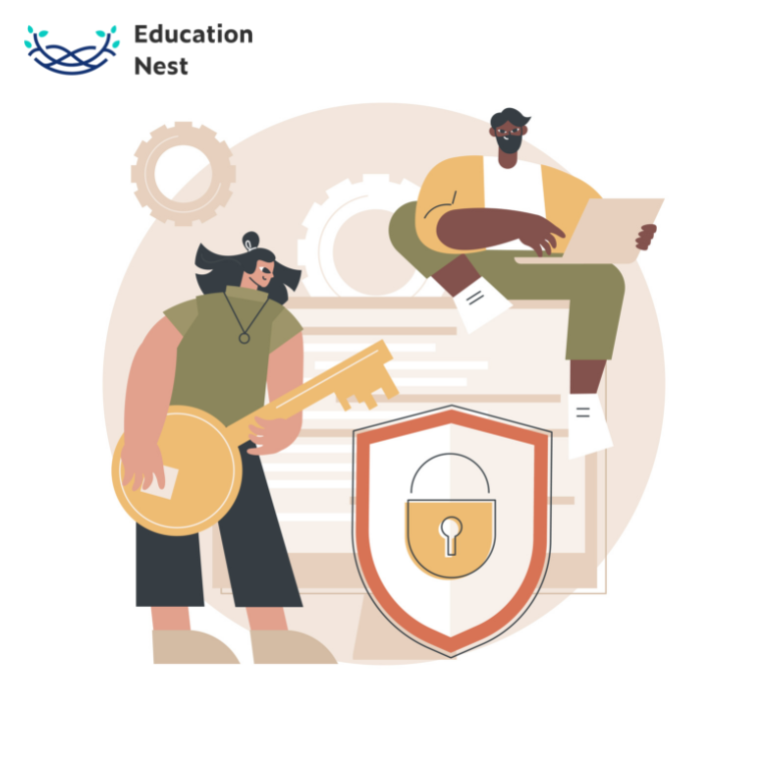
Inheritance in Java is a fundamental concept in modern object-oriented programming and is largely used today to develop complex and sophisticated applications. It can be defined as the process where one class acquires the properties of another . With the use of inheritance, the information is manageable in a hierarchical order. In this blog post, we will discuss the inheritance of Java with the help of examples and the types of inheritance in Java.
What Is Inheritance in Java?
Inheritance in Java is a mechanism that allows a class to inherit properties of another class. In simpler terms, it is a process by which one type acquires the property of another class.
The class inherited from is called the superclass or parent class, and the class inherited from the superclass is called the subclass or child class. The subclass can use all of the superclass’s public fields and methods and add new methods and fields or change the ones already there.
In object-oriented programming , inheritance is an important idea because making it easier to reuse code and helps make a class structure with a hierarchy. It lets us define a general class and then create subclasses that inherit from it and add more specific behaviors.
Also, inheritance is vital to making user interfaces and other graphical parts in Java. Using inheritance, developers can create components that can be used more than once and are easy to change and add to fit the application’s needs.
Overall, inheritance in Java is still an essential part of modern software development. It is used to make module , reusable, and expandable software architectures that can be changed to meet the changing needs of users.
Inheritance in java example:
Let’s say we have a superclass called “Vehicle” that has fields and methods like “make,” “model”, “year,” and “getDetails()”. Now, we want to make a subclass called “Car” that inherits from the “Vehicle” class but also has its fields and methods.
Here’s an example code for implementing inheritance in Java:
public class Vehicle {
private String make;
private String model;
private int year;
public Vehicle(String make, String model, int year) {
this.make = make;
this.model = model;
this.year = year;
}
public String getMake() {
return make;
}
public String getModel() {
return model;
}
public int getYear() {
return year;
}
public void getDetails() {
System.out.println(“Make: ” + make + “, Model: ” + model + “, Year: ” + year);
}
}
public class Car extends Vehicle {
private int numDoors;
public Car(String make, String model, int year, int numDoors) {
super(make, model, year);
this.numDoors = numDoors;
}
public int getNumDoors() {
return numDoors;
}
public void setNumDoors(int numDoors) {
this.numDoors = numDoors;
}
@Override
public void getDetails() {
super.getDetails();
System.out.println(“Number of doors: ” + numDoors);
}
}
Types Of Inheritance in Java
There are five types of inheritance in Java, which are :
- Single Inheritance:
In a single-inheritance structure, a subclass only extends a single superclass. That is to say, there can be exactly one parent class to a subclass. In Java, this is the most frequent method of inheritance, and it’s used to create a hierarchical structure out of classes.
- Multilevel Inheritance:
In multilevel inheritance, a subclass extends a superclass that is itself a subclass. Hence, each subclass inherits properties and methods from its parent class. With multiple-level inheritance, classes can gain functionality from a wider range of parent classes, allowing for greater specialisation.
- Hierarchical Inheritance:
Several different subclasses can inherit from the same superclass in a hierarchical inheritance structure. Classes might be both parents and children. Child classes can have their own fields and methods, but they must all adhere to the same set of shared parent class properties.
- Multiple Inheritance:
It is possible for a subclass to inherit properties from many superclasses in a system that allows for multiple inheritances. That is to say, a subclass may have several superclasses. Even though Java doesn’t provide this form of inheritance, it’s possible to mimic it with interfaces. To perform a task, a class may implement an interface, which is a collection of abstract methods. It is possible for a class to provide the appearance of having multiple parents by implementing multiple interfaces.
- Hybrid Inheritance:
Hybrid inheritance is when two or more types of inheritance are mixed. With multilevel inheritance, for example, a class can inherit from two different classes. With multiple inheritance, the class can also inherit from an interface.
It’s important to know that Java doesn’t support multiple inheritance, but interfaces can be used to make it look like it does. Also, inheritance can be a powerful tool, but it should be used carefully to keep code easy to read and avoid making class hierarchies hard to understand.
You Must Like: Data Analytics Made Easy: 10 Must-Have Tools for Every Business
Types Of Inheritance in Java with Example
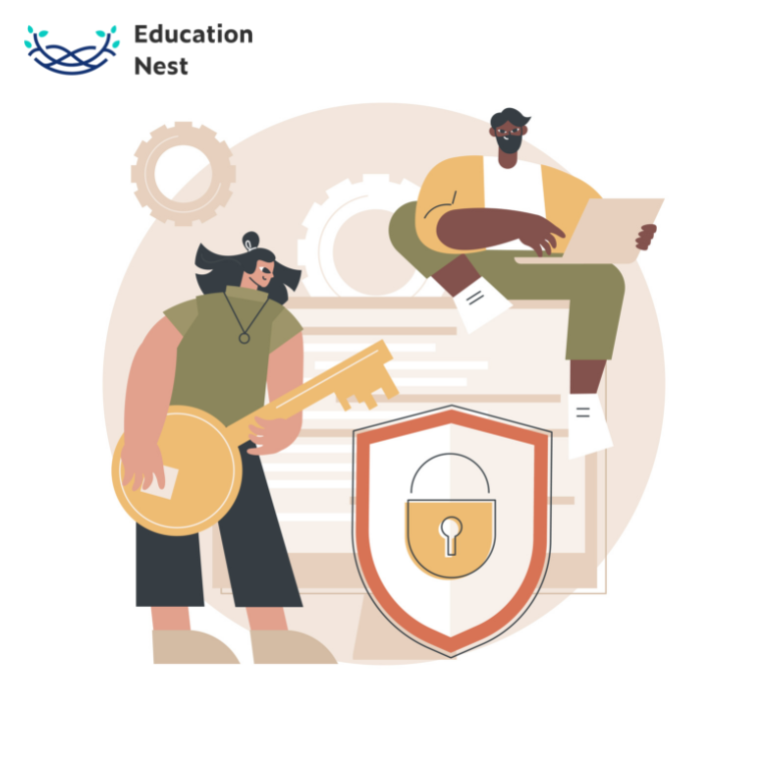
- Single Inheritance Example:
class Animal {
void eat() {
System.out.println(“Animal is eating”);
}
}
class Dog extends Animal {
void bark() {
System.out.println(“Dog is barking”);
}
}
class Main {
public static void main(String args[]) {
Dog d = new Dog();
d.eat(); // calling method of parent class
d.bark(); // calling method of child class
}
}
- Hierarchical Inheritance Example:
class Animal {
void eat() {
System.out.println(“Animal is eating”);
}
}
class Cat extends Animal {
void meow() {
System.out.println(“Cat is meowing”);
}
}
class Dog extends Animal {
void bark() {
System.out.println(“Dog is barking”);
}
}
class Main {
public static void main(String args[]) {
Cat c = new Cat();
c.eat(); // calling method of parent class
c.meow(); // calling method of child class
Dog d = new Dog();
d.eat(); // calling method of parent class
d.bark(); // calling method of child class
}
}
- Interface (Multiple Inheritance) Example:
interface Animal {
void eat();
}
interface Mammal {
void sleep();
}
class Dog implements Animal, Mammal {
public void eat() {
System.out.println(“Dog is eating”);
}
public void sleep() {
System.out.println(“Dog is sleeping”);
}
}
class Main {
public static void main(String args[]) {
Conclusion
The inheritance system in Java lets one class use the functions and features of another. It lets programmers make flexible, modular software that can use what it already has and grow as needed. In Java, the “extends” keyword is used to say which superclass a subclass inherits from. By inheriting its parent class’s properties and methods, a subclass can avoid having to define them again. By making systems more modular, inheritance makes it easier to manage and keep track of large code bases. It also lets objects of different types be handled as if they were of the same type. This is called polymorphism. In Java, inheritance is a useful tool that can save developers time and give them more freedom.