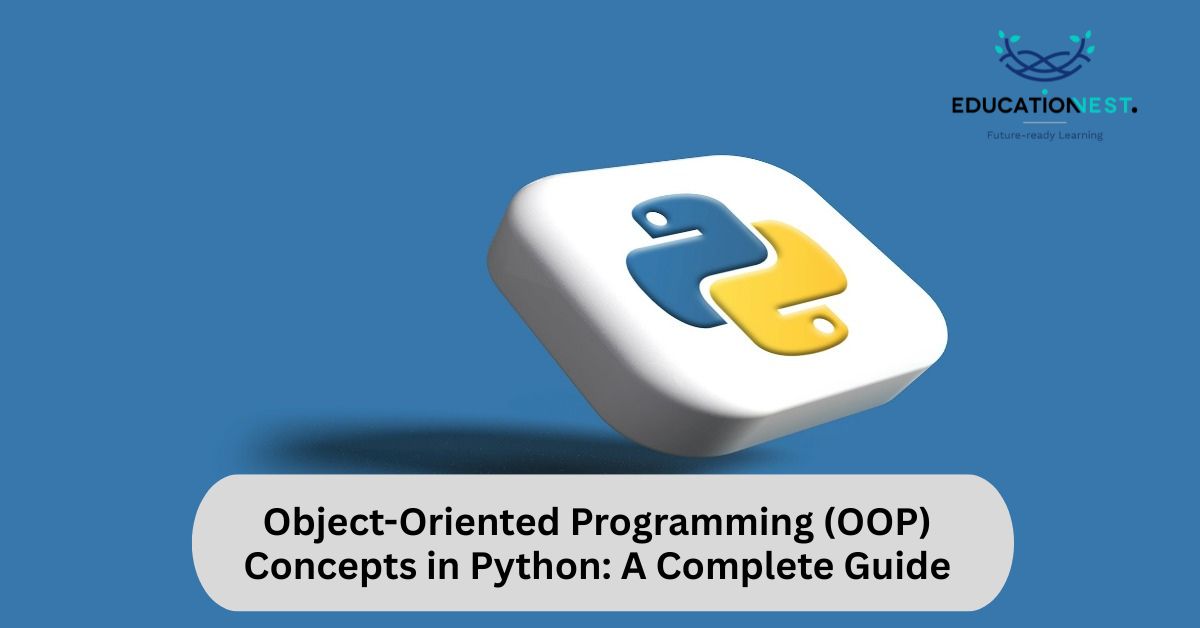
Python is one of the most used programming languages. Both beginners and experienced professionals highly prefer it because of its simple, flexible structure and versatile functions. Its extensive library makes building codes from pre-existing modules easier, and you do not need to start from scratch.
Learning Python can add a competitive edge if you are an IT professional. As per recent reports, Python ranks at the top of the TIOBE index, which rates programming languages based on their popularity. Therefore, it is time to invest in developing your Python programming skills.
Looking for effective training programs to enhance your professional competencies? Education Nest can help you with the best corporate learning solutions designed and delivered by seasoned industry experts. These courses are developed considering the latest market standards and requirements and ensure tangible outcomes through effective performance metrics.
OOP, or object-oriented programming, is one of the key concepts in Python. It promotes a modular approach to writing reusable codes to increase productivity and scalability. It also helps in modeling real-world concepts and interactions and simplifies code maintenance.
So, what are OOP concepts in Python? Read on to know more.
What is OOP?
Object-oriented programming, or OOP, is a structured programming concept that uses classes and objects to define different data attributes and behaviors. It does not follow the procedural programming framework or logic-based system. Instead, it binds data and functions for more effortless project development and maintenance.
The best part about OOP is that the system functionalities can be easily upgraded without changing the entire code. Besides, you can implement it independently without needing significant adjustments. This saves a lot of time, effort, and resources. It can also be used in diverse languages like C, C++, Java, Python, Ruby, and PHP.
OOP Concepts in Python
Since Python emphasizes flexibility and usability, it supports OOP concepts. Here are some key OOP concepts used in Python:
Objects
Objects are the heart and soul of OOP. It is the fundamental block with a set of properties and behaviors defined by the user. They have distinct features and unique identities to differentiate them. They can also interact with each other using functions.
Class
The class helps define the object. It is a user-designed template to create specific data structures and what operations can be performed on them. You can create multiple object instances for the same class.
For instance, you want to create a class called Animals with attributes like name and their species. You can then create different objects from the class Animals and pass different functions.
Here’s a simple example:
Creating class:
class Animal: #to define the class named Animal
def __init__(self, name, species): #to initialize the attributes
self.name = name #self is the instance of the class
self.species = species #there are two attributes-name and species
def speak(self): #defining a function named speak
return f”I am a {self.species} named {self.name}.”
For creating objects:
dog = Animal(“Buddy”, “dog”) #to create an object dog of the class Animal
cat = Animal(“Whiskers”, “cat”) #to create an object cat of the class Animal
#calling the function speak and printing the output:
print(dog.speak()) # Output: I am a dog named Buddy.
print(cat.speak()) # Output: I am a cat named Whiskers.
Here, the class Animal has two objects—a dog and a cat. They have the same attributes: name and species. The dog’s name is Buddy, and the species is dog. The cat’s name is Whiskers, and the species is cat.
When defining the function, the species and name of the object were asked to be returned. Thus, when printing the output for the object dog, the species is printed as dog and named Buddy. Similarly, for the object cat, it prints that the species name is cat and named Whiskers.
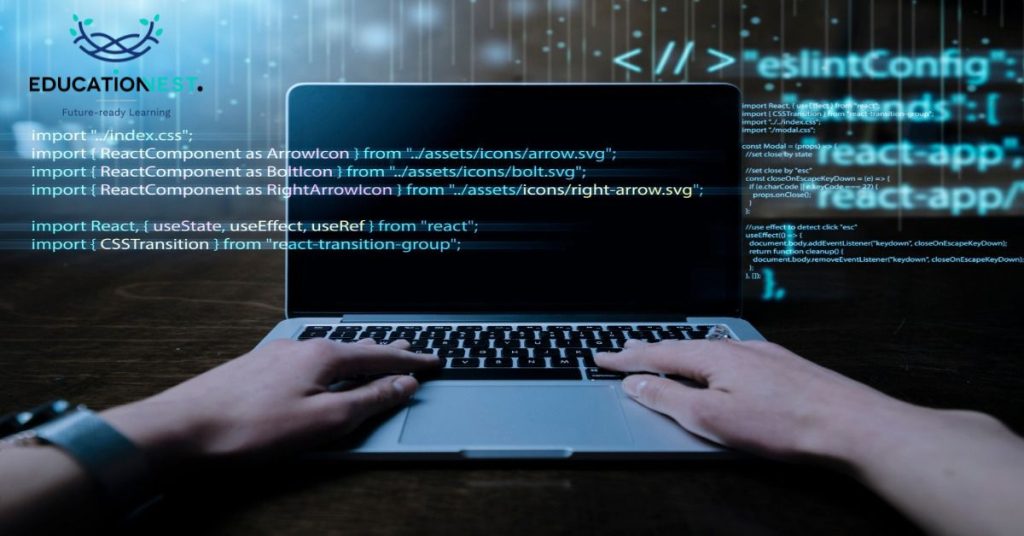
Inheritance
It is a crucial OOP principle and helps create new classes inherited from existing classes. You can define the general attributes of the primary parent class and modify them in the inherited classes. Thus, you can create a hierarchy of classes and model real-world relationships too.
The syntax is:
Class Baseclass:
{Body}
Class Derivedclass:
{Body}
For instance,
class Dog(Animal): #creating an inherited class called Dog of the parent class Animal
def speak(self): #defining a speak function
return f”{self.name} says Woof!”
dog = Dog(“Buddy”, “dog”) #passing the parameters for the base class
print(dog.speak()) # Output: Buddy says Woof!
Since the class Dog is a derived or inherited class, it has the same attributes as the parent class- Animal. It can use the properties defined in the animal. Thus, when you pass the parameters Buddy and dog, it can link it to the attribute- name and species.
As you call the function speak defined in the inherited class, it returns the output accordingly.
Polymorphism
There can be different objects of different classes, yet they can be treated uniformly and redefined for a specific implementation.
For example, you have created animals and passed the parameters of the parent class Animal.
animals = [Dog(“Buddy”, “dog”), Cat(“Whiskers”, “cat”)]
for animal in animals:
print(animal.speak())
The output will be:
Buddy says Woof!
I am a cat named Whiskers.
Thus, even without directly using the derived class Dog or the object Cat, you can access the function speak polymorphically.
Encapsulation
Another important concept in OOP in Python is encapsulation. It ensures controlled interactions and lets you make the data and methods private or public. Also, it helps maintain data security and integrity, preventing accidental modification of information.
For instance, you create a main class BankAccount, with the attributes of owner and balance. Balance is a private attribute.
class BankAccount:
def __init__(self, owner, balance):
self.owner = owner
self.__balance = balance # Private attribute
Now, you define a function called deposit with the parameter amount. If the amount value is more than 0, then the balance attribute will be increased by the deposited amount value. And return the result. Else
def deposit(self, amount):
if amount > 0:
self.__balance += amount
return f”Deposited {amount}. New balance: {self.__balance}”
return “Invalid amount.”
def __str__(self):
return f”Account owner: {self.owner}, Balance: {self.__balance}”
Now, if you define an object called account under the main class BankAccount, with attributes Name as Alica and Balance as 500, you print the value for the account by calling the function deposit, and the amount is 200.
account = BankAccount(“Alice”, 500)
print(account.deposit(200))
As the function will update the balance by increasing the existing balance by the amount, the result will be (200+500)=700.
Output:# Output: Deposited 200. New Balance: 700
But if you try to print the attribute balance for the class account directly, it will show an error as it is a private attribute encapsulated within the main class BankAccount and the function deposit only.
print(account.__balance)
# AttributeError: ‘BankAccount’ object has no attribute ‘__balance’
Read More
Master the Power of Python Programming: Your Ultimate Guide
Snake Game Python Code: How to Build an Addictive Game in Just a Few Lines
Top 10 Python Frameworks You Need to Know for Web Development
Wrapping Up
As discussed, OOP in Python makes modeling real-world applications and relationships easy, thus simplifying complex concepts. Besides, it helps develop clean and reusable code bundles for robust and scalable applications. Therefore, it is essential to master OOP in Python concepts to excel in the IT landscape.
Interested in learning in-demand tools and technologies? Check out the extensive course catalog from Education Nest that can help you with personalized training programs addressing your unique needs. They are the best corporate training providers in India. For more details, reach out today.