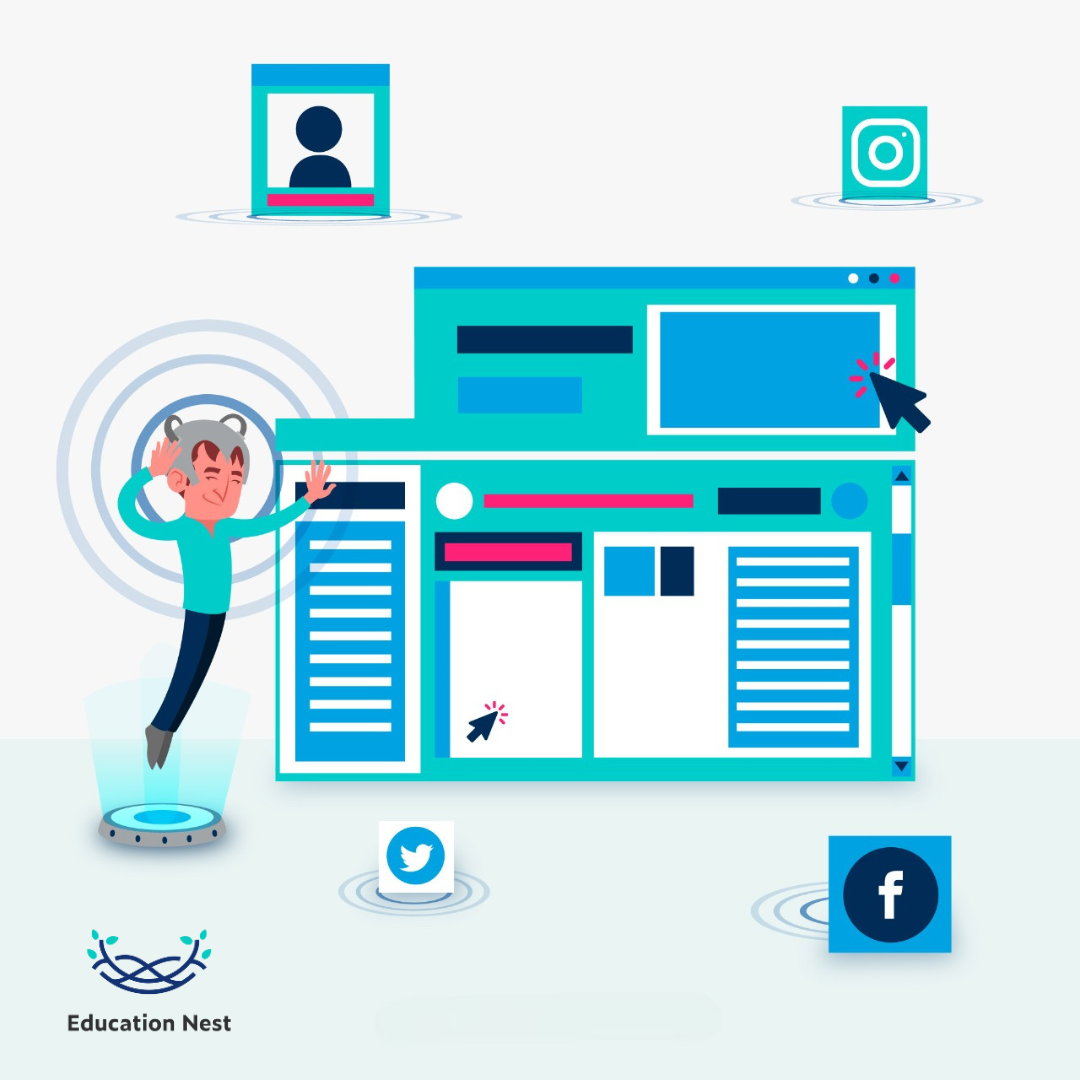
A linked list is a group of data structures linked to each other. A linked list in C is a group of items linked to each other. Each link leads to the next one in a certain way. After the array, the linked list is the second most-used way to store data.
Types of Linked List in C
In C, there are three different kinds of linked lists:
- Singly Linked List : This linked list is the most common type of linked list. Each node stores information and a link to the next node.
- Double Linked List : A double-linked list is made by adding node pointers before and after it.
- Circular List: A circular linked list contains the first and last items connected.
How Linked List in C Can Be Used?
Linked List can be used in the following ways:
- You can remember the list at all times. The node can be put anywhere in memory, and other nodes can be linked to it to make a list. This is the most suitable way to use the space you have.
- Memory size is the list size limit; you don’t have to declare it first.
- You can’t add an empty node to the linked list.
- In a singly linked list, we can store the values of either primitive types or objects .
In this article we will talk about Singly Linked List to easily understand linked lists.
One-way Chain or a Singly Linked List
A list with only one link is a set of items in an order. Depending on the application’s needs, the number of parts may change. In a single linked list, each node is made up of data and links. The node’s link part stores the next node’s address after it, while the data part stores the actual data that the node will represent.
A single–linked list or a one-way chain can only be moved in one direction. Since each node only has a pointer to the next item, we can’t go through the list backward.
Singly-Linked List Operations
Singly–linked lists can be used in many ways. This is a list of all the operations of a single list linked.
Creation of Nodes – Linked List in C
struct node
{
int data;
struct node *next;
};
struct node *head, *ptr;
ptr = (struct node *)malloc(sizeof(struct node *));
Linked List in C – Insertion
Insertions can be made into a singly linked list at different points. The insertion is put into one of the following groups depending on where the new node is being added.
1. Insertion in the Beginning
It involves placing any item at the beginning of the list. Only a few link tweaks are required to make the new node the list’s head.
2. Insertion at list’s Finish
There must be an insertion at the end of the linked list. The new node can be added as the list’s final node or as the only node. Each scenario adopts a unique line of reasoning.
3.Insertion after a Specified Node
It involves inserting data after the specified node in the linked list. The desired number of nodes must be skipped to reach the node, after which the new node will be inserted.
You Must Know: Get the Most Out of Java Control Statements with These Expert Tips
Linked List in C – Traversing and deletion
There are various positions at which a node can be eliminated from a singly linked list. The procedure is divided into the following categories according to the node’s position being eliminated.
1.Omission in the Beginning
This means getting rid of the first item on the list. This is the simplest thing you can do. Few changes are made to the node pointers.
2.Taking off the Last Two items on the List
It means taking the last node off the list. The list could be empty or full of everything. For each of the different situations, another reason is used.
3. Deletions after a Particular Node
It means removing the node from the list that comes after the node that was given. The desired number of nodes must be skipped to get to the node that will be deleted. This needs to go through the list.
4. Traversing
To do something specific to each node, like print the data section of each node in the list, we go through the list at least once, stopping at each node.
5. Searching
During a search, every item in the list is compared to the entity requested. If the element can be found anywhere, its location is returned. Null is returned if it can’t be found at any of those places.
C Program to Create a Linked List
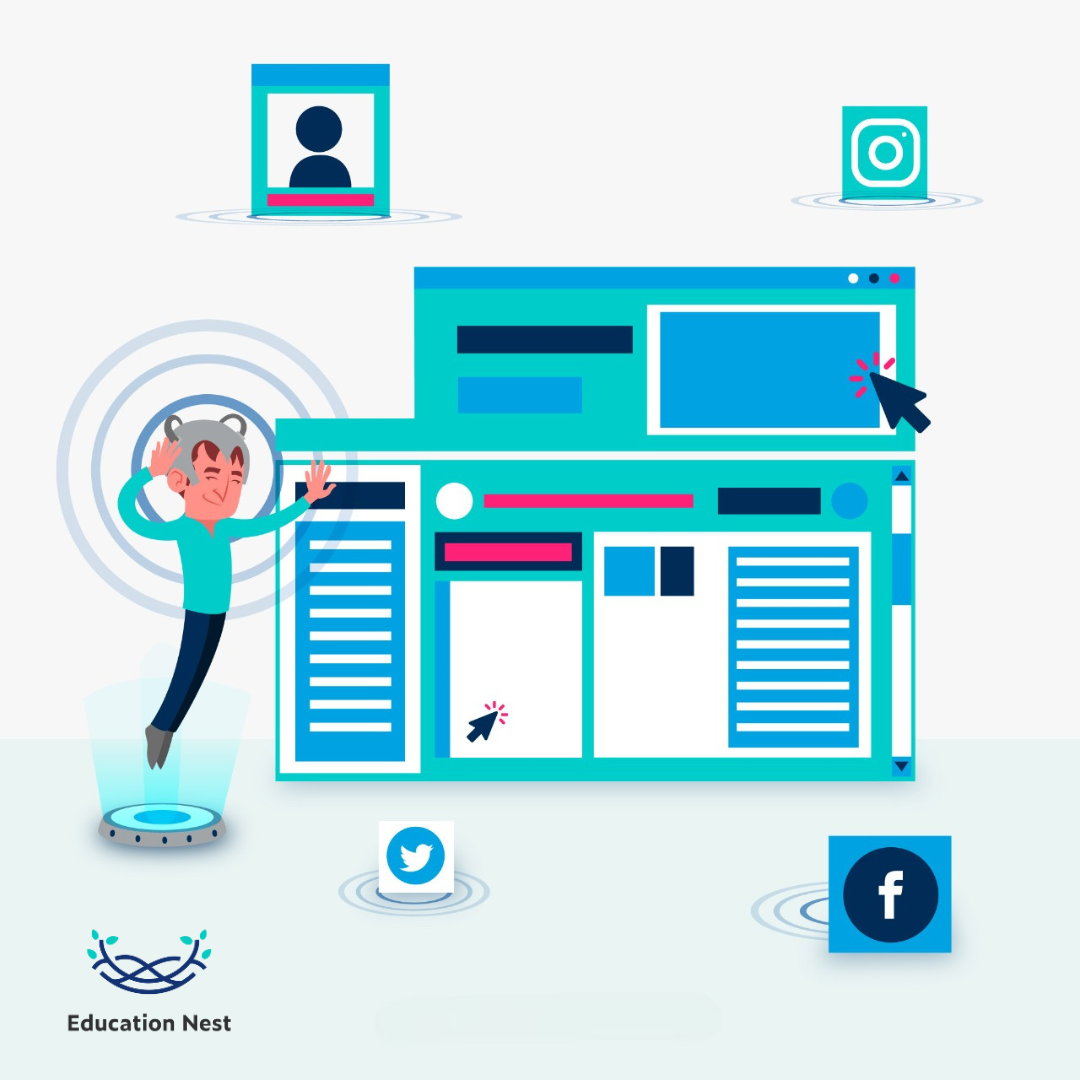
This program is supposed to make a linked list and show all the items on that list.
/*
* C program to create a linked list and display its elements
*/
#include <stdio.h>
#include <malloc.h>
#include <stdlib.h>
void main()
{
struct node
{
int num;
struct node *ptr;
};
typedef struct node NODE;
NODE *head, *first, *temp = 0;
int count = 0;
int choice = 1;
first = 0;
while (choice)
{
head = (NODE *)malloc(sizeof(NODE));
printf(“Enter the data item\n”);
scanf(“%d”, &head-> num);
if (first != 0)
{
temp->ptr = head;
temp = head;
}
else
{
first = temp = head;
}
fflush(stdin);
printf(“Do you want to continue(Type 0 or 1)?\n”);
scanf(“%d”, &choice);
}
temp->ptr = 0;
/* reset temp to the beginning */
temp = first;
printf(“\n status of the linked list is\n”);
while (temp != 0)
{
printf(“%d=>”, temp->num);
count++;
temp = temp -> ptr;
}
printf(“NULL\n”);
printf(“No. of nodes in the list = %d\n”, count);
}
$ cc pgm98.c
$ a.out
Enter the data item
5
Do you want to continue(Type 0 or 1)?
0
status of the linked list is
5=>NULL
No. of nodes in the list = 1
$ a.out
Enter the data item
5
Do you want to continue(Type 0 or 1)?
1
Enter the data item
9
Do you want to continue(Type 0 or 1)?
1
Enter the data item
3
Do you want to continue(Type 0 or 1)?
0
status of the linked list is
5=>9=>3=>NULL
No. of nodes in the list = 3
Conclusion
Therefore, Linked Lists can be used to add and remove items efficiently, and they are often used to make other data structures, like stacks and queues. But because each element must be visited in order, it can take longer to get to a specific component of a linked list than in an array or another data structure with direct access.