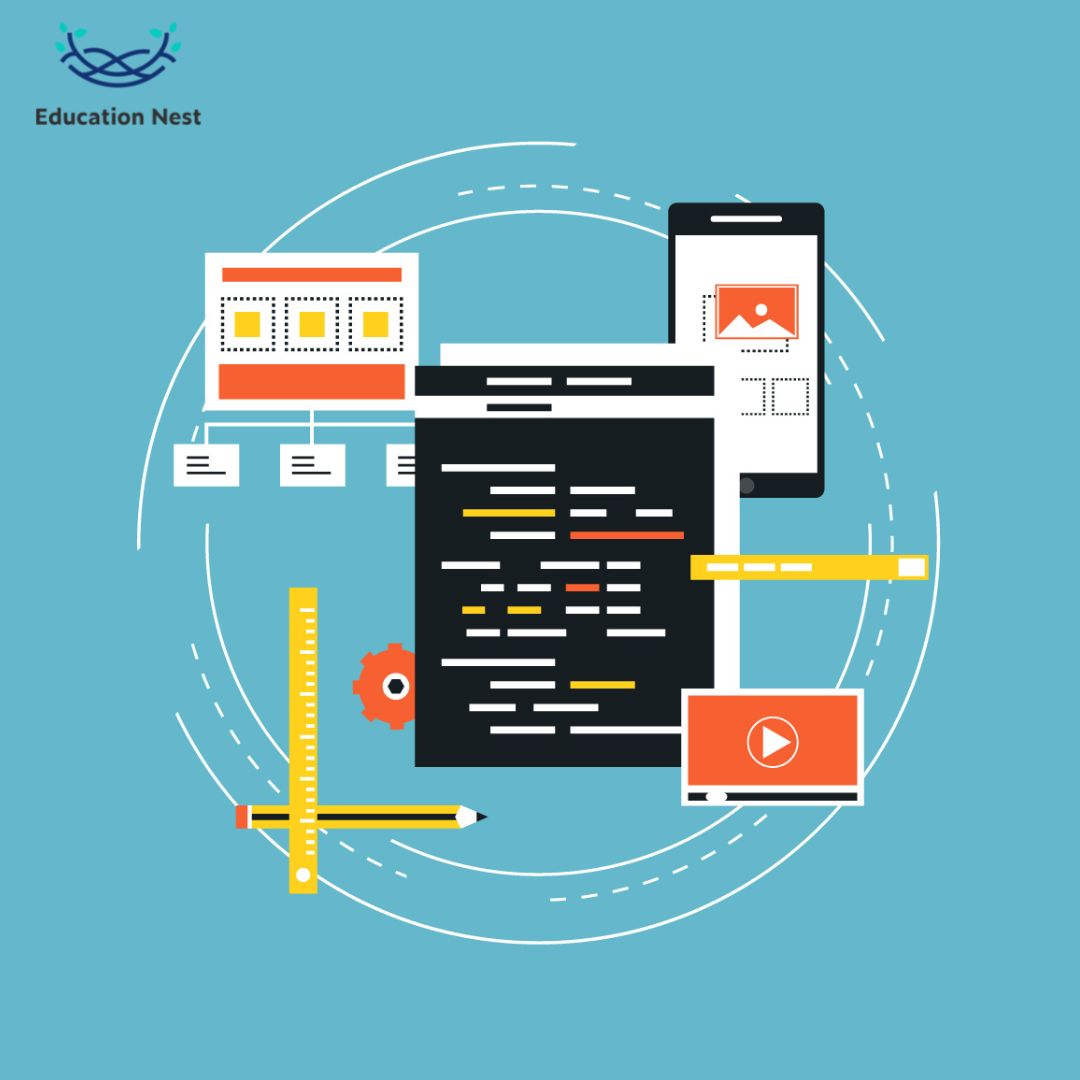
They fall into two distinct groups:
1.Java Application Programming Interface (API) packages
The extensive class library in Java is broken up into several packages, each with its own set of features.
Examples:
Primitive types, strings, mathematical functions, threads, and exceptions are all covered in Java. Lang package.
Vectors, hash tables, dates, calendars, and more can all be found in Java. Util package.
java.io: Input and output can be handled with the help of stream classes.
classes in Java. awt is used to create GUI elements like windows, buttons, menus, etc.
Connectivity-related Java APIs and classes (java.net)
Applet Classes in Java: Making and Running Your Own
Example
import java. awt.*;
public class AWTExample{
AWTExample()
{
Frame fr1=new Frame();
Label la = new Label(“Welcome to the java world”);
fr1.add(la);
fr1.setSize(200, 200);
fr1.setVisible(true);
}
public static void main(String args[])
{
Testawt tw = new Testawt();
}
}
Output: Welcome to the java world
2. User-defined packages
The developer makes user-defined packages to organize classes and other packages in a way that is easier to work with. They are a lot like Java’s built-in support. By importing it into different classes, it can be used in the same way as other built-in packages. The anonymous default package will be added to any class names that don’t have a package statement.
// Java program to create a user-defined
// package and function to print
// a message for the users
package example;
// Class
public class gfg {
public void show()
{
system.out.println (“Hello geeks! How are you?”);
}
public static void main(String args[])
{
gfg obj = new gfg();
obj.show();
}
}
Output: Hello, geeks!! How are you?
How Does One Create a Java Package?
The Java package is a container for objects like classes and interfaces related to each other. All that needs to be done is to group similar types into bundles. Then, by writing an import class, it will be easy to use existing packages.
A package is a group of linked classes, some available to the public and some hidden and only used by the program. Classes already in the packages can be used in our codebase as often as needed. Most of the time, the names of packages and the way their directories are set up go together very well.
To make a Java package, you need to do the following:
Let’s start by naming the package we’re about to create. You’ll find the package command on the first line of each Java programme.
When necessary, more classes, interfaces, annotation types, etc., can also be added to the package. For example, the package name “FirstPackage” is made with the following single line:
//Name of the package
import data.*;
//Class to which the package belongs
class ncj {
// main driver method
public static void main(String arg[])
{
// Creating an object of the Demo class
Demo d = new Demo();
// Calling the functions show() and view()
// using the object of the Demo class
d.show();
d.view();
}
}
Output: Hi Everyone
Hello
Importing a Java Package
The “java import” keyword is used in a Java program to access a Java package and its classes.
If you want your class to use a class from another package directly, you must first import that package into your Java source file using the jar file.
You can get to every class in another package in one of three ways:
- without importing the package
- import package with specified Class
- import package with all classes
Let’s look at some examples of each to get a better understanding.
1. Without import keyword accessing the package
If you import a class by its fully qualified name, you can only use that class in that package. You won’t be able to use any other classes in that package. If you go this way, you do not need an import statement. But when you call a class or interface, you must always use the full name. This is usually done when classes in two different packages have the same Both thva.util and javJava.sqlckages have the Date class.
Example
We’ll make Class A in the pack package and create a Class B instance that uses Class A.
//save by A.java
package pack;
public class A {
public void msg() {
System.out.println(“Hello”);
}
}
//save by B.java
package mypack;
class B {
public static void main (String main(String args[]) {
pack.obj = new pack. A(); //using fully qualified name
obj.msg();
}
}
Output: Hello
2. Import the Specified Class
Java lets us add the class name to the package name so that we can only access one class in our application, even though a package can contain more than one class. The only class that can be used from that package is the one whose name is given in the import packagename.class name statement.
Here’s an example: we have a class called Demo that we’ve placed in the pack package, and we want to use the methods from Demo in another class called Test.
//save by Demo.java
package pack;
public class Demo {
public void msg() {
System.out.println(“Hello”);
}
}
//save by Test.java
package mypack;
import pack.Demo;
class Test {
public static void main (String main(String args[]) {
Demo obj = new Demo();
obj.msg();
}
}
Output: Hello
3. Import all of the classes in the package
Using the packagename.* statement, you can search through packages. This gives you access to all classes and interfaces inside the given package, but not to any classes or interfaces inside any sub-packages.
Using the import keyword, classes from another package can be used in the current package.
Here’s a simple example of using the import keyword to use a class you’ve already made from another class.
//save by First.java
package learn Java;
public Class First{
public void msg() {
System.out.println(“Hello”);
}
}
//save by Second.java
package Java;
import learnjava.*;
class Second {
public static void main (String args []) {
First obj = new First();
obj.msg();
}
}
Output: Hello
Conclusion
Java packages are an important part of the Java programming language. They help programmers organize their code in a modular and hierarchical way. When classes and interfaces are put into packages, the code is easier to read, reuse, and keep safe. Packages also make it easier to manage and keep up with large projects by reducing the chance of name collisions. Packages are an indispensable resource when developing reliable, scalable, and easily maintainable Java applications.
We now understand what a package is and why it is functional. Undoubtedly, the Java package is essential to being a good Java developer. It improves the programmer’s coding style and eliminates a lot of unnecessary tasks.
For more interactive topics, visit educationnest.com