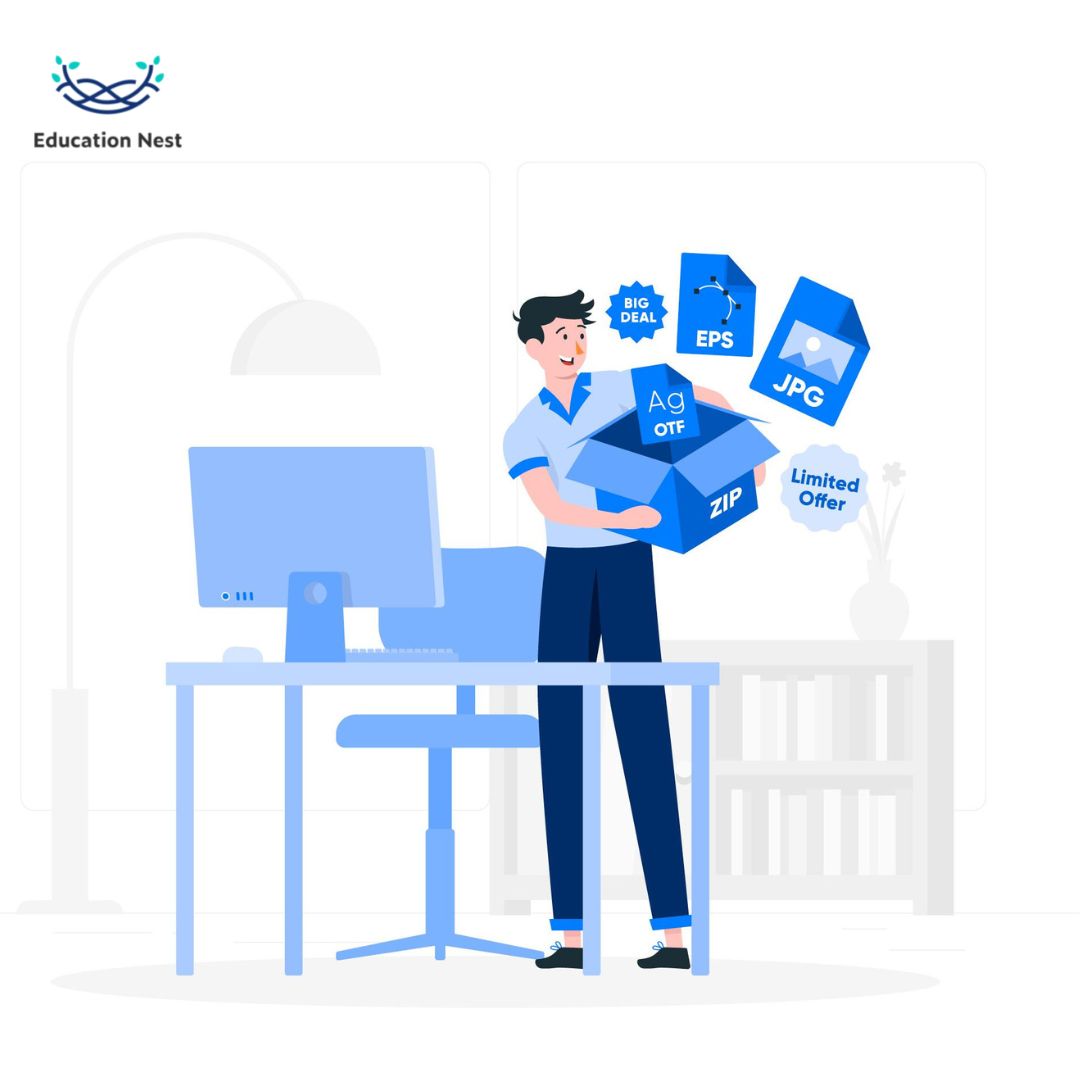
This blog is on how to work with file handling in Python, including creating, reading from, writing to, closing, renaming, and deleting files, with complete notes on file handling in Python.
Files are used to both temporarily and permanently store information. Information saved to a disc and accessible under a specific name is called a file.
We’ll talk about types of file handling in Python and the complete guide to handling files.
In this article, we have also added Python file-handling exercises for your better understanding.
File Handling in Python: An Introduction
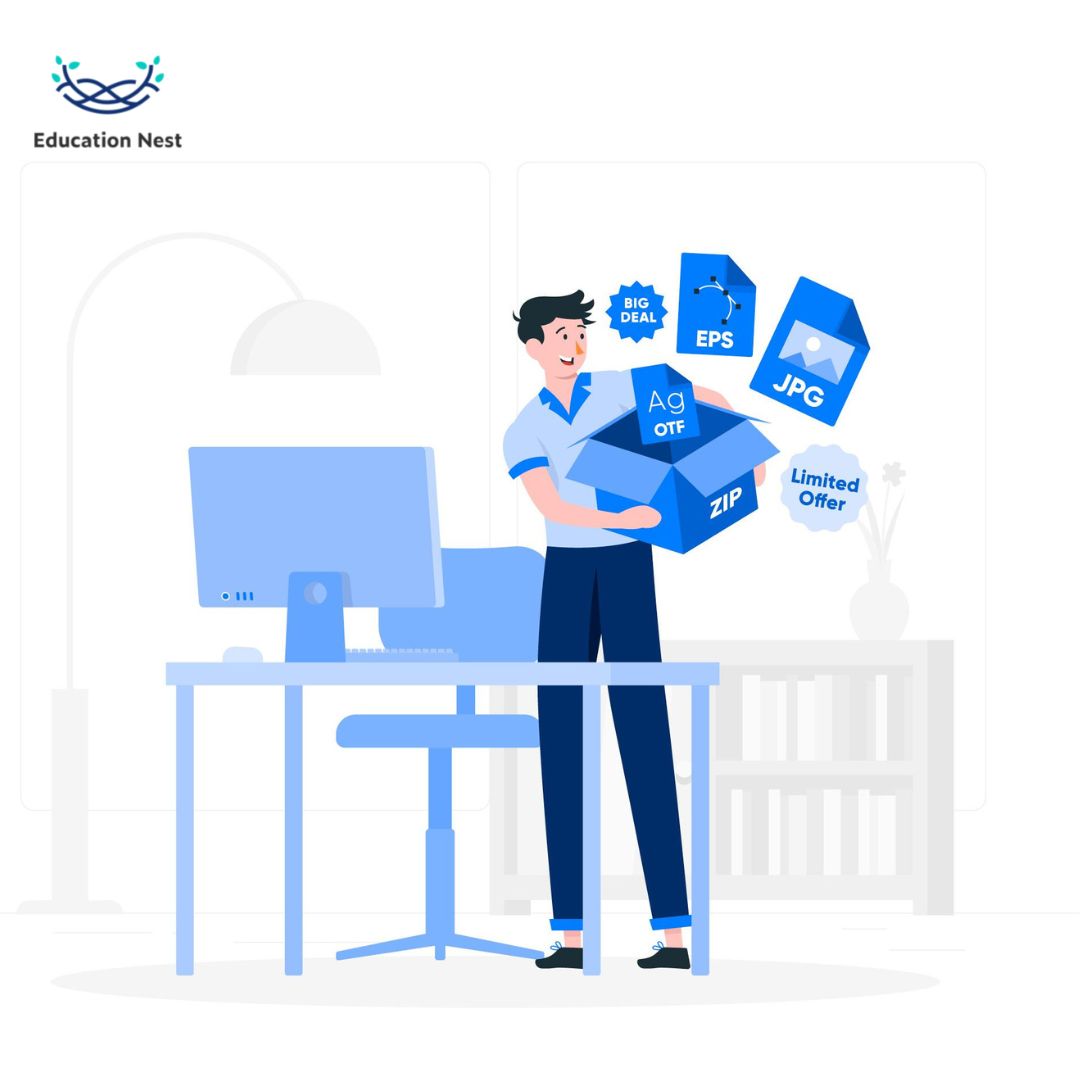
Python has built-in support for handling files, so users can do simple things like read and write files. In other languages, managing files takes a lot of work to implement and takes a long time. In Python, the idea is simple and easy to explain.
Python’s handling of text versus binary files is distinct. A text file comprises lines of code that each have a set of characters. End-of-line (EOL) characters, such as the comma “,” or newline “, mark the end of each line in a file. It signals the end of the current line and the start of the next one for the interpreter. First, let’s get into some basic file I/O.
How to Manage File Handling in Python
- Python file reading
To begin, let’s read some code to read a text file. Before we can read files, we must first create a file object.
- Using Python to Create Documents
Before, we made file objects with the r option, which indicates read mode. The read mode cannot be used for writing files; the write mode (w) must be used instead.
- Python attribute reading
When you store a file on your computer, it will also store information about the file itself, known as metadata or file attributes. This information may include the file’s size, creation date, the last time accessed, etc.
- Making new Python directories
Python’s os.mkdir method lets you create a new directory from scratch.
- Creating folder/ABC, for instance, necessitates the first two subfolders also being developed. The os. makers function will be helpful in those cases.
- Reading directory contents with Python
Python’s os. listdir method is another convenient API for listing directory contents.
- We can use the os. Remove the function in Python to delete files and directories. To avoid errors, you can utilize the os. Path. exists function before calling os. remove.
- Python for searching through files
Disk searches are occasionally required when working with automation programs. Developers frequently use Python scripts to find particular types of files, such as logs, images, and text documents. Python provides multiple options for searching for files:
- Using os.listdir to go over all possible results and a for loop with an if statement to evaluate each possible outcome
The os.walktree method is used to find all entries one by one, and an if statement inside a for loop checks that they are correct.
Using the glob.glob function to query all entries and pull out the ones you require
Because it has built-in filtering, high performance, and only requires a small amount of developer-written code, the third method is the most useful and Pythonic.
- Tasks involving file copying and relocation
The shutil module provides API features for transferring and copying files across platforms.
- Processing Python archives and extracting their contents
Online Python programs, web services, desktop Python programs, and utility Python programs often use archive files to output or enter multiple files at once. For example, if you are making a web-based file organizer, you could let people download a lot of files at once by automatically making a zip file.
File Handling In Python Example
Suppose we have a file called “example.txt” in the same directory as our Python script. The contents of the file are:
Python
Copy code
Hello, world! This is an example file.
To read the contents of the file in our Python script, we can use the following code:
Python
Copy code
# Open the file for reading with open(“example.txt,” “r”) as file: Read the contents of the file: file contents file. ts = file. read() Print the contents of the file. print(contents)
This code opens the file “example.txt” in read-only mode using the open() function and assigns it to the variable file. The with statement ensures that the file is properly closed when we’re done with it.
Then, we used the file object’s read() method to read the file’s contents, which we put into the variables’ scope.
Finally, we print the file’s contents using the print() function.
Output:
Python
Copy code
Hello, world! This is an example file.
To write to the file, we can use the following code:
Python
Copy code
# Open the file for writing with open(“example.txt,” “w”) as file: Write some text to the file. Write (“This is some new text!”) # Open the file for reading with open(“example.txt,” “r”) as file: Read the contents of the file: contents = file. read() Print the contents of the file. print(contents)
This code opens the file “example.txt” in write mode using the open() function and assigns it to the variable file. We then write the string “This is some new text!” to the file using the write() method of the file object.
We reopen the file in read-only mode, read its contents, and print them to the console.
Output:
This is some new text!
The Importance of File Handling in Python
Working with files can be applied to other programming languages, but doing so may take time and effort. Python’s built-in support for managing files makes it easier than most other languages to put into practice.
Also, if you want to analyze the data, you must know how to read the data from a specific file. Because of this, the idea of file handling is crucial.
Types Of File Handling In Python
These items are:
- Binary file
- Text file
Text file
According to one definition, a text file is just a string of letters, numbers, and other symbols. Text documents often have filename extensions like “.txt,” “.py,” “.csv,” and others. When we open a text file in a text editor (like Notepad), the lines of text that show up can be different lengths. Still, this is different from how the file’s contents are saved. Instead, they are kept as a string of 0s and 1s called a “byte.” Whether a text file is encoded in ASCII, UNICODE, or some other way, the bytes representing a character’s value are always the same. This means that when we open a text file, the text editor will take each ASCII value and show us what it looks like in English. In a text editor, for example, the character whose ASCII value is 65 and whose binary value is 1000001 is shown as the letter “A.”
The End of Line character is always used to mark the end of a line in a text file (EOL).
Binary Files
Bytes (0s and 1s) are also used to store information in binary files, but unlike text files, the bytes in a binary file do not correspond to the ASCII values of individual letters. Instead, they stand in for the data themselves, whether it’s an image, music, or video file, a compressed copy of another file, an executable file, etc. A person can’t read these files. So, if you try to open a binary file in a text editor, you will see many random numbers.
We need special software to read or write the data in a binary file.
A string of bytes is how a computer stores binary files. Even changing one bit can corrupt the file, meaning that the program that uses it can no longer use it.
Since humans can’t read what’s in a binary file, fixing any mistakes made when the file was first made is also challenging. We can read and write both text and binary files with Python.
Python file-handling exercises
Q.Explain the differences between
A) a text file and a binary file
B) write() and write lines()
C) readline() and readlines() ()
Q. Use and syntax for the following procedures:
A) open
B) read
C) seek
D) dump ()
Q. Why should we close the file when we finish the read and write operations? What could happen if we don’t shut it down? Will there be a blinking error message?
Q. Create a Python script to open the hello.txt file from question 6 in read mode to view its contents. What would change if the file was opened in write mode instead of append mode?
Q. Please give a pythonic explanation of picklase. Describe how Python objects are serialized and deserialized.
Conclusion
Python’s syntax is straightforward to use. As a result, most file operations can be implemented with little effort. Yet, Python’s standard library must be better designed, leading to the same API functions. Because of this, you need to choose the best standard module that fits your needs.
Handling files is crucial for every web-based program.
For more interactive topics, visit educationnest.com right away!