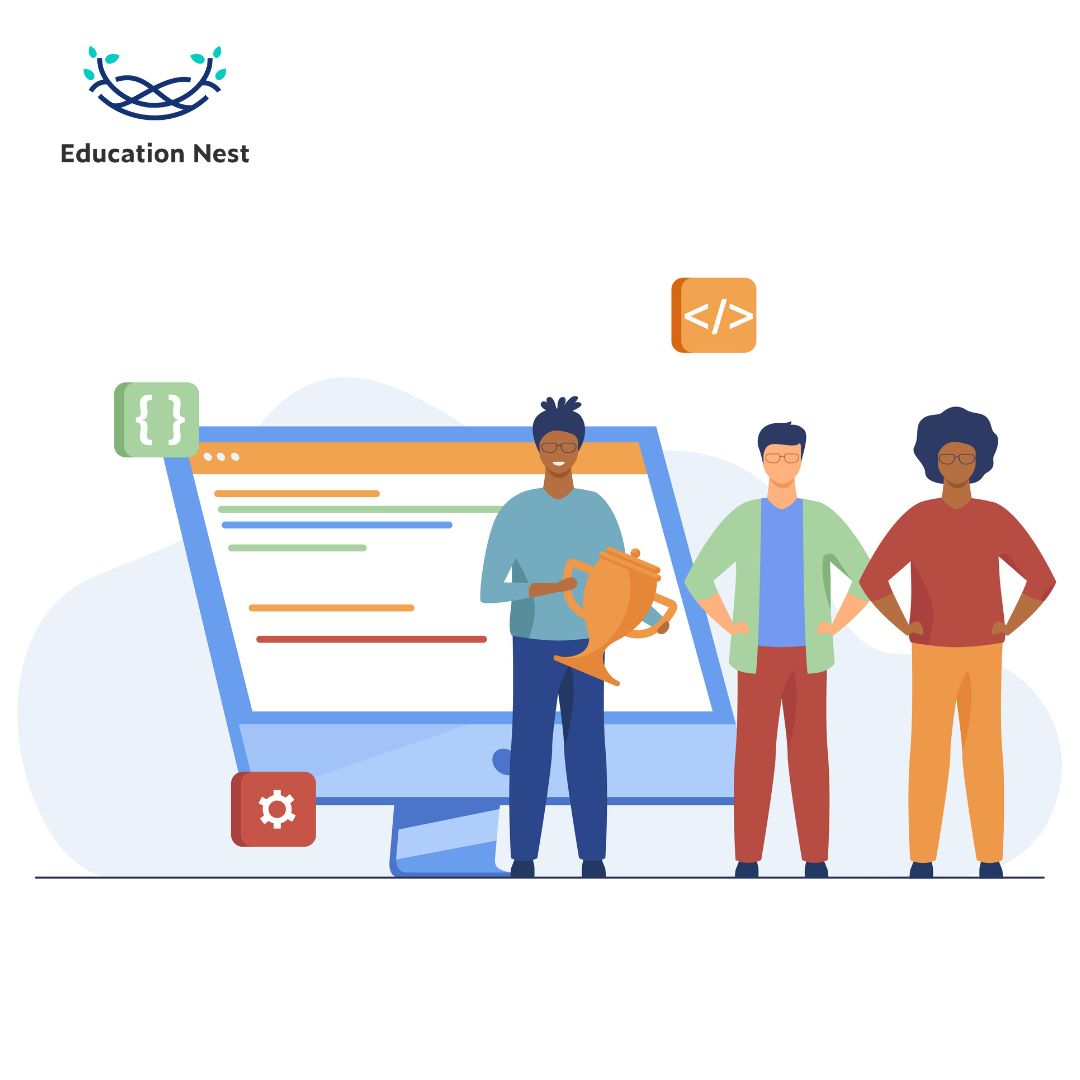
In this tutorial, we’ll discuss the basics of the Python queue, such as what it is and different ways to implement it in Python.
What Is Python Queue
A Python queue is a type of linear data structure that is used to store data in order.
Queues are different from arrays and lists because the data stored in them can’t be accessed in any way. Instead, the data in a queue is in a certain order. So if you add something to a queue, it will go at the end. This is called a FIFO queue, which stands for “first-in, first-out.”
An example of a Python queue can be any sort of line where we all stand from time to time, sometimes in front when we are lucky, or maybe at the end. Such as we stand in line to see a movie, at the grocery store checkout, or at the cafeteria,
But with the help of a Python queue, we can control how our tasks are done.
What is the Use of a Queue?
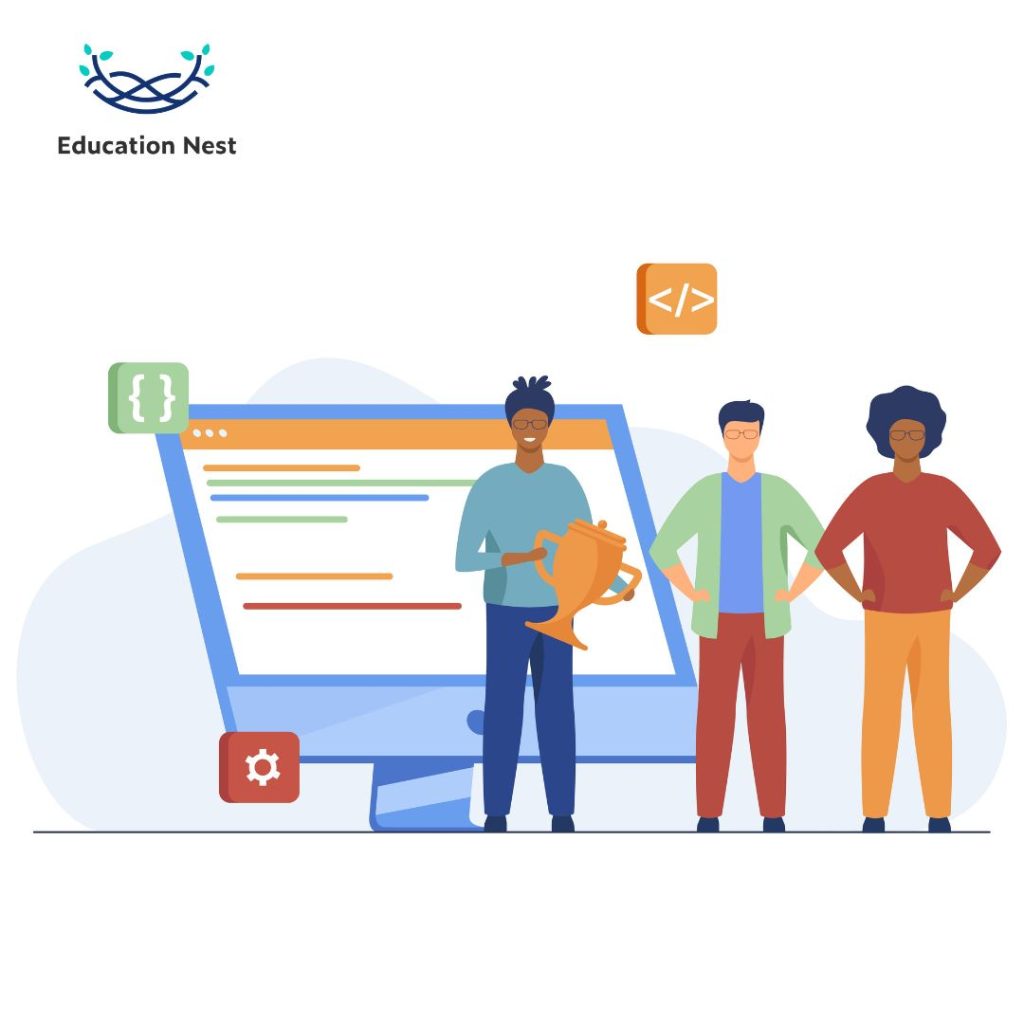
Queues are used in technology, transportation, and operational research for storing and retaining things like data, items, individuals, or events so that they can be dealt with afterwards. In these situations, the queue works like a buffer of data.
There are different kind of queue in Python
Python has four different kinds of queues. They are as follows:
- Python First-in First-out Queue
- Python Last-in First-out Queue
- Python Priority Queue, and
- Python Circular Queue.
What is Python Multiprocessing Queue
Using Python’s multiprocessing queue module, we can create separate processes that can run on different cores of a computer at the same time. One way for these processes to communicate with one another is through queues.
There are two types of queues in the multiprocessing module:
- The Queue class makes it easy to create a queue that can be used by multiple processes.
- The manager(). Queue() class generates a queue, which is managed by a separate process called a manager.
The multiprocessing queue creates a queue in which multiple processes can be used to send messages to one another. The queue is built with shared memory, which lets processes converse with each other quickly and easily.
It also lets you set the queue’s maximum size to ensure it is limited to holding a certain number of items at one time. This can be useful for managing the queue’s memory usage or ensuring that the queue does not become overloaded with items.
Read More: Top 10 Python Frameworks You Need to Know for Web Development
Python Queue Vs Deque
In Python, you can use a standard list as a queue. But adding and removing items from a list can be very slow because changing an item at the top of the list means moving all the other items in the list down. So, if you need a first-in, last-out list, you should use a queue.
Deque is a hybrid data structure that doesn’t use either FIFO or LIFO to add and remove items. It is simply a hybrid data structure that is put together with a dynamic array. Compared to the queue, it is more efficient in terms of time and use of resources. With the Deque data structure, you can get to each element in any sequence of data.
Let’s talk about a few of the most significant differences between Deque and Queue:
Sr. No | Deque | Queue |
1 | As the deque is a sequence container, the insertion of elements takes place in both directions, i.e., front and rear. | As is the general behavior of queues, insertion of the elements takes place from the front, i.e., from the rear. |
2 | In deque, deletion of elements takes place from both the front and rear. | In the normal queue, deletion of elements takes place only from the front. |
3 | Since the deque has the ability to expand and contract from both ends, it is implemented as a dynamic array in the programs. | When talking about the implementation of queues in a programming language, they are implemented as container adaptors. |
4 | Elements can easily be accessed in the deque using the iterators. | It is not possible to access the elements of the queue using the iterators. |
5 | Deque is basically a hybrid data structure that provides the functionality of both the stack and queue in a single data structure. | A queue is a single data structure that provides the facility of only having the Queue data structure. |
6 | Insertion and deletion of elements are more efficient as the resources are completely utilized in the case of the deque. | Insertion and deletion of elements are not as efficient in Qqueueas ctheyto dearee. |
Implementation of Python Queue
There are different ways to make a queue function in Python.
Let’s further discuss how to set up a queue using Python structures for data and modules.
Queues can be set up in Python in the following ways:
- Python Queue Peek
The first item in a Python queue is shown by using the peek function. It gives back the item in the queue that is at the front of the index. It won’t delete the first item, but it will print it.
- Python Queue List
List is a data structure that comes with Python and can be used as a queue. append() and pop() are used instead of enqueue() and dequeue(). But lists are slow for this because adding or removing an item from the beginning requires moving all the other items one by one, which takes time.
- Python Queue Pop
The items pop out in the same order that they were pushed in.
- Python Queue Task Done
Queue.task_done() shows that a task that was put on the queue is done. used by threads that use a queue. For each call to get() to get a task, a call to task_done() tells the queue that the task is being worked on.
If a join() is currently blocking, it will start again when all items have been processed, which means that a task_done() call has been received for each item that was put into the queue.
If called more times than there are items in the queue, a value error is thrown.
Conclusion
Python is a growing language that is also getting more and more important in big data science and AI projects.
In this Python tutorial, we learned about queues, how to use them, and the main differences between a dequeue and a queue.
I’m sure by now you know that the first-in-first-out rule applies to Python queues.