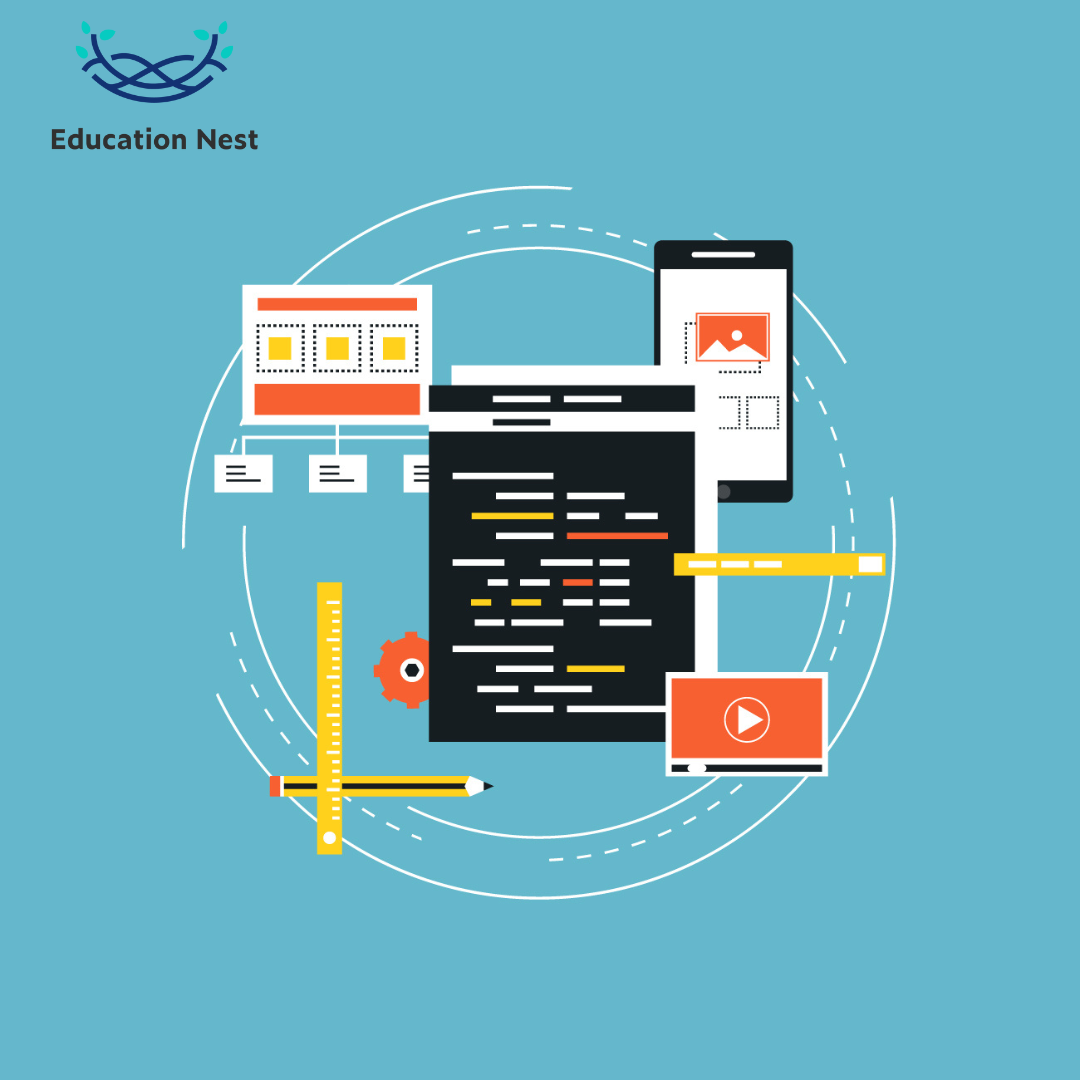
A data type specifies the sizes and values it can store. In Java Data Type, there are two kinds of data types:
- Primitive Data Type
- Non Primitive Data Type
Primitive data types include Booleans, chars, bytes, short ints, long ints, floats, and doubles.
Data types that are relatively old Classes, Interfaces, and Arrays are examples of non-primitive data types. Primitive data types are the foundation of how Java interacts with data. These are the most fundamental types of data that the Java programming language can handle.
Java, a programming language, defines data types. According to this rule, all variables must be declared before being used. As a result, we must specify the type and name of each variable.
Primitive Java Data Type
Primitive data types are classified into eight categories:
- boolean data type
- byte data type
- char data type
- short data type
- int data type
- long data type
- float data type
- double data type
Boolean Data
You can only store two possible values with the Boolean data type: true and false. Simple flags that keep track of whether something is true or false are used with this type of data. Even though the Boolean data type only specifies one piece of information, its exact “size” is unknown.
Example:
1 Boolean is false.
Byte Data
Simple data types include the byte data type. It is an 8-bit number with two’s complements. It can range between -128 and 127. (inclusive). It has a minimum value of -128 and a maximum value of 127. The default setting is 0.
The byte data type is used when memory is at a premium, such as in large arrays. Because a byte takes up four times less space than an integer, space is saved. This can be used in place of the “int” data type.
Example:
Byte A equals 10, and Byte B equals -20.
Short Java Data Type
The short data type is a 16-bit integer with a signed two’s complement value. The values range between -32,768 and 32,767. (inclusive). The values range from -32,768 to 32,767. The default setting is 0.The short data type, like the byte data type, can be used to save memory. A short data type is one-half the size of an integer.
Example:
Short s is equal to $10,000, and Short r is equal to $5,000.
You Must Know: Master the Advanced Java Tutorial: A Complete Guide
Integer Java Data Type
The int data type which stands for a signed 32-bit two’s complement integer. Its value can range from -2,147,483,648 (-31) to 2,147,483,647 (-31). (inclusive). It has a minimum value of 2,147,483,648 and a maximum value of 2,147,483,647. The default setting is 0.Unless there is a memory issue, the int data type is typically used for integral values.
Example:
int a = 100000, int b = -200000
Long Java Data Type
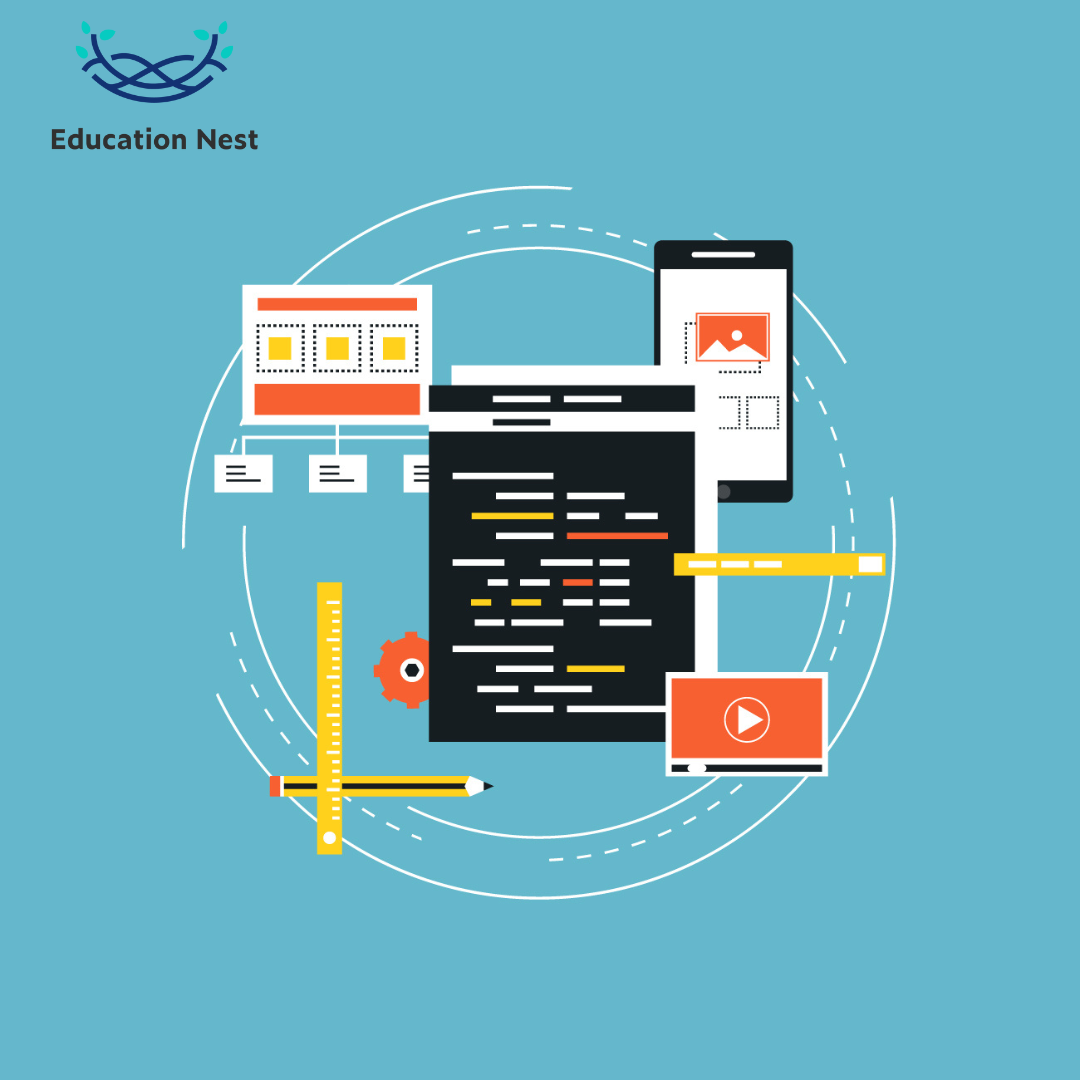
An integer is a 64-bit long data type with the value two’s complement. Its possible values range from -9,223,372,036,854,775,808 (-263) to 9,223,372,036,854,775,807 (-263). (-263-1). (inclusive). The range is from -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807. The default setting is 0. When you need a more extensive range of values than the int data type can provide, use the long data type.
Example:
- long a = 100000L, long b = -200000L
Type of Data: Float
The float data type is a 32-bit IEEE 754 floating point with single precision.
It can be given any value. If you need to save memory in large arrays of floating point integers, use a float (instead of a double). Use the float data type only for numbers that must be exact, such as money. The default value is 0.0F.
Example:
float f1 = 234.5f
Double Java Data Type:
The IEEE 754 double data type is a double-precision 64-bit floating point. It can be given any value. Like the float data type, the double data type is frequently used for decimal values. Furthermore, the double data type should never be used for precise numbers such as money. The default value is 0.0d.
Example:
double d1 = 12.3
Char Data Type
The char data type is a single 16-bit Unicode character. Its value-range lies between ‘\u0000’ (or 0) to ‘\uffff’ (or 65,535 inclusive).The char data type is used to store characters.
Example:
char letterA = ‘A’
Non Primitive Data type
Non-primitive data types are sometimes called “reference data types” because they make references to objects.
the following are non-primitive data types:
- Class
- Array
- String
- Interface
Let’s take a look at each one individually.
1. Class
Objects are created using a user-defined data type known as a class. A class is a collection of things and methods that all members of the class share.A class’s declaration includes the following elements:
- Access Modifier: A class can have default access, meaning it does not require an access modifier or can be marked as public by using the public keyword.
Class names must begin with a capital letter, according to the rules. It is used to create objects and determine which class they belong to.
A parent class is not always required, but if a class needs to extend its parent class and inherit its properties, the parent class’s name can be written with the word spread in front of it.
- Interfaces: A class can use any number of interfaces if a list of interfaces follows the keyword implements.
- Body and Members: A class’s data members and methods are contained in the body, surrounded by curly braces.
Example
In the following example, we’ve separated the Main class’s public interface from the Demo class.
It has two methods for addition and subtraction: add and sub. It also includes a function called Object() that prints the value of the res variable. This function is automatically called (class member variable) when an object is created.
2.String
Unlike character arrays, which contain independent char entities, Java strings are created in a way that they can store a series of characters in a single variable. In contrast to C/C++, Java does not require the null character at the conclusion of strings.
3. Array
In Java, arrays are non-primitive data structures that store items of the same data type next to each other. Because arrays are not pre-configured, users must declare and initialize them. A unique reference name is used to access each item in an array. Things are kept in an indexed format, with a starting index value of 0.
Considerations when Using Arrays in Java
All arrays in Java are created on the fly, and a programmer can change the size of an array while it is running. This means that Java array memory space is allocated when the programme is executed rather than written.
An array’s size must be specified as an integer, not a long or short value.
4. Interface
The interface is a Java tool that allows you to separate things. Abstract methods, or those that have yet to be implemented, can be found in interfaces lacking the method body. This is the most significant distinction between a class and an interface. Java interfaces can have the following properties:
- abstract techniques
- static and default methods (since Java 8)
- Private methods (since Java 9)
There are a few things to keep in mind about Java interfaces:
If a class implements an interface, it must also implement all of the interface’s abstract methods. If it does not, the class must be referred to as an abstract class.
The interface specifies which methods the class must implement. Consider the interface to be the class’s blueprint.
Conclusion
- Java programming language has two categories of java data types which are classified as Primitive and Non-Primitive data types.
- Non-Primitive data types in Java are user-defined & can be easily created or modified by the programmers. They can be used to store multiple values of either the same data type or different data types in a single variable. They always start with the initial first letter in capital.
- Non-Primitive data types are classified as Array, Class, String, and Interface.