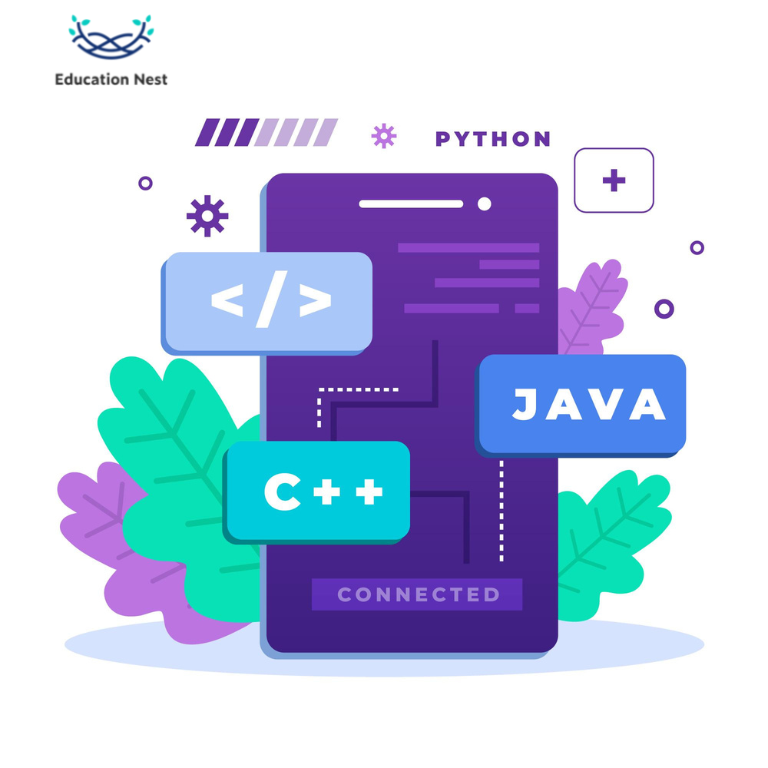
Java Programs, an object-oriented, class-based, general-purpose programming language, was designed to be simple. It is a platform for creating computer programs. As a result, Java is quick, secure, and dependable. It is frequently used to develop Java applications for smartphones, game consoles, laptop computers, data centers, and other devices.
The Java Platform is a collection of programs that make it simple for developers to create and run Java programs. It includes a compiler, a program execution engine, and several libraries. It consists of a set of requirements as well as software. James Gosling created Sun Microsystems’ Java platform, and Oracle Corporation eventually purchased it.
The following are some of the most important Java programs:
It is used to create applications for Android devices.
allows you to create a variety of mobile Java apps and business software
Use Hardware Devices for Java Programming for Big Data Analytics by server-side technologies such as GlassFish, JBoss, and Apache.
In this article we will study about Java Programs using String,For loop,Charat etc .
Before framing the programs lets go through about Java Compilers.
What are Java Compilers?
A Java compiler application converts a developer’s text file work into a platform-independent Java file. The Java Programming Language Compiler (javac), the GNU Compiler for Java (GCJ), the Eclipse Compiler for Java (ECJ), and Jikes are all examples of Java compilers.
The compiler parses and analyzes each statement in the language from a syntactic standpoint before generating the output code at runtime. The compiler generates the output code in one or more “passes,” ensuring that any statements that refer to other statements are correctly referenced in the output code.
Java compilers are typically started and pointed to a programmer’s source code in a text file to generate a class file that the Java virtual machine (JVM) can use on multiple platforms. Jikes, an open-source compiler, is an example of this.
Java Compiler Online –
Codiva
Codiva.io is our top recommendation for an online Java compiler. Even though it is new to the game, it has many great features.
Codiva’s most intriguing feature is that it compiles as you type, reads compilation errors, and displays them in the editor. The compilation results are shown as soon as you finish typing.
Additionally, autocompletion works quite well. These two features will save you significant time when writing code.
Codiva allows for unique file names and supports many files and packages. You can also run interactive programs. As a result, Codiva can teach you most of what you need to know to begin the Java and algorithms course.
JDoodle
JDoodle is a well-known Java compiler that is available online. Initially designed for Java, it now supports nearly 70 languages. You can only upload one file to JDoodle, but it does not need to be named. It finds it by inspecting the contents of the file.
JDoodle provides excellent terminal support for interactive programs. By default, the programs operate in a mode where you cannot do anything and only have 10 seconds to do so. However, if you need to switch to an interactive way for a specific project, you can do so.
JDoodle is an excellent choice if you enjoy learning a variety of programming languages or switching between them frequently.
Rextester
Rextester began as a Means To implement Tester before developing into an online IDE. It supports about 30 languages, including Java, but C# users are likelier to use it.
Rextester allows you to select which editor widgets to use based on your requirements.
It provides one of the best ways to collaborate in real-time. Begin typing and provide the URL to others. I have not found any bugs, and more than one person can edit simultaneously.
The class’s name should be Rextester, and it should only work with one file. Nobody else should be shown the class either. It only works with Java 8 at the time of writing.
OnlineGDB
The tool that assists with online debuggers is known as Online GDB. If you create a complex program and need to fix it if something goes wrong, the debugger will come in handy.
When you set a breakpoint, the program will halt at that line, run step by step, and display the variable values at each step.
It also includes a code formatter that automatically formats the code. However, you cannot select your preferred code style.
Only Java 8 will function. Even though the compilation is slow, it is still worthwhile to try.
Browxy
Browxy was popular before it fell behind. Several file types can be used. There is currently only Java 8.
Just Browxy is a web browser with almost no limitations. URLs from outside the network can also be queried.
This is a great place to start if you want to improve your API request skills. Applets are supported, but they have yet to be used.
IDEOne
IDEOne is one of the first online compilers that are still used today. So, it’s worth taking note of.
About 60 or more languages can be used. Ideone has yet to be made to work with Java 9. IDEOne has an API for compilation as a service that you can use to make your online IDE.
For loop programming –
A section of the program is repeatedly iterated using the Java for loop. A for loop is advised if the number of iterations is fixed.
In Java, there are three distinct forms of for loops.
- Simple for Loop
- For-each or Enhanced for Loop
- Labeled for Loop
Example 1: Display a Text Five Times
// Program to print a text 5 times
class Main {
public static void main(String[] args) {
int n = 5;
// for loop
for (int i = 1; i <= n; ++i) {
System.out.println(“Java is fun”);
}
}
}
Output
Java is fun
Java is fun
Java is fun
Java is fun
Java is fun
Example 2: Display numbers from 1 to 5
// Program to print numbers from 1 to 5
class Main {
public static void main(String[] args) {
int n = 5;
// for loop
for (int i = 1; i <= n; ++i) {
System.out.println(i);
}
}
}
Output
1
2
3
4
5
Java for-each Loop
The Java for loop has an alternative syntax that makes it easy to iterate through arrays and collections. For example,
// print array elements
class Main {
public static void main(String[] args) {
// create an array
int[] numbers = {3, 7, 5, –5};
// iterating through the array
for (int number: numbers) {
System.out.println(number);
}
}
}
Output
3
7
5
-5
Java Infinite for Loop
If we set the test expression in such a way that it never evaluates to false, the for loop will run forever. This is called infinite for loop. For example,
// Infinite for Loop
class Infinite {
public static void main(String[] args) {
int sum = 0;
for (int i = 1; i <= 10; –i) {
System.out.println(“Hello”);
}
}
}
Here, the test expression ,i <= 10, is never false and Hello is printed repeatedly until the memory runs out.
Java Programmings using Charat method-
String charAt in Java ()
The Java String class charAt() function returns a char value at the supplied index number.
The index number indicates the string’s length, n, which ranges from 0 to n-1. If the provided index number is less than, equal to, or a negative value, it throws a StringIndexOutOfBoundsException.
Let’s see Java program related to string in which we will use charAt() method that perform some operation on the given string.
FileName: CharAtExample.java
public class CharAtExample{
public static void main(String args[]){
String name=“javatpoint”;
char ch=name.charAt(4);//returns the char value at the 4th index
System.out.println(ch);
}}
Output:
t
FileName: CharAtExample.java
public class CharAtExample{
public static void main(String args[]){
String name=“javatpoint”;
char ch=name.charAt(10);//returns the char value at the 10th index
System.out.println(ch);
}}
Output:
Exception in thread “main” java.lang.StringIndexOutOfBoundsException:
String index out of range: 10
at java.lang.String.charAt(String.java:658)
at CharAtExample.main(CharAtExample.java:4)
Let’s see an example where we are accessing all the elements present at odd index.
FileName: CharAtExample4.java
public class CharAtExample4 {
public static void main(String[] args) {
String str = “Welcome to Javatpoint portal”;
for (int i=0; i<=str.length()-1; i++) {
if(i%2!=0) {
System.out.println(“Char at “+i+” place “+str.charAt(i));
}
}
}
}
Output:
Char at 1 place e
Char at 3 place c
Char at 5 place m
Char at 7 place
Char at 9 place o
Char at 11 place J
Char at 13 place v
Char at 15 place t
Char at 17 place o
Char at 19 place n
Char at 21 place
Char at 23 place o
Char at 25 place t
Char at 27 place l
The position such as 7 and 21 denotes the space.
String Programming-
In Java, a string is a group of characters that work together. For example, “hello” is a string made up of the letters “h,” “e,” “l,” “o,” and “l.”
In Java, we use double quotes to show a string.
1. Get length of a String
To find the length of a string, we use the length() method of the String. For example,
class Main {
public static void main(String[] args) {
// create a string
String greet = “Hello! World”;
System.out.println(“String: ” + greet);
// get the length of greet
int length = greet.length();
System.out.println(“Length: ” + length);
}
Output
String: Hello! World
Length: 12
2. Join Two Java Strings
We can join two strings in Java using the concat() method. For example,
class Main {
public static void main(String[] args) {
// create first string
String first = “Java “;
System.out.println(“First String: ” + first);
// create second
String second = “Programming”;
System.out.println(“Second String: ” + second);
// join two strings
String joinedString = first.concat(second);
System.out.println(“Joined String: ” + joinedString);
}
Output
First String: Java
Second String: Programming
Joined String: Java Programming
3. Compare two Strings
In Java, we can make comparisons between two strings using the equals() method. For example,
class Main {
public static void main(String[] args) {
// create 3 strings
String first = “java programming”;
String second = “java programming”;
String third = “python programming”;
// compare first and second strings
boolean result1 = first.equals(second);
System.out.println(“Strings first and second are equal: ” + result1);
// compare first and third strings
boolean result2 = first.equals(third);
System.out.println(“Strings first and third are equal: ” + result2);
}
}
Output
Strings first and second are equal: true
Strings first and third are equal: false
How to Reverse String in Java?
Below are the nine common methods by which you can reverse a string in java:
1) Using StringBuilder
In Java, we can reverse a string by first converting it to StringBuilder, which is just a mutable string. Reverse(), a built-in function of the class StringBuilder, allows you to reverse a specified string and get the result. Hence, we will use this built-in method reverse() to reverse the StringBuilder and then use the built-in function function toString() { [native code] } to convert the reversed StringBuilder to String ().For example:
public class ReverseStringByFavTutor
{
public static void main(String[] args) {
String stringExample = “FavTutor”;
System.out.println(“Original string: “+stringExample);
// Declaring a StringBuilder and converting string to StringBuilder
StringBuilder reverseString = new StringBuilder(stringExample);
reverseString.reverse(); // Reversing the StringBuilder
// Converting StringBuilder to String
String result = reverseString.toString();
System.out.println(“Reversed string: “+result); // Printing the reversed String
}
}
Output
Original string: FavTutor
Reversed string: rotuTvaF
2) Swapping characters
Using this strategy, we will first use the built-in string function toCharArray to transform the original string into a character array (). To create the supplied string’s reverse, we will swap the first character with the last one, the second with the next-to-last character, and so on. Later, we will transform the reversed character array to a string using the built-in function String.valueOf().For example:
public class ReverseStringByFavTutor
{
public static void main(String[] args) {
String stringExample = “FavTutor”;
System.out.println(“Original string: “+stringExample);
// Converting String to Character Array
char str[] = stringExample.toCharArray();
int n = str.length; // length of character array
int start=0,end = n-1;
while(start<=end){
// Swapping the characters
char temp = str[start];
str[start] = str[end];
str[end] = temp;
start++;
end–;
}
// Converting characterArray to String
String reversedString = String.valueOf(str);
System.out.println(“Reversed string: “+reversedString); // Printing the reversed String
}
}
Output:
Original string: FavTutor
Reversed string: rotuTvaF
3) Creating a new String
We will make a new string and use a for loop to iterate through the original string to reverse a string in Java. We shall concatenate every character from the starting string to the beginning of the new string as we iterate.The new string formed will be the reversed form of the original string as shown in the example below:
For example:
public class ReverseStringByFavTutor
{
public static void main(String[] args) {
String stringExample = “FavTutor”;
System.out.println(“Original string: “+stringExample);
int n = stringExample.length(); // length of String
String reversedString =””;
char character;
for(int i=0;i<n;i++){
//extracts each character
character= stringExample.charAt(i);
//concatenates each character in front of the new string i.e. reversedString
reversedString = character+reversedString;
}
System.out.println(“Reversed string: “+reversedString); // Printing the reversed String
}
}
Output
Original string: FavTutor
Reversed string: rotuTvaF
4) Using a List
Using an internal function called toCharArray, we will first convert the original string into a character array in this method (). After that, a Character ArrayList will be created. And fill the ArrayList with the entire string’s characters. Because the class Collections in the Java Collections Framework includes the built-in function reverse(), we’ll utilize that method to reverse the ArrayList.
For example:
import java.util.*;
public class ReverseStringByFavTutor
{
public static void main(String[] args) {
String stringExample = “FavTutor”;
System.out.println(“Original string: “+stringExample);
char str[] = stringExample.toCharArray();
int n = str.length; // length of characterArray
// Declaring ArrayList of Characters
ArrayList<Character> list = new ArrayList<>();
for(int i=0;i<n;i++){ // Iterating through characterArray and adding character into list
list.add(str[i]);
}
Collections.reverse(list); // Reversing list
int size = list.size(); // size of ArrayList
System.out.print(“Reversed string: “);
for(int i=0;i<size;i++){
// Printing characters from ArrayList in reversed manner
System.out.print(list.get(i));
}
}
}
Output
Original string: FavTutor
Reversed string: rotuTvaF
5) Using Stack
Using this method, we’ll create a Stack object of characters and call the stack’s built-in push function to push every character from the original string onto the stack (). Characters will be popped out in reverse order since the stack adheres to the “First In Last Out” principle. As a result, we will make a new string, pop every character out of the stack, and concatenate them together.
For example:
import java.util.Stack;
public class ReverseStringByFavTutor
{
public static void main(String[] args) {
String stringExample = “FavTutor”;
System.out.println(“Original string: “+stringExample);
int n = stringExample.length(); // length of String
Stack<Character> stack = new Stack<>(); // Creating a stack object
for(int i=0;i<n;i++){
// inserting characters of string one by one
stack.push(stringExample.charAt(i));
}
String reversedString = “”;
// popped characters will be in reversed order
while(!stack.isEmpty()){
reversedString+=stack.pop();
}
System.out.println(“Reversed string: “+reversedString); // Printing the reversed string
}
}
Output
Original string: FavTutor
Reversed string: rotuTvaF
6) Using StringBuffer
A string can be reversed by first converting it to a StringBuffer, a mutable string. Reversing the StringBuffer is possible using the built-in function reverse() provided by the class StringBuffer. Later, we’ll use the built-in function toString() { [native code] } to convert the reversed StringBuffer to a String (). For a better understanding of StringBuffer, see the sample below.
For example:
import java.util.*;
public class ReverseStringByFavTutor
{
public static void main(String[] args) {
String stringExample = “FavTutor”;
// Converting from String Object to StringBuffer
StringBuffer reversedStringBuffer = new StringBuffer(stringExample);
reversedStringBuffer.reverse(); // reversing string
System.out.println(“Original String: “+stringExample);
System.out.println(“Reversed String: “+reversedStringBuffer.toString());
}
}
Output
Original string: FavTutor
Reversed string: rotuTvaF
7) Using XOR operation
Using the function toCharArray, we will change the initial string in this technique into a character array (). Afterward, we’ll preserve two-pointers with lows corresponding to starting indices and highs corresponding to ending indices. By iterating through array of characters and using the XOR () operator, we may swap the first and last characters, the second and second-last characters, and so on to output the reverse text, as is demonstrated below.
For example:
import java.util.*;
public class ReverseStringByFavTutor
{
public static void main(String[] args) {
String stringExample = “FavTutor”;
System.out.println(“Original string: “+stringExample);
// Converting String to Character Array
char[] str = stringExample.toCharArray();
int low = 0;
int high = str.length – 1;
while (low < high) {
str[low] = (char) (str[low] ^ str[high]);
str[high] = (char) (str[low] ^ str[high]);
str[low] = (char) (str[low] ^ str[high]);
low++;
high–;
}
System.out.print(“Reversed string: “);
//display reversed string
for (int i = 0; i < str.length; i++) {
System.out.print(str[i]);
}
}
}
Output
Original string: FavTutor
Reversed string: rotuTvaF
8) Converting to a byte array
Using the built-in Java function getBytes(), we will convert the original String to a byte array to obtain the reverse String and then build a new byte array to store the outcome. The final step is to convert the resulting byte array to String by constructing a new String object and passing the resulting byte array in the function Object() { [native code] }. The last element of the original byte array will be inserted at index “0” of the resulting byte array, the second final at index “1,” and so on.
For example:
import java.util.*;
public class ReverseStringByFavTutor
{
public static void main(String[] args) {
String stringExample = “FavTutor”;
System.out.println(“Original string: “+stringExample);
byte[] byteArray = stringExample.getBytes();
byte[] result = new byte[byteArray.length];
// Store result in reverse order into the
// result byte[]
for (int i = 0; i < byteArray.length; i++)
result[i] = byteArray[byteArray.length – i – 1];
System.out.println(“Reversed string: “+new String(result));
}
}
Output
Original string: FavTutor
Reversed string: rotuTvaF
9) Using recursion
Using recursion to reverse a string is one of the most interesting ways. Here, it would be best to create a function that only takes one argument, the original string, and makes it recursive. The function will return the original string if the string is null or has a length of less than or equal to one. Otherwise, it will print the string’s last character and call the function repeatedly. See the example below to get a better idea of what I mean.
For example:
import java.util.*;
public class ReverseStringByFavTutor
{
static void reverse(String stringExample)
{
if ((stringExample==null)||(stringExample.length() <= 1))
System.out.println(stringExample);
else
{
System.out.print(stringExample.charAt(stringExample.length()-1));
reverse(stringExample.substring(0,stringExample.length()-1));
}
}
public static void main(String[] args) {
String stringExample = “FavTutor”;
System.out.println(“Original string: “+stringExample);
System.out.print(“Reversed string: “);
// recursive function call
reverse(stringExample);
}
}
Output
Original string: FavTutor
Reversed string: rotuTvaF
Java Programmings using Scanner Class
The java.util package contains the Scanner class, which is used to gather user input.
You can utilise any of the various methods listed in the Scanner class documentation by creating an object of the class and using it. The nextLine() method, which is used to read strings, will be utilised in our example:
Example 1: Read a Line of Text Using Scanner
import java.util.Scanner;
class Main {
public static void main(String[] args) {
// creates an object of Scanner
Scanner input = new Scanner(System.in);
System.out.print(“Enter your name: “);
// takes input from the keyboard
String name = input.nextLine();
// prints the name
System.out.println(“My name is ” + name);
// closes the scanner
input.close();
}
}
Output
Enter your name: Kelvin
My name is Kelvin
Import Scanner Class
As we can see from the above example, we need to import the java.util.Scanner package before we can use the Scanner class.
import java.util.Scanner;
Create a Scanner Object in Java
Once we import the package, here is how we can create Scanner objects.
// read input from the input stream
Scanner sc1 = new Scanner(InputStream input);
// read input from files
Scanner sc2 = new Scanner(File file);
// read input from a string
Scanner sc3 = new Scanner(String str);
Example 2: Java Scanner nextInt()
import java.util.Scanner;
class Main {
public static void main(String[] args) {
// creates a Scanner object
Scanner input = new Scanner(System.in);
System.out.println(“Enter an integer: “);
// reads an int value
int data1 = input.nextInt();
System.out.println(“Using nextInt(): ” + data1);
input.close();
}
}
Output
Enter an integer:
22
Using nextInt(): 22
Example 3: Java Scanner nextDouble()
import java.util.Scanner;
class Main {
public static void main(String[] args) {
// creates an object of Scanner
Scanner input = new Scanner(System.in);
System.out.print(“Enter Double value: “);
// reads the double value
double value = input.nextDouble();
System.out.println(“Using nextDouble(): ” + value);
input.close();
}
}
Output
Enter Double value: 33.33
Using nextDouble(): 33.33
Example 4: Java Scanner next()
import java.util.Scanner;
class Main {
public static void main(String[] args) {
// creates an object of Scanner
Scanner input = new Scanner(System.in);
System.out.print(“Enter your name: “);
// reads the entire word
String value = input.next();
System.out.println(“Using next(): ” + value);
input.close();
}
Output
Enter your name: Jonny Walker
Using next(): Jonny
Example 5: Java Scanner nextLine()
import java.util.Scanner;
class Main {
public static void main(String[] args) {
// creates an object of Scanner
Scanner input = new Scanner(System.in);
System.out.print(“Enter your name: “);
// reads the entire line
String value = input.nextLine();
System.out.println(“Using nextLine(): ” + value);
input.close();
}
}
Output
Enter your name: Jonny Walker
Using nextLine(): Jonny Walker
Conclusion-
So these are the mentioned JAva programs and compilers available online. Through this blog you will be able to code programs using String Charat ,Scanner and For loop. Also you get to know about the types of Java Compilers available online .