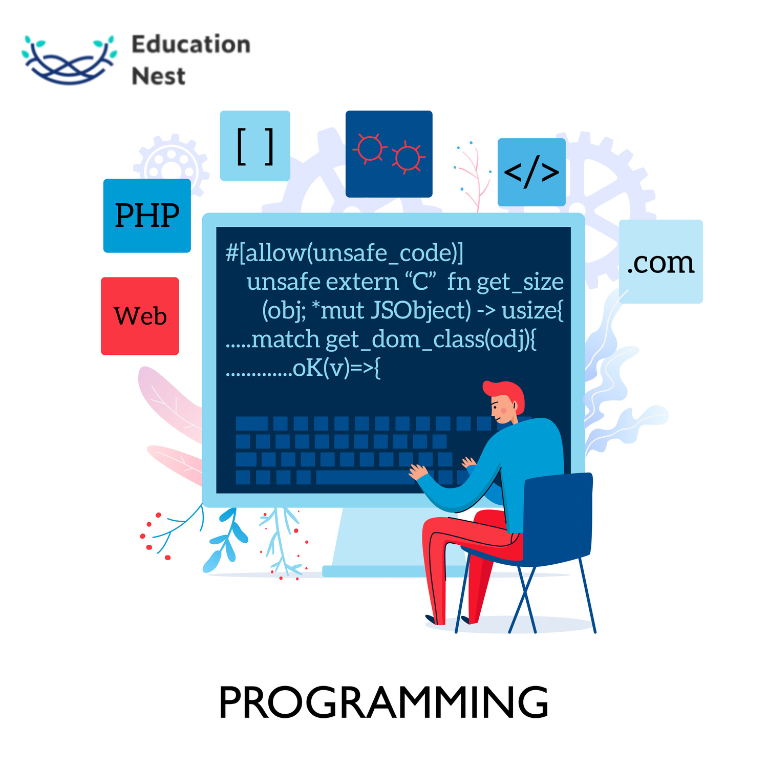
Palindromes are fascinating and fun to play with. They are words, phrases, or numbers that read the same backward as forwards. For example, “racecar,” “level,” and “madam” are all palindromes. Creating a program that can recognize palindromes is a great way to improve your coding skills, as well as impress your friends and colleagues. In this blog post, we will unveil the mystery behind palindrome strings and guide you through the process of writing a program that can identify them. Whether you are a beginner or an experienced programmer, this step-by-step guide will help you build your own palindrome string program from scratch. So, let’s get started and discover the magic of palindromes!
It’s interesting to talk about computer science topics that can be seen in more than one way. A palindrome is a group of letters or words that mean the same thing, whether they are read forward or backward. No matter how the word is spelled, it means the same thing. “Palindrome” is the word for it. This article will look at a few different ways to make a Python program that comes up with palindromes. We will talk about how to use functions, while loops, and curls, among other things. We’ll also look at a real-life example to show how vital palindromes are in coding.
What Is a Palindrome Program in Python?
The palindrome can be a number, phrase, sequence, or word of characters that reads the same backward and forward. Here is an example of a Palindrome Program In Python:
def is_palindrome(word):
# Remove all spaces and convert to lowercase
word = word.replace(” “, “”).lower()
# Check if the word is the same when reversed
return word == word[::-1]
# Example usage
print(is_palindrome(“rotator”)) # Output: True
print(is_palindrome(“sentence”)) # Output: False
With this code, if the word is a palindrome function, it will show “True” if the given string is a palindrome. Otherwise, it will show False. The following describes how it works:
First, we use the replace method to eliminate any spaces in the input string. Then, we use the lower function to change the string to lowercase. This ensures the process will work correctly for phrases with both small and capital letters.
Then, we use the slicing syntax [::1] to see if the result is the same as the exact string backward. The function will return True if the input is a palindrome and the two strings match up. If the information is not a palindrome, the process will return False.
Palindrome String Program in Python Using for Loop
A python program is that which checks if the given string is a palindrome using for loop:
def is_palindrome(s):
# Convert the input string to lowercase and remove non-alphanumeric characters
s = ”.join(e for e in s if e.isalnum()).lower()
# to see if the string is equal to it’s reverse using a for loop
for i in range(len(s)):
if s[i] != s[-i-1]:
return False
return True
# Example usage
word = input(“Enter a word: “)
if is_palindrome(word):
print(word, “is a palindrome”)
else:
print(word, “is not a palindrome”)
This program, ”is_palindrome” function works as follows:
- The first stage is cleaning the input string by removing all non-alphanumeric characters and converting them to lowercase, similar to the example.
- Then function uses the loop to see if the string is the exact same as its reverse. Then the loop runs from the first to the central character from the end of the string, checking each character against the same one at the end. If any of these characters are wrong, the method will return false to show that the string is not a palindrome. The process will return True if the given string is a palindrome.
This method is slightly faster than slicing the whole string and comparing it to its opposite because it only needs to reach characters up to the middle.
How To Write a Program in C To Check If a Given String Is a Palindrome or Not
To determine whether a string is a palindrome, we need to compare the first and last characters. To begin, we can write a function to check if a given string is a palindrome and return true if it is or false otherwise. To put it in practice, consider this example: #include <stdio.h>
#include <string.h>
int isPalindrome(char str[]) {
int i = 0;
int j = strlen(str) – 1;
while (i < j) {
if (str[i] != str[j])
return 0; // not a palindrome
i++;
j–;
}
return 1; // is a palindrome
}
int main() {
char str[100];
printf(“Enter a string: “);
fgets(str, 100, stdin);
if (isPalindrome(str)) {
printf(“%s is a palindrome\n”, str);
} else {
printf(“%s is not a palindrome\n”, str);
}
return 0;
}
Suppose we go through this program one by one. First, we include the most important header files, such as stdio. h for input/output and string. h for working with strings. Next, we make a function called palindrome that takes a string as an input.
In the function’sfunction’s body, we give the variables I and j the values 0 and 255, which are the start and end of the string, respectively. Then, the characters at I and j are checked to see if they are the same using a while loop. If the two values are not the same, we treat the string as if it weren’t a palindrome and return 0. If they are the same, I will move forward one character, J will move backward one character, and the loop will keep going.
When the while loop is made, we return one if the string is a palindrome.
In the primary method, we make a 100-character array called str to store what the user types. Next, we ask the user for a string of up to 100 characters using the fgets method.
Then, we send the input string to the palindrome function to see if it is a palindrome. If the value is 1, the string is shown as a palindrome. If the string isn’t a palindrome, we’ll show that.
All done! If you use software to figure out if a string is a palindrome, it should always give you the correct answer. Please remember that this implementation cares about the case and looks at spaces and punctuation when deciding whether a string is a palindrome. The palindrome function is easy to change so that it doesn’tdoesn’t look at certain characters.
How to Write a Program to Check Whether a String Is Palindrome or Not in Python
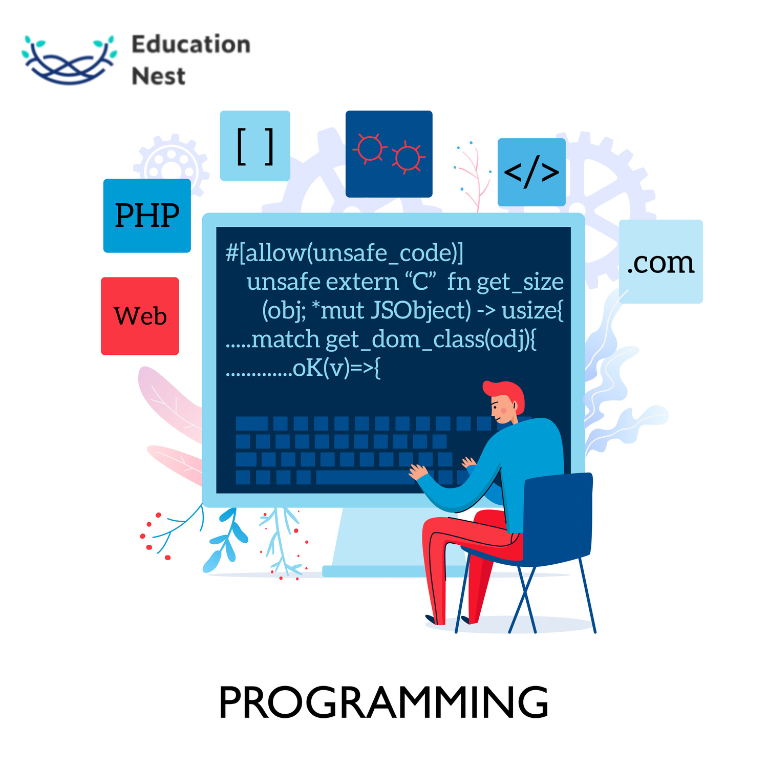
To determine if a string is a palindrome, must take out all non-alphanumeric letters, and the string must be changed to lowercase. This step ensures that the program treats the string the same way, no matter what case it is in or how many characters it has. We can get there by combining the filter function, which removes everything except letters and numbers, with the lower approach, which makes everything lowercase.
Here’sHere’s an example of a function that does this:
def clean_string(s):
# Remove all non-alphanumeric characters and convert to lowercase
return ”.join(filter(str.isalnum, s)).lower()
To clean up a string, the clean string function takes it as input, changes all the letters to lowercase, and removes any symbols that aren’taren’t letters or numbers. You can do more with the string by using this function.
The next step in determining if a string is a palindrome is to compare the original to its mirror image. We can do this easily by slicing the string. A string is a palindrome if it still means the same thing when read backward.
Here’s an example of a function that does this:
def is_palindrome(s):
# Clean the input string
s = clean_string(s)
# Compare the reversed string with the original string
return s == s[::-1]
The palindrome function takes a string as input, runs it through the clean string to eliminate unwanted characters, and then uses slicing to compare the inverted string to the original string. With the [::-1] notation, you can make the string’sstring’s mirror image.
With the right functions in place, it’s easy to write software that asks the user for a string and then tells the user if the string is a palindrome.
def main():
s = input(“Enter a string: “)
if is_palindrome(s):
print(“The string is a palindrome”)
Else:
print(“The string is not a palindrome”)
if __name__ == ‘__main__’:
main()
The input string s is run through the clean string function to eliminate any extra characters. The output string is then inverted, and the slicing function is used to compare it to the input string to see if it is a palindrome. You can turn the string around using the notation [::-1].
With the correct methods, we can quickly code a tool that asks the user for a string and then tell them if it is a palindrome.
You Must Like: 10 Amazing Pattern Programs in Java Every Developer Should Know
Example of Palindrome String:
We will discuss exploring palindrome string examples and checking if the string is a palindrome using different programming languages.
One of the most common examples of palindrome string is the “racecar” word. The word remains the same if you read this word right to left or left to right. This kind of word is called palindrome string.
Another word that can read both forwards and backward is ” level.” It looks the same whether you read this word from top to bottom or bottom to top. Another word with a string of palindromes is “deified.” This word means the same thing whether you read it from left to right or from right to left. This makes it a palindrome.
The word “rotator” can be read both ways. This word can be read from left to right or right to left without changing what it means. “Madam” is another word with a string of palindromes. The term “civic” is an example of a string of words that can be read in both directions.
The word “rotator” can be read both ways. This word can be read from left to right or right to left without changing what it means. “Madam” is another word with a string of palindromes. The term “civic” is an example of a string of words that can be read in both directions.
Now that we’ve seen what palindrome strings look like, it’s time to look at how different programming languages can tell if a string is a palindrome.
Python is a popular computer language that can use in many different ways. The code below shows how to use Python to find out if a string is a palindrome or not:
def is_palindrome(s):
return s == s[::-1]
s = “racecar”
if is_palindrome(s):
print(“The string is a palindrome”)
else:
print(“The string is not a palindrome”)
Here, we show how to make a function that checks if a string is a palindrome and returns True or False, depending on what it finds. The name of the function is a palindrome. The function’s job is to compare the original string to its mirror image. This string is a palindrome if it reads the same from left to right and right to left.
To move on, let’s make a new string variable called “s” and give it the value ” racecar.” The palindrome function is then given the string s as an argument. The message “The string is a palindrome” will be shown if the function works. If the function returns false, the message “The string is not a palindrome” is displayed.
Java is another widely used programming language that can use in many different ways. Here’sHere’s an example of how to tell if a string is a palindrome using Java:
public static boolean isPalindrome(String s) {
int length = s.length();
for (int i = 0; i < length / 2; i++) {
if (s.charAt(i) != s.charAt(length – i – 1)) {
return false;
}
}
return true;
}
String s = “racecar”;
if (isPalindrome(s)) {
System.out.println(“The string is a palindrome”);
} else {
System.out.println(“The string is not a palindrome”);
}
The Palindrome function, defined here, checks to see if the given text is a palindrome. If it is, then it returns true; otherwise, it returns false. The function does its job by scanning the string from beginning to end and comparing the two sets of characters at the end. If the characters are different, then the string is not a palindrome.
Conclusion:
To sum up, finding out if a string is a palindrome is a common but difficult programming task that can solve in many different ways. Checking for palindromes in Python can be done with a for loop, a while loop, or a function.
Palindrome string program in Python using for loop lets us go through each character in a string and compare them to their mirrors at the end. This method is easy to use and works very well.
A palindrome program in Python using the function can separate the method for figuring out if a string is a palindrome into its module. This makes the code more reusable and modular. This method could be helpful for more complicated tasks where they must test palindromes in more than one place.
We can start at either end of the string and work our way in, comparing characters as we go with Python’s Python’s while loop, like the for-loop method but a bit wordier.
Even though it can be harder to test for palindromes in C than in Python, there are a lot of resources that show how to do it in a variety of ways.
Lastly, checking for palindromes is a vital programming skill that can use in many ways. Knowing how to use different methods to check for palindromes can help us write easier to maintain, change, and understand code.