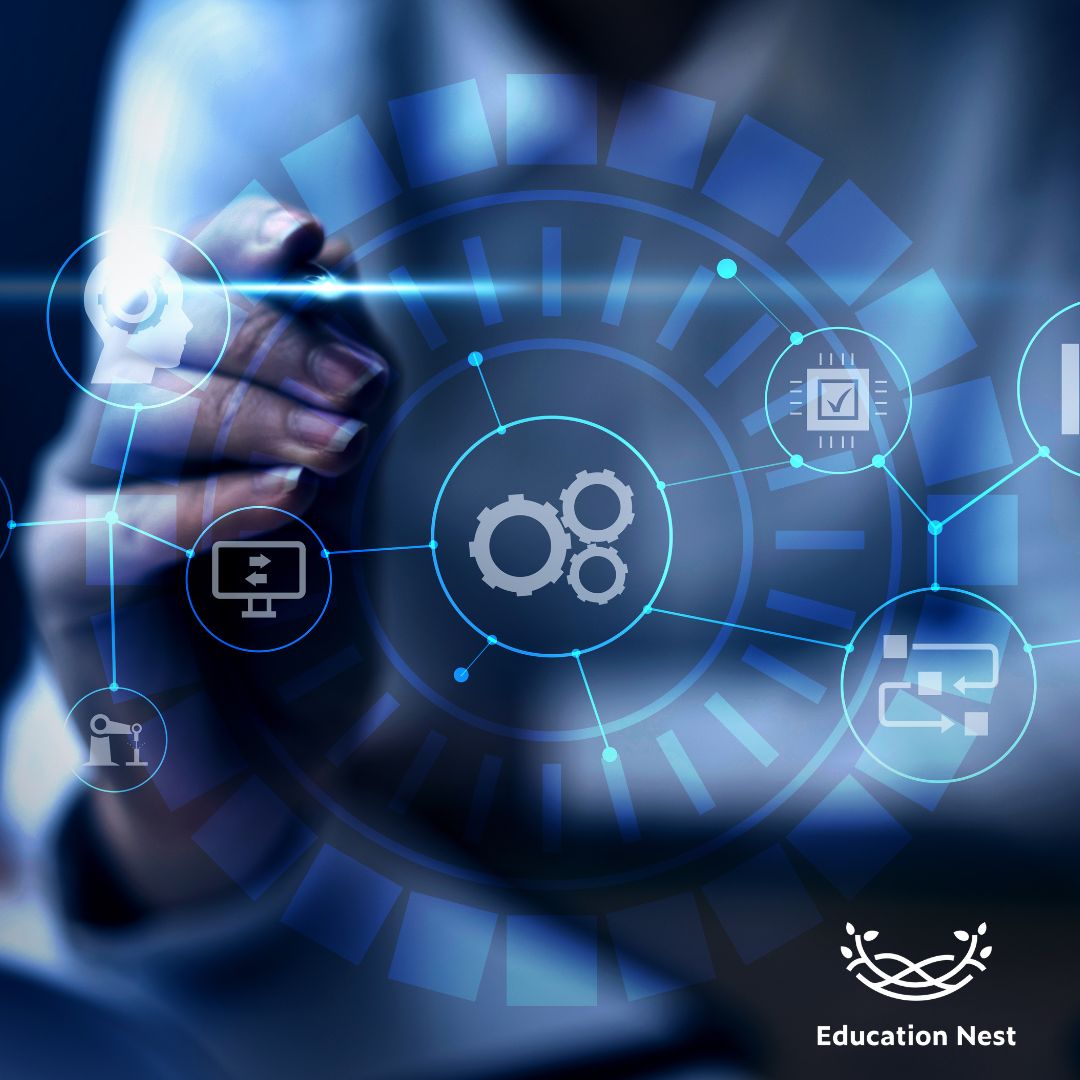
The Selenium WebDriver framework is a web-based tool for performing browser-independent testing. With this tool, web applications can be tested automatically to make sure they work as expected.
With Selenium WebDriver, you can write test scripts in any language you like. This newer version of Selenium is better than the old one because it fixes some of Selenium RC’s problems.
Tools such as Sikuli, Auto IT, etc. can be used to work around Selenium WebDriver’s inability to manage window components.
What Is Selenium Webdriver Python
Selenium WebDriver is well-known as one of the best tools for automating a website’s user interface. Python is one of the languages that can use the Selenium library to perform web UI automation testing. This is because Selenium is an open-source tool.
Since Selenium is Python-compatible, you can use it to conduct tests. Python is simple because it is less verbose than other programming languages. Python provides the APIs necessary to establish a Selenium browser connection.
The Selenium Framework: Why You Should Use It for Browser Testing Automation
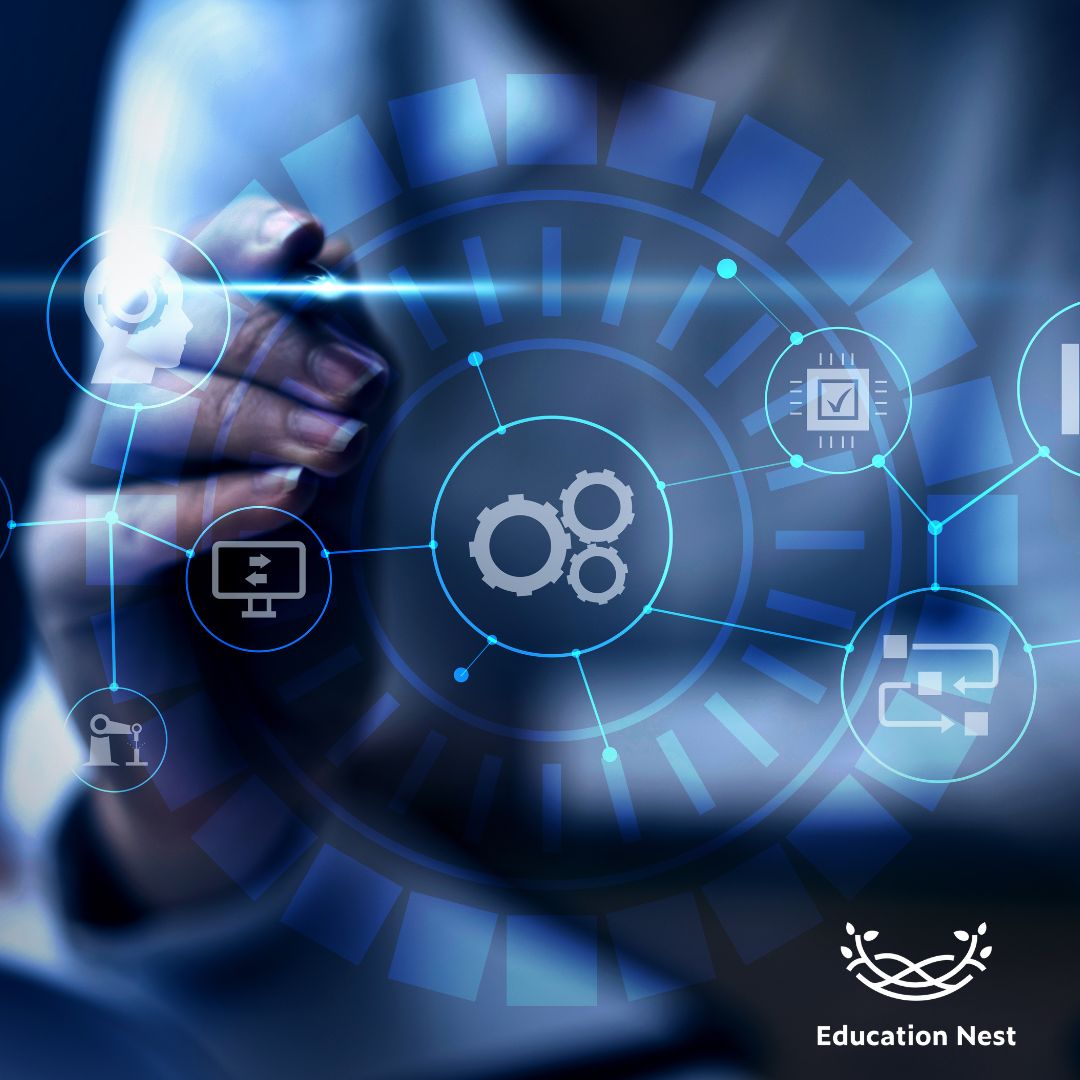
Because it is open source, downloading and using Selenium does not necessitate a license or payment of any kind. This is not the case with the vast majority of available automation software.
Using Selenium WebDriver’s ability to act like user input, you can automate key presses, mouse clicks, dragging and dropping, clicking and holding, selecting, and much more.
Selenium is a suite of tools, so you can probably find one that works for your situation and the way you like to get things done.
Advantages of Selenium
- One of the advantages is having support for multiple languages. Selenium gives the developer a lot of leeway by supporting a wide variety of languages. These include Java, JavaScript, Python, Ruby, C#, Perl,.Net, and PHP.
- Selenium supports a wide variety of browsers, OSes, and devices. These include Chrome, Firefox, Opera, Internet Explorer, Edge, and Safari (for Windows and Mac).
- To further facilitate the automation of tests, Selenium supports a wide variety of frameworks. These include Maven, Junit, and TestNG. The deployment procedure can be automated with the help of CI/CD tools like Jenkins.
- WebDriver scripts can be used in multiple browsers, increasing their reusability. This allows testers to use the same framework for a variety of different test cases.
- The Selenium community is very open and supportive. As a result, a wealth of resources is at your disposal.
- WebDriver’s user input features are very advanced. For example, you can tell it to click the browser’s back and forward buttons. This is a useful function for situations like testing electronic money transfer programs. There aren’t that many programs out there that have this function, especially free ones.
Let’s dive into Selenium Webdriver Architecture
The Selenium WebDriver API allows browsers and browser drivers to communicate with one another. The four components that make up this architecture are the Selenium Client Library, the JSON Wire Protocol, the Browser Drivers, and the Browsers themselves.
The Selenium Client Library supports a wide variety of programming languages. When the test cases are executed, the entire Selenium codebase is serialized to JSON.
The acronym JSON refers to the Java Object Notation format. It’s in charge of relaying data from the server to the user. The primary mechanism for information exchange between HTTP servers is the JSON Wire Protocol. The browser drivers can access the generated JSON through the HTTP protocol.
There is a unique driver for each browser. The browser’s driver communicates with the browser to interpret and carry out the user’s JSON commands. The browser is immediately executed upon receiving instructions from the browser driver. After that, an HTTP response is sent back to the client.
Read More: The Ultimate Guide to Web Scraping: How to Extract Data Like a Pro
Let’s analyze this snippet of code:
When using Chrome, set WebDriver’s driver to be: new ChromeDriver();
For example, driver.get (“https://www.tutorialspoint.com/index.htm”);
When this code snippet is executed, the entire piece of code will be transformed using the JSON Wire Protocol over HTTP into the form of a URL. The Chromedriver will be given the converted URL as input.
The browser’s driver connects to the server over HTTP to retrieve the request. The browser’s driver then forwards the URL to the browser over HTTP. Invoking this event will cause the browser to carry out the Selenium commands.
Now, the browser will respond with some kind of behavior if the request is a POST. If the request is a GET, the browser will generate the response. At last, it will be sent to the browser’s driver via HTTP. In turn, the UI will receive it via the JSON Wire Protocol from the browser driver.
This concludes the extensive overview of Selenium WebDriver’s underlying architecture.
Selenium WebDriver Python Example
# Importing necessary modules
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
# Setting up the driver
driver = webdriver.Chrome()
# Navigating to a website
driver.get(“https://www.google.com/”)
# Finding an element by name and sending keys
search_box = driver.find_element_by_name(“q”)
search_box.send_keys(“Selenium WebDriver”)
# Sending an Enter key
search_box.send_keys(Keys.ENTER)
# Printing the page title
print(driver.title)
# Closing the driver
driver.quit()
Explanation:
In this example, we begin by importing the required modules, which in this case are the Selenium webdriver module and the Keys module from the selenium.webdriver.common.keys package. The driver is initialized after a new instance of the Chrome class is created.
After calling get() to load the Google homepage, we use find element by name() to locate the search box element based on the value of its name attribute. Then, using the send keys() method, we simulate pressing the Enter key by sending the keys “Selenium WebDriver” to the search box.
The last step is to print the page’s title using the driver’s title property before closing it with the quit() method.
Selenium Webdriver Interview Questions
Several of the best-known browser makers have built Selenium into their products, which shows how important they think it is. This technology is used as the base for a lot of other browser automation tools, application programming interfaces, and frameworks.
Below is a list of the most commonly asked Selenium interview questions, along with the answers.
- What does selenium consist of in its various forms?
- How many automated test cases per day are considered a reasonable maximum?
- Describe the steps involved in making use of the automation framework.
- In what web browser can I use the Selenium IDE?
- What is webdriver in selenium java
- Define the Selenium Grid, please.
- Where is Selenium Grid’s central node located?
- Define the Selenium Grid node.
- What are the various selenium Web driver APIs that are available?
- What are some examples of open-source frameworks that Selenium WebDriver works with?
Conclusion
When performing automation testing on a browser, the proper browser drivers are used to communicate with the browser. This makes using a web browser more like working with an application.
Existing Selenium implementations that use Python can be migrated to work with a cloud-based Selenium Grid with only minor changes. Using Selenium Grid in the cloud is a great way to speed up your test automation. For more interactive topics, visit educationnest.com right away!