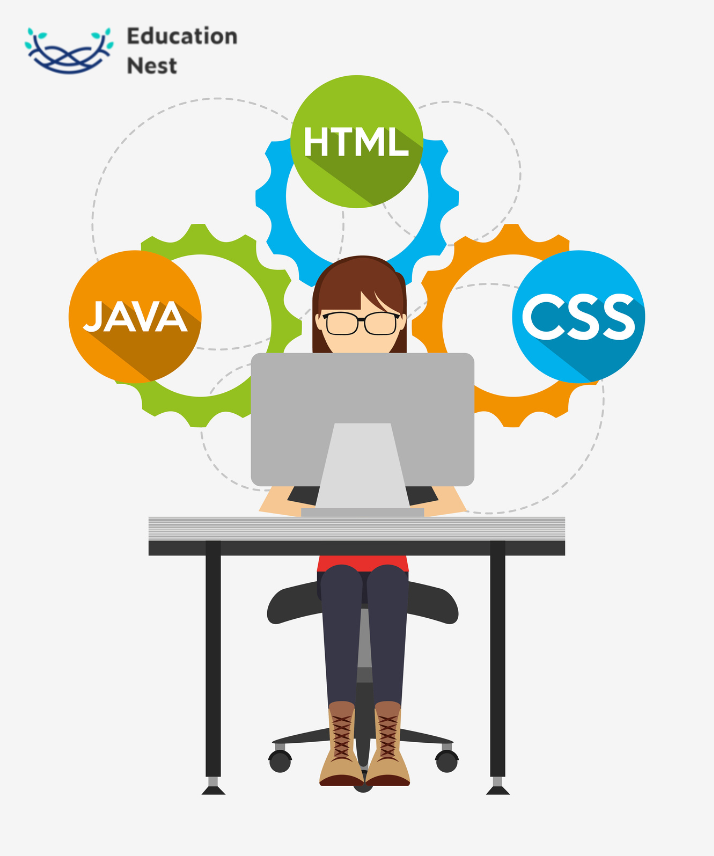
Hi! Today we’ll consider a critical topic that concerns our objects. Without exaggeration, you’ll use this topic in real life every day! We’re talking about Java Constructors and types of java constructors with the help of examples. This may be your first time hearing this term, but you’ve already used constructors. You didn’t realize it. We will convince ourselves of this later.
What is a constructor in Java?
Java’s special function Object() [native code] is always called upon when a new object is created. Its invocation guarantees that the object’s necessary fields are initialized correctly and establishes the object’s initial state. To restate this in terms of programming, “Object()” (native code) is the process of creating and modifying an object’s properties.
There is no return value from Java’s constructors, which share the same name as the classes they are associated with. In order to accomplish this, they often give objects default settings and initial values. When creating an object in Java, using the built-in Object() function ensures that all required fields are set to null and other rules are followed.
What are the types of constructors in Java?
There are mainly two types of constructors in Java: default constructors and parameterized constructors.
Default Constructors: A default constructor is a constructor that takes no arguments. It is also called non parameterized constructor in Java. It is created automatically by the Java compiler if no constructor is explicitly defined for a class. The purpose of a default constructor is to initialize all instance variables to their default values. For example, a default constructor for a class with two integer fields would look like this:
public class MyClass {
int x;
int y;
// default constructor
public MyClass() {
x = 0;
y = 0;
}
}
In this example, the default constructor initializes the values of x and y to zero. If a default constructor is not defined explicitly in a class, the Java compiler will create one automatically.
- Parameterized Constructors:
Parameterized constructors are those that can take more than just the default values as arguments. Its purpose is to limit how the object is built based on parameters that have already been set and to start it up with settings that have already been set. For the same class with two integer fields, a parameterized version of the Object() [native code] function could look like this:
public class MyClass {
int x;
int y;
// parameterized constructor
public MyClass(int x, int y) {
this.x = x;
this.y = y;
}
}
Example: The parameterized function Object() { [native code] } in this case accepts two integer arguments, x and y, and sets their values to those passed in. The object’s instance variables are referred to with this keyword.
To ensure that certain criteria are met during object construction , parameterized constructors can be used . Take the case of a class representing a bank account, for instance:
Class of the general public Having a bank account
String accountNumber;
String accountholder name;
double balance;
public BankAccount(String accountNumber, String accountHolderName) {
if (accountNumber == null || accountNumber.isEmpty()) {
throw new IllegalArgumentException(“Account number cannot be empty”);
}
if (accountHolderName == null || account.HolderName.isEmpty()) {
throw new Illegal Argument Exception(“Account holder name can’t be empty”);
}
this.accountNumber = accountNumber;
this.accountHolderName = accountHolderName;
this.balance = 0;
}
}
In this example, the parameterized constructor takes two arguments accountNumber and accountHolderName. It also enforces the rule that the account number and holder name cannot be empty or null. If either of these conditions is violated, an exception is thrown.
- Constructor Overloading:
In Java, constructors can be overloaded. Meaning can define multiple constructors for a single class with different parameter lists. This allows for more flexibility in object creation and initialization. For example, consider a type that represents a rectangle:
public class Rectangle {
int length;
int width;
// parameterized constructor
public Rectangle(int length, int width) {
this.length = length;
this.width = width;
}
// default constructor
public Rectangle() {
this.length = 0;
this.width = 0;
}
}
In this case, the Rectangle class has both a default and a parameterized function, Object() [native code]. The initial values for height and width are determined by the values passed into the parameterized function Object() [native code] as integers.
You Must Like: Mastering Inheritance in Java: A Complete Guide for Beginners
What do you mean by copy constructor in Java?
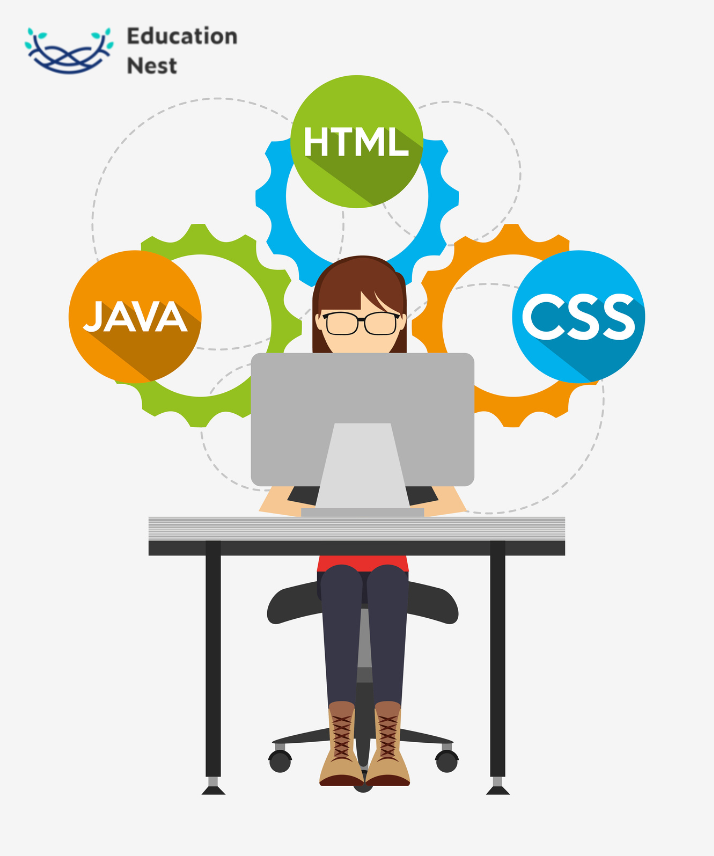
In Java, programmers use the Object () copy constructor to make a new object. It is possible to make a new object that is the same as a normal one in every way, including where it is stored in memory. Use the copy constructor method Object () when you need a new instance of an object that is the same as an existing one but don’t want to change the existing one.
In Java, a copy function is called Object (). Native code for Object () must be a function that takes an object of the same class as the code. Then, the state of the object passed as an argument is copied into the object that was just made. The following would be a good example:
public class Person {
private String name;
private int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
// Copy constructor
public Person(Person other person) {
this.name = otherPerson.name;
this.age = otherPerson.age;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
}
In this example , the Person class has a copy constructor that takes an instance of the same type as an argument . The new Person object is created by copying the name and age fields of the argument object into the new thing .
Here’s an example of how to use the copy constructor to create a new object:
Person john = new Person(“John”, 30 );
Person johnCopy = new Person(john);
In this example, a new Person object john is created with the name “John” and age 30. Then, a new Person object john Copy is created using the copy constructor, which creates a new object identical to john .
It’s important to remember that the copy function Object() { [native code] } only makes a shallow copy of the object. This means that if the thing has any reference types (like arrays or other objects), only the references to those objects are copied , not the objects themselves . If you want to make a deep copy of an object , you must write your code .
Conclusion:
In conclusion, Java’s constructors are important for object-oriented programming and are widely used in software development today. Java constructors are used to set up the state of an object and make sure that all of its fields are set up right. In Java, constructors can be either “default” or “parameterized.” You can set up an object in a different way with each of these. Also, function Object() [native code] overloading and copy constructors give you more ways to make and set up things. Overall, constructors are an important part of object-oriented programming and are needed to make software applications that are reliable, robust, and efficient in the modern technological age.