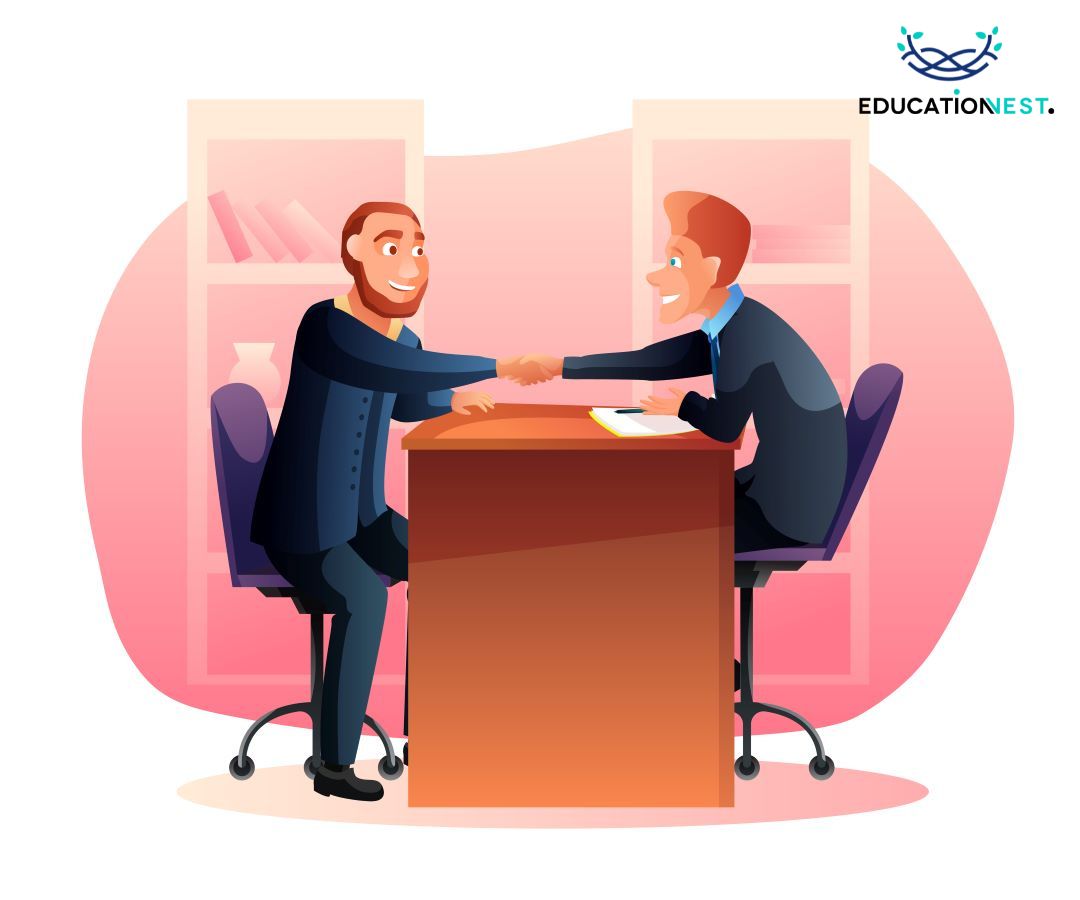
Preparing for a C++ interview can be hard because you need to know a lot about the language’s complexities and how to use it in real life. Whether you are an experienced C++ developer or a recent college graduate looking for your first job, this detailed guide will help you prepare well for your C++ interview. We’ve put together a list of the top C++ interview questions and answers to help you feel confident during your interview. Let’s delve into the world of C++ and explore these questions under various categories.
Basic Concepts C++ Interview Questions
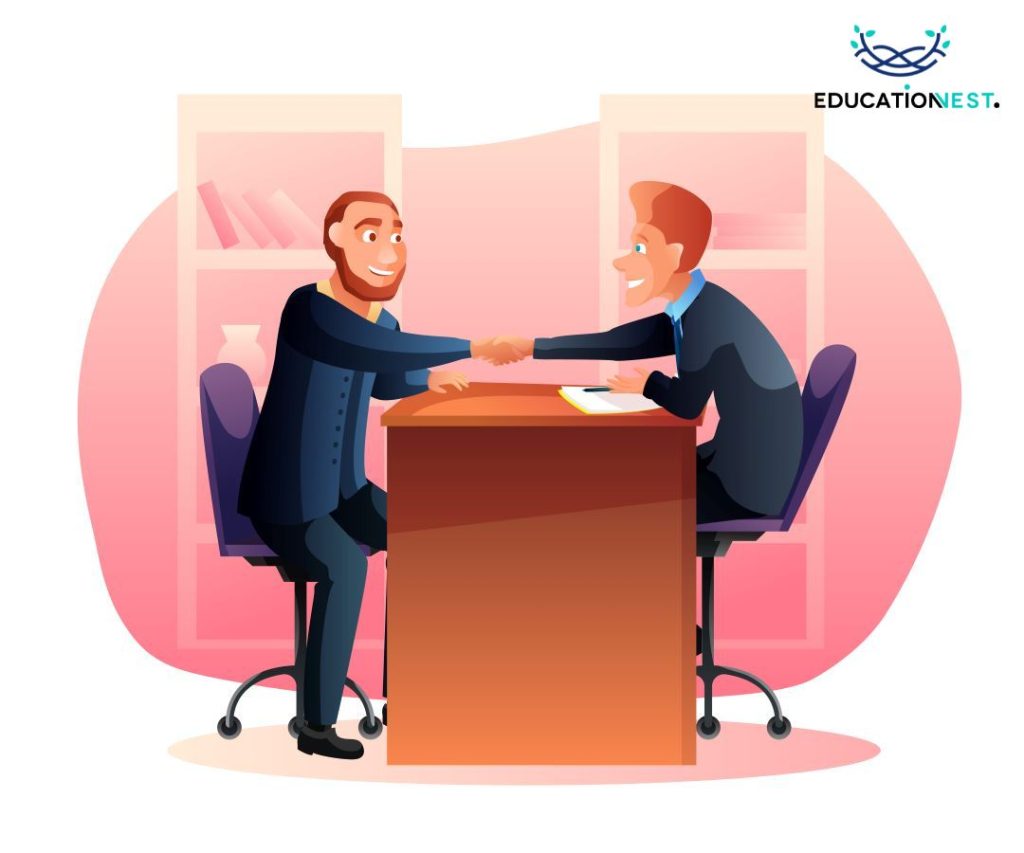
- What is C++?
C++ is a high-level programming language that extends the capabilities of the C programming language. It is an object-oriented programming (OOP) language that combines the features of both high-level and low-level languages.
- What are the key differences between C and C++?
Discuss the main distinctions, such as OOP support, standard libraries, memory management, and more.
- Explain the concept of Object-Oriented Programming (OOP) in C++.
Describe the four fundamental principles of OOP: encapsulation, inheritance, polymorphism, and abstraction.
- What is a class and an object in C++?
Clarify the difference between a class and an object, and explain their roles in C++.
- What is a constructor in C++?
Discuss the purpose of constructors, types of constructors (parameterized and default), and their syntax.
- What is a destructor in C++?
Explain the role of destructors, and how they differ from constructors.
- What is the difference between new and malloc()?
Elaborate on memory allocation methods in C++ and C, highlighting the advantages of the new operator in C++.
Data Types C++ Interview Questions
- What is the difference between a built-in data type and a user-defined data type?
Compare and contrast the two types of data in C++.
- What are the fundamental data types in C++?
Discuss data types like int, float, double, char, and their memory requirements.
- Explain the concept of pointers in C++.
Describe pointers, their declaration, and their role in memory manipulation.
- What are reference variables in C++?
Discuss reference variables, their use cases, and how they differ from pointers.
- What is the difference between stack and heap memory allocation?
Clarify the distinctions between stack and heap memory and their implications in C++.
Control Flow C++ Interview Questions
- What is an if statement in C++?
Describe the syntax and usage of if statements, including nested if statements.
- Explain the switch statement in C++.
Discuss the switch statement, its purpose, and when to use it instead of if-else statements.
- What is a loop in C++?
Introduce loops, including for, while, and do-while loops, and their differences.
- What is the purpose of the break and continue statements in C++?
Explain how break and continue statements affect loop execution.
C++ Functions and Scope
- What is a function in C++?
Define functions, their syntax, and their importance in modular programming.
- What is function overloading in C++?
Discuss function overloading, including its benefits and rules.
- Explain the difference between call by value and call by reference.
Elaborate on the two parameter-passing methods in C++.
- What is the scope of a variable in C++?
Describe the concepts of global and local scope and their implications on variable visibility.
Also Read:
The Ultimate Guide to Top Programming Languages in 2023
Object-Oriented Programming C++ Interview Questions
- What is inheritance in C++?
Discuss inheritance, types of inheritance (single, multiple, multilevel, hierarchical), and access control.
- What is polymorphism in C++?
Define polymorphism and explain static and dynamic polymorphism.
- What are abstract classes and pure virtual functions?
Introduce abstract classes and their use in achieving abstraction in C++.
- What is encapsulation in C++?
Explain encapsulation and the use of access specifiers (public, private, protected).
- What is a constructor chaining in C++?
Discuss constructor chaining and its role in class hierarchies.
Standard Template Library (STL) Interview Questions
- What is the Standard Template Library (STL) in C++?
Provide an overview of STL, its components (containers, algorithms, iterators), and benefits.
- What is a vector in C++?
Describe the vector container, its features, and common operations.
- Explain the difference between a set and a map in C++ STL.
Clarify the distinctions between set and map containers and their use cases.
- What is an iterator in C++ STL?
Discuss iterators, their types (begin, end), and their role in STL algorithms.
- What are algorithms in C++ STL?
Introduce commonly used algorithms like sort, find, and transform.
File Handling Interview Questions
- How do you open and close a file in C++?
Describe the file handling process in C++.
- What are the modes of file opening in C++?
Explain file modes (read, write, append) and their uses.
- What is the difference between text and binary file modes?
Discuss the distinction between text and binary file handling.
Error Handling Interview Questions
- What is exception handling in C++?
Explain the concept of exception handling, including try, catch, and throw keywords.
- What is the importance of the std::exception class in C++?
Describe the std::exception class and its role in exception hierarchy.
- What is RAII (Resource Acquisition Is Initialization) in C++?
Introduce RAII and how it helps manage resources safely.
C++ Best Practices
- What are the best practices for coding in C++?
Discuss coding conventions, naming conventions, and code optimization techniques.
- Explain the importance of memory management in C++.
Highlight the significance of proper memory management, including avoiding memory leaks.
- What are smart pointers in C++?
Describe smart pointers (unique_ptr, shared_ptr, weak_ptr) and their use in managing memory.
Advanced Topics C++ Interview Questions
- What is template metaprogramming in C++?
Introduce template metaprogramming and its applications in creating generic code.
- Explain the concept of multi-threading in C++.
Discuss multi-threading, its advantages, and potential pitfalls.
- What is move semantics in C++?
Describe move semantics, including move constructors and move assignment operators.
C++ Interview Tips
Now that we have covered these essential C++ interview questions, here are some tips to help you succeed in your C++ interview:
Practice coding: Solve coding challenges and work on C++ projects to reinforce your skills.
Review your C++ fundamentals: Ensure you have a strong grasp of the basics, as they form the foundation for more advanced topics.
Prepare for technical questions: Be ready to explain your thought process and problem-solving approach during technical discussions.
Research the company: Familiarize yourself with the company’s products, culture, and values to tailor your answers accordingly.
Ask questions: At the end of the interview, ask thoughtful questions to demonstrate your interest and engagement.
Conclusion
In this comprehensive guide, we’ve covered 40+ essential C++ interview questions and answers across various categories, including basic concepts, data types, control flow, functions, object-oriented programming, the Standard Template Library, file handling, error handling, best practices, and advanced topics. Remember that success in a C++ interview goes beyond memorizing answers; it requires a deep understanding of the language and the ability to apply your knowledge to real-world scenarios. By studying these questions and practicing your coding skills, you’ll be well-prepared to excel in your C++ interview and land the job of your dreams. Good luck!