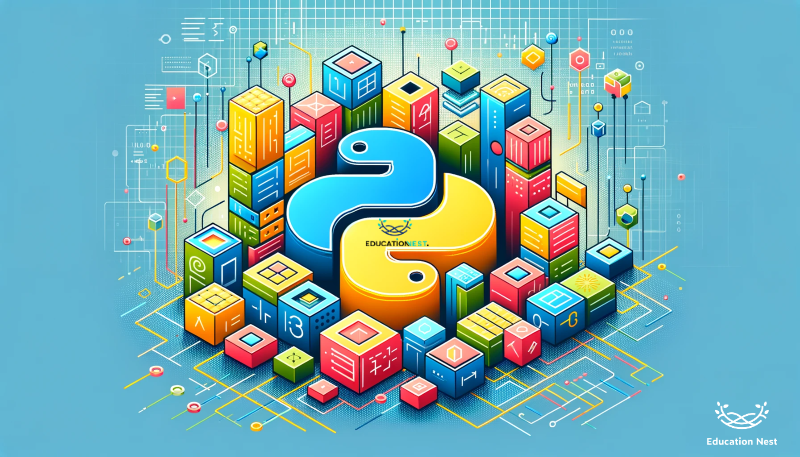
In the ever-evolving world of programming, Python remains a constant favorite, thanks in part to its simplicity and versatility. A fundamental aspect of mastering Python is understanding its python data types and python data structures. Whether you’re a beginner or looking to refresh your knowledge, this cheat sheet will guide you through the data types in Python with examples. Python’s ease of use is partly because of how it handles different python numeric data types and python core data types, making it a go-to language for many developers. This guide will delve into the question of how many data types in python there are and how they can be effectively utilized in your coding journey.
What are Python Data Types?
In Python, data types are foundational concepts that dictate the kind of data a variable can hold. Think of them as labels that tell Python how it should handle different kinds of information. Data types are integral in helping Python understand what sort of operations can be performed with the data. For example, you can add and subtract integers or concatenate strings, but you can’t mix these operations across different types (like adding a string to an integer).
Understanding the Basics
At its core, Python classifies data into various types, which include:
- Numeric Types: For representing numbers. These include integers (whole numbers), floats (numbers with a decimal point), and complex numbers (numbers with a real and imaginary part).
- Sequences: These are ordered collections of items. Examples include lists, tuples, and ranges.
- Text Type: Specifically for textual data, Python uses the string data type.
- Mapping Type: Python uses dictionaries as its primary mapping type, which consists of key-value pairs.
- Set Types: Sets and frozensets are used for unordered collections of unique items.
- Boolean Type: This represents two values: True or False, and is commonly used in conditional and control statements.
By understanding these data types, programmers can effectively store and manipulate data in their Python programs. Each data type comes with its own set of operations and methods, making Python a flexible and powerful tool for data manipulation and analysis. Whether you’re storing a simple number, a collection of data, or textual information, knowing the appropriate Python data type to use is key to writing efficient and error-free code.
Data Types in Python with Examples
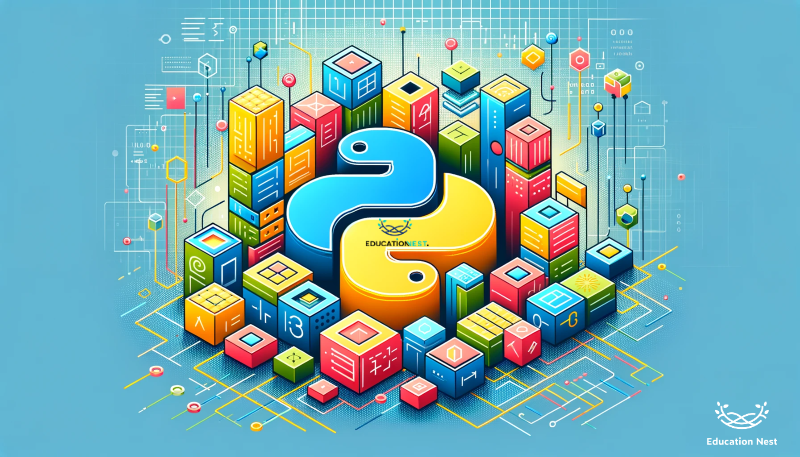
Under the umbrella of Data Types in Python with Examples, we delve into how each data type functions in real-world scenarios. Python’s versatility in data handling is best understood through these practical examples, which illustrate the different ways data can be represented and manipulated in Python.
Numeric Types
- Integers (int): Used for whole numbers.
Example: days_in_week = 7 represents the number of days in a week.
- Floating Point Numbers (float): Used for numbers with decimal points.
Example: average_temperature = 23.5 could represent the average temperature in Celsius.
- Complex Numbers (complex): Used in advanced mathematical computations, containing a real and an imaginary part.
Example: complex_number = 3 + 4j represents a complex number with real part 3 and imaginary part 4.
Sequence Types
- Strings (str): Used for textual data, enclosed in either single or double quotes.
Example: greeting = “Hello, World!” is a string containing a greeting message.
- Lists: Ordered, mutable (changeable) collections of items.
Example: fruits = [“apple”, “banana”, “cherry”] is a list containing three fruit names.
- Tuples (tuple): Ordered, immutable (unchangeable) collections of items.
Example: coordinates = (10.0, 20.0) could represent the x and y coordinates on a graph.
Boolean Type
Boolean (bool): Represents one of two values: True or False. Often used in conditions and logical operations.
Example: is_raining = True could be used to represent whether it is raining or not.
Set Types
- Set: Unordered collections of unique items. Useful for operations like unions and intersections.
Example: unique_numbers = {1, 2, 3, 4, 5} is a set of numbers without duplicates.
- Frozenset: An immutable version of a set.
Example: frozen_set = frozenset([“apple”, “banana”, “cherry”]) represents an immutable set of fruits.
Mapping Type
Dictionary (dict): Key-value pairs. Each key is unique and is used to store and retrieve values.
Example: person = {“name”: “Alice”, “age”: 25} is a dictionary storing a person’s name and age.
The Practicality of Python Data Types
These examples illustrate the flexibility and practicality of Python data types in representing different kinds of data. From simple numbers and text to more complex structures like lists and dictionaries, Python’s data types are equipped to handle a wide range of data management and processing needs. Understanding these data types and their applications is a fundamental step in becoming proficient in Python programming.
Also Read:
Mastering Data Structures in Python: Your Complete Guide
Importance of Python Data Types
Python data types are much more than mere classifications of data; they are pivotal in determining how data is stored, manipulated, and conveyed within a Python program. Their significance lies in the fundamental role they play in the construction of efficient, reliable, and readable code.
Efficient Data Handling
Each data type in Python has been optimized for specific kinds of data and operations. For instance, integers and floats are tailored for arithmetic computations, while strings are optimized for text manipulation. Using the correct data type means Python can process and handle the data more efficiently, both in terms of speed and memory usage.
Code Reliability and Safety
Using the appropriate data type helps prevent errors in your code. For example, trying to perform a mathematical operation on a string would result in an error, alerting you to a potential issue in your logic or data handling. This type-checking aspect of Python makes your code more robust and less prone to bugs.
Enhancing Readability and Maintainability
Understanding and using Python data types correctly also contribute to the readability and maintainability of the code. When a variable is assigned a specific data type, it becomes clearer to other programmers (or even to you at a later date) what the intention of the variable is and how it should be used. This clarity is crucial in collaborative environments and for long-term maintenance of the code.
In conclusion, the importance of Python data types extends beyond simple categorization. They are central to writing effective, efficient, and error-free Python code. Understanding and utilizing these data types correctly is a key skill for any Python programmer.
Conclusion
Python’s data types and structures are fundamental to its programming prowess. This cheat sheet serves as a guide to understanding and utilizing these types effectively. Whether you’re a beginner or brushing up on your skills, this guide is designed to provide a clear and comprehensive understanding of Python’s data handling capabilities. Happy coding!