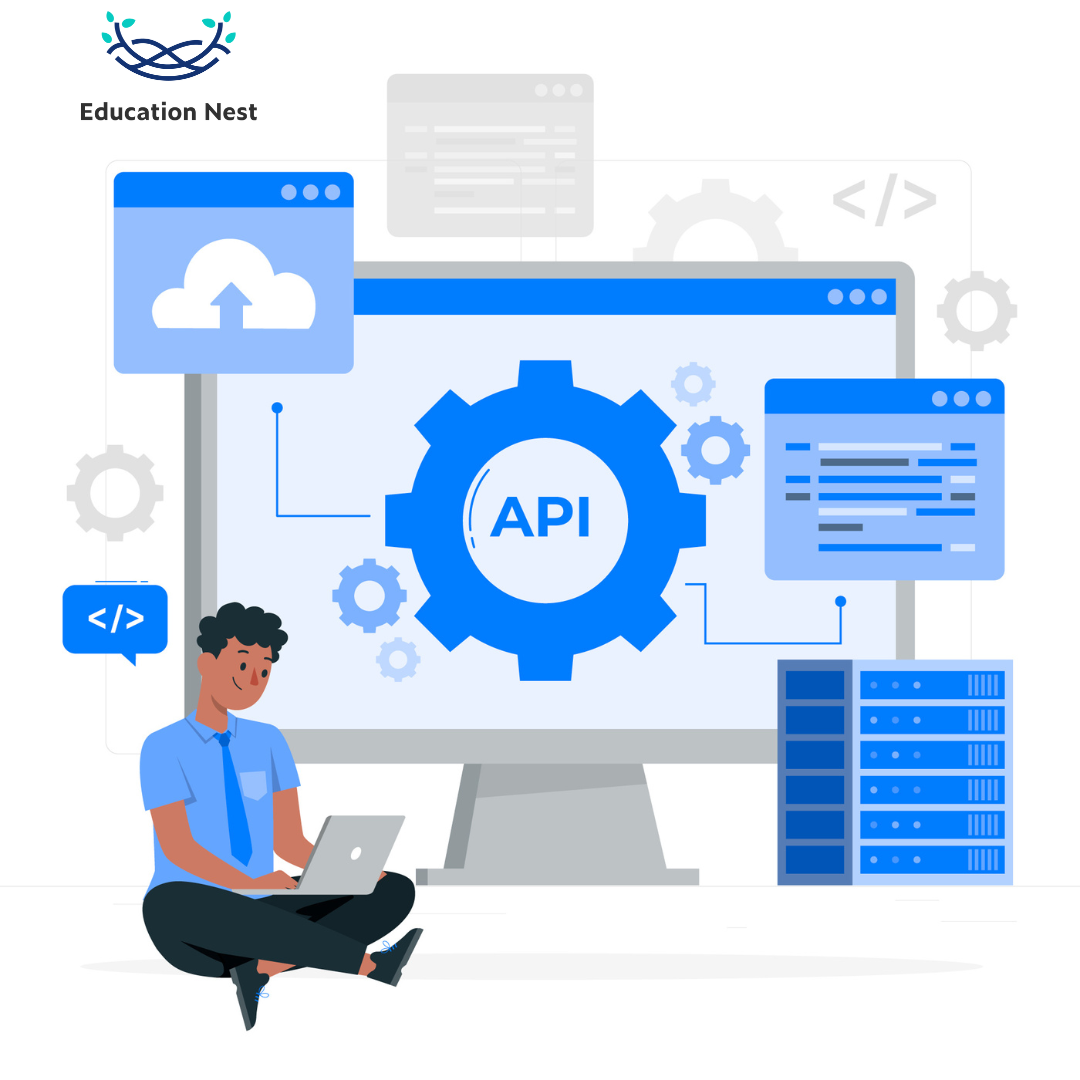
A REST API, also known as a REST API, is a web API that adheres to the REST architectural style and allows you to communicate with RESTful web services. REST is an abbreviation for “representational state transfer.” Roy Fielding, a computer scientist, invented it.
What is REST API?
REST is a set of architectural guidelines. REST provides API developers with numerous options.
Requesters or endpoints receive resource state representations from REST APIs. This information is sent via HTTP using JSON, HTML, XLT, Python, PHP, or plain text. Despite its amusing name, JSON is the most widely used file format because it is language-independent and can be read by humans and machines.
Remember that RESTful API HTTP request headers and parameters include metadata, authorization, URI, caching, cookies, and other information. Headers for the request and response. Each header contains a status code as well as HTTP connection information.
This is a public API implemented as RESTful web service (it follows REST conventions). Your browser will show a single JSON-formatted quiz question with answers, such as:
{
“response_code”: 0,
“results”: [
{
“category”: “Science: Computers”,
“type”: “multiple”,
“difficulty”: “easy”,
“question”: “What does GHz stand for?”,
“correct_answer”: “Gigahertz”,
“incorrect_answers”: [
“Gigahotz”,
“Gigahetz”,
“Gigahatz”
]
}
]
}
You could request the same URL and get a response using any HTTP client, such as curl:
curl “https://opentdb.com/api.php?amount=1&category=18”
RESTAPIs must meet the following requirements
- Client-server architecture based on HTTP with clients, servers, and resources.
- Stateless, client-server communication means no client data is stored between get requests.
- Data that can be cached to speed up client-server connections.
- A standard mechanism for components to exchange data. This implies that the client’s requested resources must be distinct.
- The client is given enough information to make changes to resource utilization.
- Clients are capable of handling self-descriptive messages.
- When clients access a resource, they should be able to use hyperlinks to find immediate alternative activities. This is possible with hypertext and hypermedia.
- Each server (security, load balancing, and so on) has a layered system for retrieving data into hierarchies that customers cannot see.
- Code-on-demand is an optional feature that lets the server transmit executable code to the client when requested. Client power grows.
Even though it must meet these standards, the REST API is more user-friendly than SOAP (Simple Object Access Protocol), which is slower and heavier due to its XML messaging and built-in security and transaction compliance.
REST is an adaptable set of standards. REST APIs are faster, lighter, and more scalable, making them ideal for IoT and mobile app development.
Difference Between SOAP and REST API.
We’ll examine some of the most significant differences between the two paradigms below.
- REST is an architectural style, whereas SOAP is a set of rules.
SOAP employs a service interface for an API, whereas REST uses URIs. An API is designed to make certain parts of a server’s business logic available. REST APIs are designed to provide data, whereas SOAP APIs are designed to provide functions. A SOAP API, for example, might have a process called “CreateUser” that allows you to create users. The SOAP body would describe this function. A REST API user would be created when a POST request was sent to the URL /users.
- SOAP APIs obtain information from a resource, whereas REST APIs act (a URI).
SOAP is a standard protocol for exchanging structured data, whereas REST is more function-driven. SOAP only supports XML, whereas REST supports various data formats, including plain text, HTML, XML, and JSON, which makes sense for data and makes it easier for browsers to collaborate. As shown in the example above, SOAP APIs can only use XML and the SOAP envelope, header, and body formats. REST APIs, on the other hand, are format-independent. Although JSON is the most widely used format, REST APIs can also work with XML, plain text, and file types.
- Security is handled in various ways.
At the transport level, WS-Security outperforms SSL and has more features. It is also SOAP-compliant, making it ideal for integrating with enterprise-level security products. For complete security, both can use SSL, and REST can also use HTTPS, which is the secure version of HTTP. WS-Security, SOAP’s additional layer of protection, works on the message level to ensure that not only the correct server but also the proper process on the server can read the content of a message. HTTPS and SSL can be used to encrypt the connection between a SOAP API and a REST API.
- REST consumes less bandwidth and resources than SOAP (depending on the API)
Because the payload is sent in an envelope, SOAP has a little more overhead at first. Because REST is frequently used for web services, being light in these situations is advantageous.
As the example SOAP request in the previous section shows, a SOAP request contains more information than a REST request. This means that if you use a SOAP API, you will need to use more bandwidth. This could slow down heavily used systems.
You Must Like: Snake Game Python Code: How to Build an Addictive Game in Just a Few Lines
RESTAPI in Spring boot
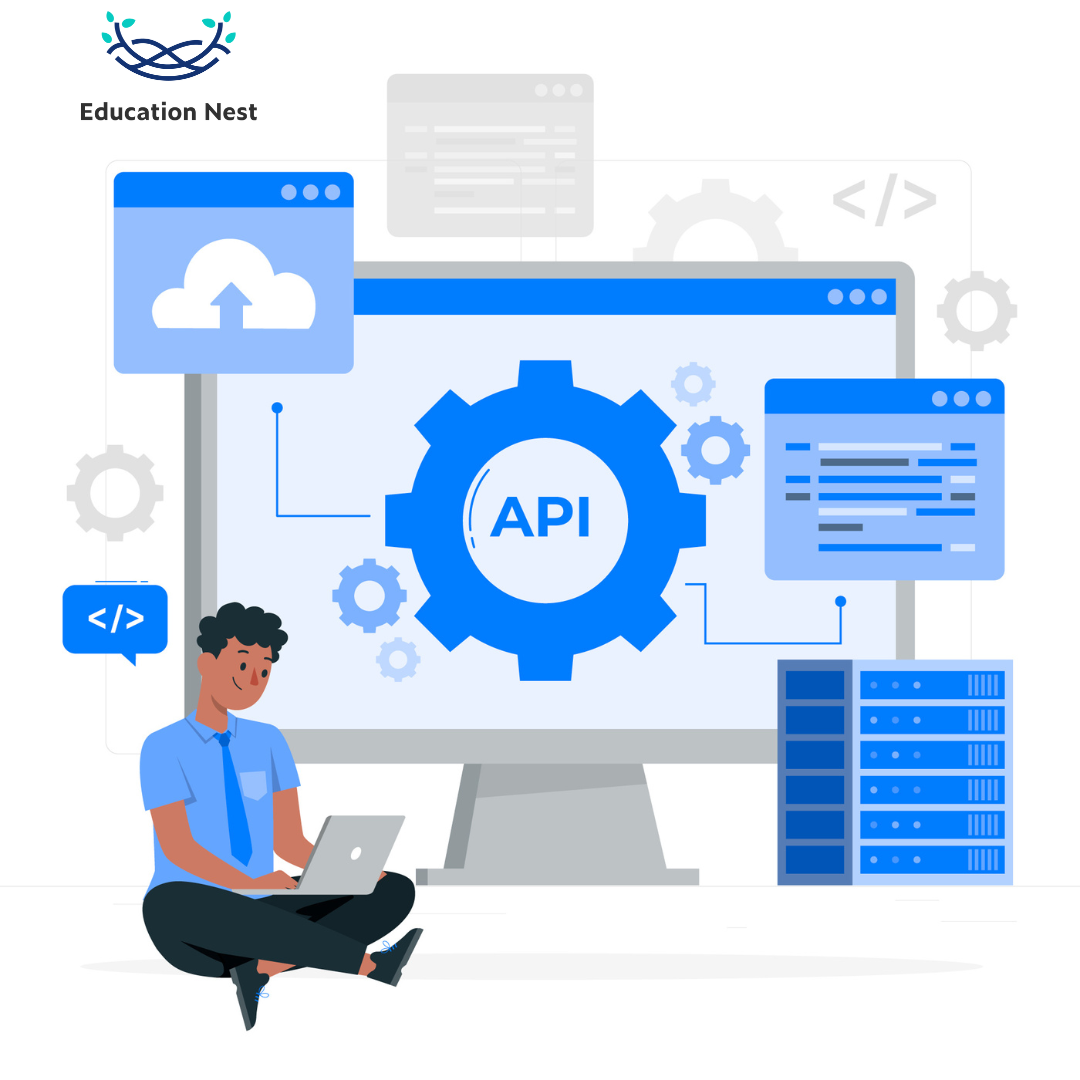
Springboot is frequently used to create scalable applications. Spring MVC is a popular Spring module for building scalable web apps. It is compatible with web apps. The main issue with spring projects is that they require a lot of work and can be challenging to understand for new developers. SpringBoot can help with this. SpringBoot is built on top of the spring and has all of the spring’s characteristics. In this tutorial, we’ll create a RESTAPI to retrieve the employee list and add people to it. To begin, create a basic SpringBoot project in one of the IDEs and then do the following:
package com.example.demo;
// Creating an entity Employee
public class Employee {
public Employee() {}
// Parameterized Constructor
// to assign the values
// to the properties of
// the entity
public Employee(
Integer id, String firstName,
String lastName, String email)
{
super();
this.id = id;
this.firstName = firstName;
this.lastName = lastName;
this.email = email;
}
private Integer id;
private String firstName;
private String lastName;
private String email;
// Overriding the toString method
// to find all the values
@Override
public String toString()
{
return “Employee [id=”
+ id + “, firstName=”
+ firstName + “, lastName=”
+ lastName + “, email=”
+ email + “]”;
}
// Getters and setters of
// the properties
public Integer getId()
{
return id;
}
public void setId(Integer id)
{
this.id = id;
}
public String getFirstName()
{
return firstName;
}
public void setFirstName(
String firstName)
{
this.firstName = firstName;
}
public String getLastName()
{
return lastName;
}
public void setLastName(
String lastName)
{
this.lastName = lastName;
}
public String getEmail()
{
return email;
}
public void setEmail(String email)
{
this.email = email;
}
}
public Employees getAllEmployees()
{
return list;
}
}
After implementing all the classes in the project, run the project as Spring Boot App. Once the server starts running, we can send the requests through the browser or postman.
REST API using Java
To build a RESTful web service in Java, you typically define the resource(s) that the service will expose, define the endpoints (URIs) that clients will use to access those resources, and implement the business logic that handles the requests and responses. This can be done using annotations and Java classes that define the resource methods (GET, POST, PUT, DELETE, etc.) and their parameters and return types.
Here is an example of a simple REST web service using Spring Boot:
@RestController
@RequestMapping(“/api”)
public class MyController {
@GetMapping(“/greeting”)
public String greeting(@RequestParam(value = “name”, defaultValue = “World”) String name) {
return “Hello, ” + name + “!”;
}
}
REST API in Python
Python provides several libraries and frameworks for building REST APIs, including Flask, Django, Pyramid, and FastAPI.
Here’s an example of building a simple REST API using Flask:
from flask import Flask, jsonify, request
app = Flask(__name__)
# sample data
books = [
{“id”: 1, “title”: “Python for Beginners”, “author”: “John Doe”},
{“id”: 2, “title”: “Python Web Development”, “author”: “Jane Smith”},
]
# get all books
@app.route(‘/books’, methods=[‘GET’])
def get_books():
return jsonify(books)
# get a specific book by id
@app.route(‘/books/<int:book_id>’, methods=[‘GET’])
def get_book(book_id):
book = next(filter(lambda x: x[‘id’] == book_id, books), None)
if book:
return jsonify(book)
else:
return jsonify({“message”: “Book not found”}), 404
# add a new book
@app.route(‘/books’, methods=[‘POST’])
def add_book():
new_book = request.json
new_book[‘id’] = max(book[‘id’] for book in books) + 1
books.append(new_book)
return jsonify(new_book), 201
# update a book by id
@app.route(‘/books/<int:book_id>’, methods=[‘PUT’])
def update_book(book_id):
book = next(filter(lambda x: x[‘id’] == book_id, books), None)
if book:
book.update(request.json)
return jsonify(book)
else:
return jsonify({“message”: “Book not found”}), 404
# delete a book by id
@app.route(‘/books/<int:book_id>’, methods=[‘DELETE’])
def delete_book(book_id):
book = next(filter(lambda x: x[‘id’] == book_id, books), None)
if book:
books.remove(book)
return ”, 204
else:
return jsonify({“message”: “Book not found”}), 404
if __name__ == ‘__main__’:
app.run(debug=True)
REST API in node JS
- Ensure that Node.js is installed on your computer. I’ll use version 14.9.0 for this article, but it may also work with earlier versions.
- Next, ensure that MongoDB is installed. Start the server interactively (as mongo from the command line) rather than as a service. This will get the fundamentals up and running. We will not discuss how Mongoose and MongoDB are used in this case. This is because, at some point in this tutorial, we’ll need to communicate with MongoDB directly rather than through our Node.js code.
- Navigate to the resulting rest-api-tutorial/ folder in your terminal. You’ll see that our project contains three module folders:
- (handling all shared services, and information shared between user modules)
- users (everything regarding users)
- auth (handling JcommonWT generation and the login flow)
- Now, run npm install (or yarn if you have it.A simple Node.js REST API back end is now configured and has all the dependencies required.
Conclusion
In this article we have come to know about REST API and how it can be used through Spring boot. It also states how it is brought in Node js, Python and Java.