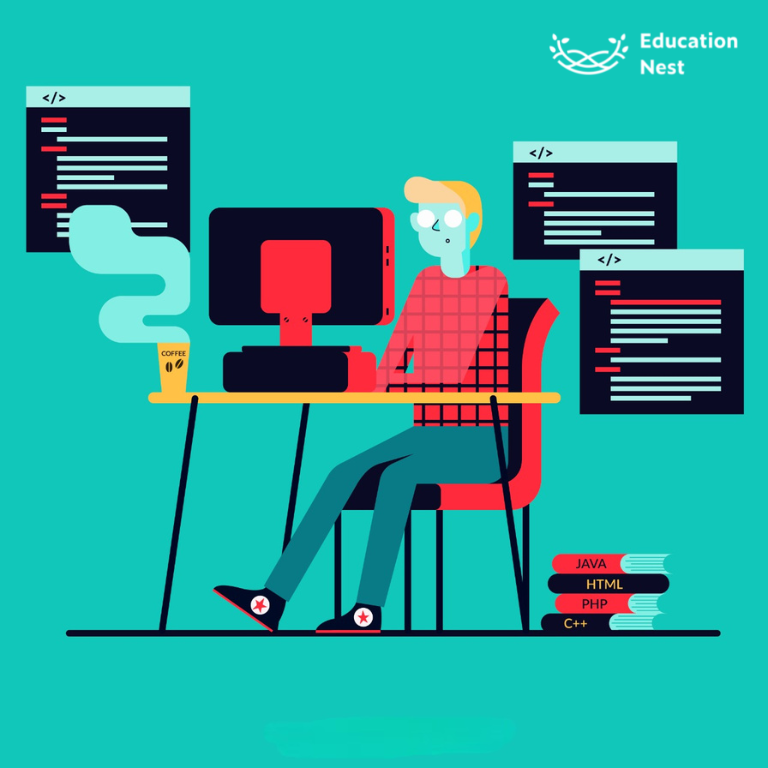
This blog will cover some of the most common and complex Java interview questions that software developers are asked, from Java interview questions for 2-3 years of Experience to Java interview questions for ten years of experience.
The kinds of questions that are asked in Java interviews have changed in the past few months. Questions are getting more challenging, and interviewers are asking more in-depth questions. The days of knowing the difference between String and StringBuffer could help you advance to the next round of interviews are long gone. Earlier, it was common to prepare for job interviews by memorizing answers to questions about how vectors, arrays, hashmaps, and hash tables are different. These days, you might be asked about NIO, patterns, advanced unit testing, and more difficult-to-understand programming topics like concurrency, algorithms, data structures, and coding.
What is precisely Java Technology?
Sun Microsystems came out with Java, a computer programming language and platform, in 1995. Since its early days, it has grown to be the backbone of the modern internet, giving many services and programs a stable place to run. Java is still important for making digital products and services that are cutting-edge and focused on the Future.
Even though modern Java programmes are improving at combining the Java runtime and the application, some programmes and websites can still only be used with a desktop Java installation. This website, Java.com, is for people who still need Java for desktop apps, especially ones made for Java 8. For more information, business customers should go to oracle.com/java. Developers and people who want to learn how to program in Java should go to the dev.java website.Questions in the interview will be about things from the beginning, intermediate, and advanced levels of the Java programming language.
Frequently Asked Java Interview Questions
- Sample Java interview questions will get you started by covering the following topics:
- How threads, multiple tasks, and simultaneous processing work
- Dates and date formats: the basics
- Pickup of Garbage
- Using Collections in Java Framework Structured Design Patterns for Arrays and Strings from the Governing Organization for Internet Design, based on the SOLID principles
- Abstraction of classes and interfaces
- Fundamentals of the Java programming language, like the equals() and hashcode
- Generic words and listing
- NIO and Java’s I/O
- Networks use standard protocols.
- Algorithms in Java and Data Structure
- Regular Expressions Are Used
- An inside look at the Java Virtual Machine
- Advice on how to programme in Java
Java Interview Questions for 2 to 3 Years Of Experience
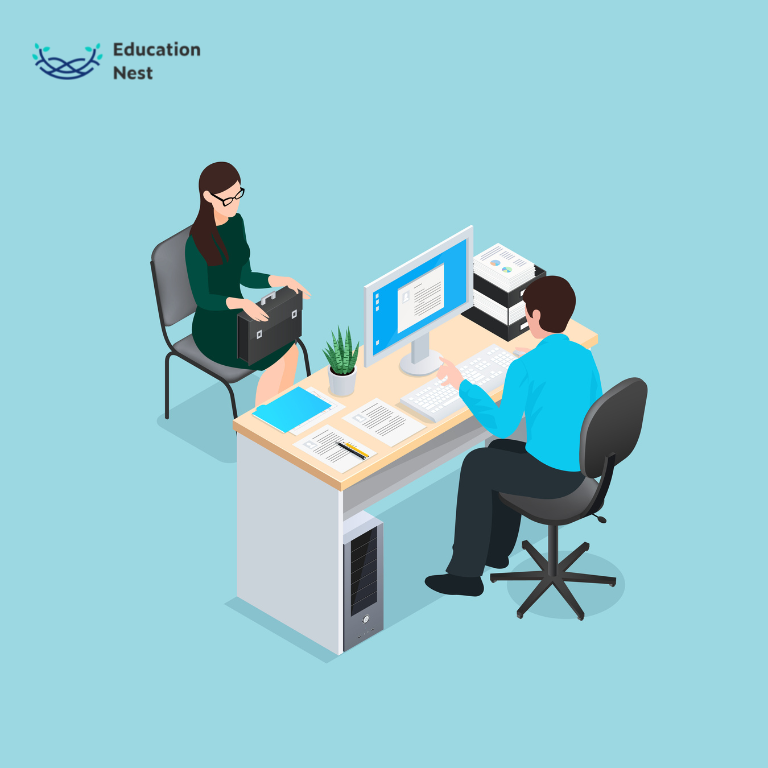
Since 2–3 years is not a long time and you are still in the beginner–intermediate range, you won’t be judged harshly if you haven’t done things like profile apps, tune the Garbage Collection, make complex Java programs, or develop concurrent Java design patterns. A Java developer with 2–4 years of experience should know about OOPS concepts like Abstraction, Encapsulation, Polymorphism, Composition, and Inheritance. They should also understand the Java collection API and the different types of collections, like List, Set, and Map, and be familiar with popular Collection classes like ArrayList, HashMap, Vector, HashSet, etc.
Interviewers for intermediate Java jobs also look for candidates who have a firm grasp of the language’s threading API, wait-notify mechanism, and exception handling, in addition to the expected skill with basic programming and coding exercises like finding the length of a linked list in a single pass, inverting a String in Java, etc.
Q1. How do you tell the difference between a Vector and an ArrayList in Java?
This is a general Java question for developers with two years of experience. It is meant to test how well you know the Java collection API. One crucial point is that ArrayList is slow compared to Vector, which can be synced quickly. Please read the above articles to learn more about how Java’s Vector and ArrayList classes differ.
Q2. What is the difference between Java’s ArrayList and LinkedList?
This question is often asked in Java interviews of people with two to three years of experience. In contrast to synchronization, here you should stress the underlying data structure’s importance.
Q3. What is the difference between a fail-fast Iterator and a fail-safe Iterator in Java?
It’s a newer Java question than others. Still, candidates with 2 to 3 years of Experience in Java are being asked about it more often in interviews. The main difference here is that the fail-fast Iterator throws a ConcurrentModificationException while the fail-safe Iterator does not. See the fail-safe Iterator and fail-fast Iterator to learn more about the differences between these two Iterators in Java.
Q4. What’s the difference between Java’s keywords “throw” and “throws”?
This is a Java question about handling exceptions, a common subject for developers with 2–4 years of experience. One says what kinds of exceptions a Java method can throw, and the other is used to throw those exceptions.
Q5. How about a Java programme that shows the Fibonacci sequence?
You will almost always be asked about your coding skills at interviews that require 2–4 years of Java experience. Even though the Fibonacci series is used a lot and doing it through recursion only makes the problem harder, not every Java programmer can correctly implement it in an interview.
Q6. How can you write a Java programme to flip a String without using StringBuffer?
Another Java programming question for people who have worked with the language for 2–4 years. Interviewers often mentioned that StringBuffer has a reverse() method that makes these tasks easy and said that you shouldn’t use StringBuffer. So, to be good at it, you must review iteration and recursion.
Q7. What is the basic difference between Java’s Runnable and Thread classes?
Questions about threading that are often asked of Java developers with 2 to 4 years of experience Thread can be used in Java in not just one, but two different ways. You could implement either Java. lang or the Thread class as an interface that can be run or called.
Anyone working with Java development for at least five years should know the language inside and out.
Java Interview Questions for 5 years of Experience
Q1. What do you think are Java 8’s most important new features?
Java 8, which came out in 2014, is still used by almost all programs. It is open-source, can be used for business, and has many valuable features like static methods and functional interfaces. Adding object-oriented interfaces to available interfaces has made programming more accessible and practical.
Q2. What exactly is a Java Class? What are some of the most common Java classes you could tell us about?
In Java, classes are groups of objects with similar behaviors or properties. It is often where designers who work in object-oriented languages start. Java classes like static, final, concrete, inner, abstract, and POJO are often used when making apps.
Q3. What are the most important things you need to know about wrapper classes?
When learning about wrapper classes, the most important things to understand are the eight basic types of wrapper classes and when to use each. When a wrapper class is given, it becomes final and can’t be changed anymore.
Q4. How do you use the four Java access modifiers?
You can use access modifiers in Java like default, public, private, or protected. They are used to sort which keywords or pieces of information within a class can be seen by which users.
Q5. Explain how the Java Virtual Machine and the Java Development Kit (JDK) differ.
A Java Virtual Machine (JVM) is needed to run Java on a device. A Java Development Kit (JDK) is a set of tools that can be used to make apps that can be put on mobile devices and used there.
Q6. How do you define platform independence?
If your app is platform-independent, it will work well on any operating system. Java is the best programming language because it can be used in many different ways.
Q7. Why can’t strings be changed?
A string that can’t be changed is a thread-safe data structure. Unless the string is immutable, a change to the value of one reference will affect all other considerations.
Q8. What’s the difference between a class that is abstract and a class that is an interface?
In response, an interface and an abstract class are different. The first is the process of doing something. Interfaces and abstract classes have methods, but only abstract classes can use those methods. An abstract class can be used to make the interface work.
Q9: Can you explain a ternary operator and how it works?
Java gives you a number of if/then statements as a solution. A ternary operator decides which of three possible values should be given to a variable.
Q10. Can you explain serialization and deserialization briefly?
Managing data streams in Java is done with the help of serialization and deserialization. To “serialize” something means turning it into a byte stream so it can be stored or moved. Deserialization is the process of going back to a state that was not serialized.
Java Interview Questions for 10 + years of experience
Q. What’s wrong with using HashMap in a setting with multiple threads? What starts the loop in the get() method that goes on forever?
Actually, it’s OK; it depends on what you’re talking about. For example, it’s OK if only one thread starts making a HashMap, and all the other threads only read from it after that. One example of this would be a Map with configuration properties. When at least one of these threads updates HashMap—that is, when it adds, changes, or removes any key-value pair—things get messy. Since the put() operation can cause a resize, which can lead to an infinite loop, you should use Hashtable or ConcurrentHashMap, with the latter being better.
Q. If you don’t override the hashCode() method, does that hurt performance?
This is an excellent question that anyone can answer. A lousy hash code function causes a HashMap that is slow because of a lot of collisions.
In Java 8 and later, collisions have less of an effect on performance because, at a certain point, the linked list is replaced with a binary tree, which has a worst-case version of O(logN) instead of O(n) for a linked list.
Q. Does every member of an object that can’t be changed in Java have to be final?
As the linked response article explains, it is unnecessary because the same functionality can be achieved by making a member non-final but private and preventing any changes to it outside of the function Object() [native code].
If the object can be changed, you shouldn’t leak a reference to any of its members, and you shouldn’t give them a setter function.
Remember that finalizing a reference variable only ensures that it will never get a new value. You can’t change the value of a reference variable, but you can change the features of the object it points to.
Q. Where does the () substring operator come into play in a String?
Here’s another great Java interview question. The answer is not good enough, but here it is anyway. “Substring” does what its name says: it takes a piece of the source string and uses it to make a new object.
The main reason for this question was to determine whether the developer is aware that sub-strings can cause a memory leak.
Until Java 1.7, the substring keeps a reference to the original character array, so even a five-character extended substring can stop a 1GB character array from being trashed.
To fix this in Java 1.7, references to the original character array had to be removed, which slowed down the process of making substrings. Before Java 7, it was about O(1). In the worst case, it could be O(n).
You Must Like: Java Programs: From Basic to Advanced, How to Write Better Code
Q. What is the algorithm for the singletons’ critical section?
This classic Java interview question usually asks for an example of double-checked locking in a Java singleton.
Use a volatile variable to make sure that your Singleton is thread-safe.
In a thread-safe Singleton pattern, the phrase “double-checked locking” is used in the following way.
Singleton is a public class that declares the following: Private static volatile Singleton instance;/** * Verified locking code for Singleton * @return Singleton instance */public static Singleton getInstance () if (_instance == null), then return null (null) _instance = new Singleton(); return _instance; If you have this code:
You should also know about classic design patterns like Singleton, Factory, and Decorator.
Q. What’s the difference between Executor. execute() and Executor? submit()?
This is one of the most common questions about multi-threaded programming in Java interviews. As the need for Java programmers who are good at concurrency grows, so does the popularity of this method. This answer to a Java interview question explains that this is what the former process does, which returns a Future object and can be used to get the result from a worker thread. When you look at how exceptions are handled, you can see that there is a difference. If one of your jobs throws an exception, control will go to the uncaught exception handler (if you haven’t set one, System.err will be printed instead).
Any exception thrown, whether checked or not, is included in the return status of a task that was sent with the submit() method.
Suppose an error happens while a submitted task runs in the Future. The get () method will re-throw the error wrapped in an ExecutionException.
Q. What is the main difference between a factory pattern and an abstract one?
Abstract Factory is a valuable tool that lets you think of things more broadly. Think about the factories that extend Abstract Factory and are responsible for making things at different levels in the hierarchy. For example, other factories, such as CarFactory, UserFactory, RoleFactory, etc., can improve how AbstractFactory works. Every factory would be responsible for making its unique line of goods.
Conclusion
Java is one of the most widely used programming languages in the world. More than 45 billion virtual machines are running Java programmes at any time. It is the most popular language for making microservices. It is also used extensively in DevOps, AI, VR, Big Data, Continuous Integration, Analytics, Mobile, Chatbots, and other areas.
With more than 9 million active Java developers worldwide, the need for Java developers is at an all-time high. Mercer, a global leader in evaluating talent, says that a Java developer will be one of the most in-demand jobs in 2023. This is because Java is increasingly used in many different areas, devices, and organizations.
For a Java interview with at least five years of experience, prepare well. Spend ample time studying JavaScript’s unique domain knowledge, programming data structures and algorithms, system architecture, and leadership and behavioral interviewing techniques.