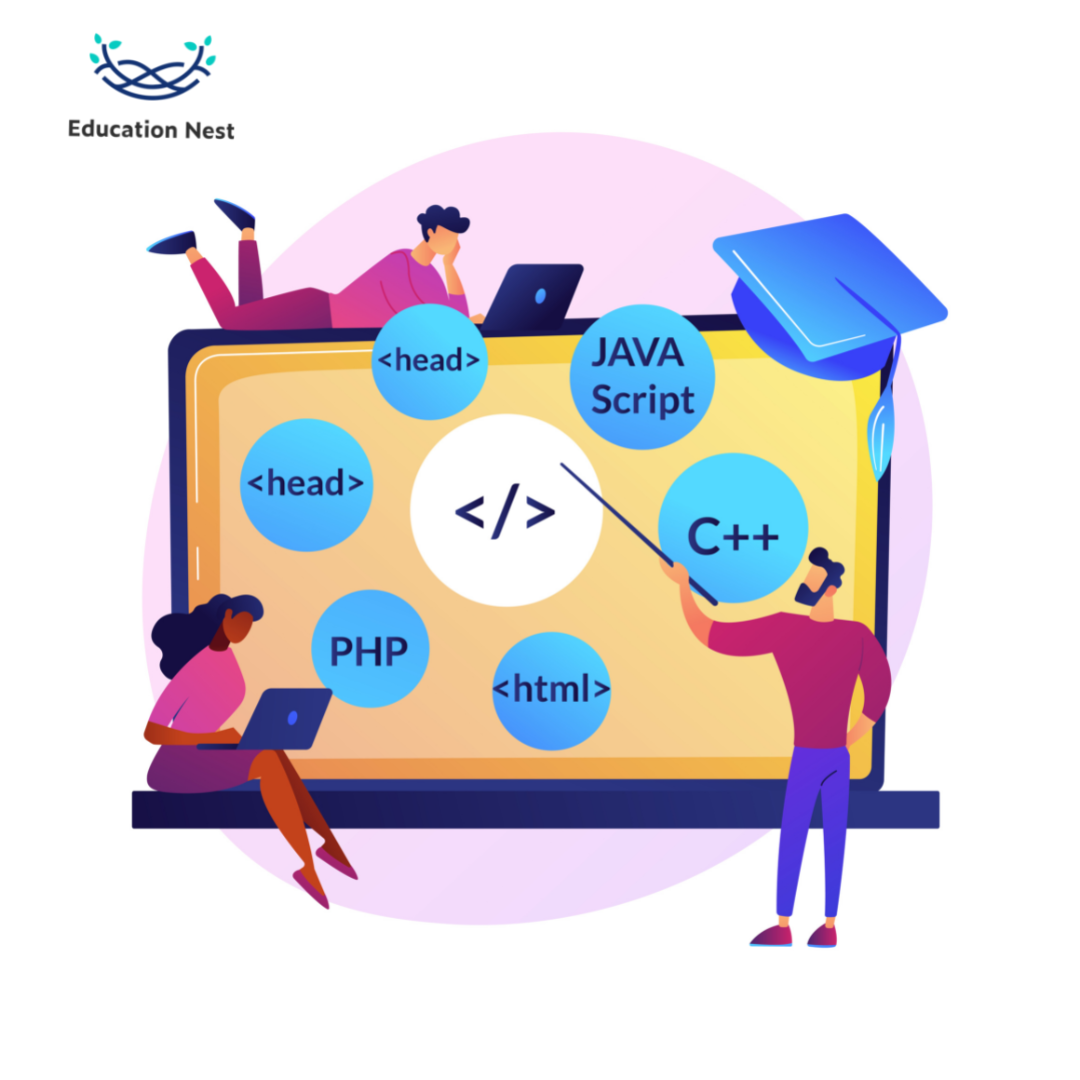
The Java Collections framework lets you store and work with groups of objects in a structured way.
Java Collections enables you to do all kinds of things with data, like searching, sorting, adding, updating, and
removing. It is a set of interfaces and classes that make storing and processing data organizationally easier.
In this article, we will discuss the following:
- Collections in Java
- Collection interface in Java with examples
- Java Collection interview questions
- Collections in Java
The Java Collections API has a lot of support for a wide range of data manipulation tasks, such as storing, retrieving, sorting, editing, and deleting data. The Java Collection Framework is a set of classes and interfaces that may treat multiple Java collections as a single object.
A Java Collection is like a box that holds several objects with the same name and behavior.
What does the word “framework” mean in the Java programming language?
- It’s like buying a ready-made house.
- It takes the place of a group of classes and APIs.
- You’re not forced to do it.
What Is a Collection Framework, exactly?
The Collection framework is a standard way to organize data about groups of things. There are both interfaces
and the classes that use them.
There needs to be a separate infrastructure for collecting money.
Before the Collection Framework (or JDK 1.2), arrays, vectors, and hash tables were the most common ways to organize groups of Java objects
. There was no one place where all these databases could be
accessed simultaneously.
This means that even though the primary goal of all collections is the same, there is no
link between how the collections are put together. Users also need help keeping track of the
many other collection classes since each has its methods, syntax, and constructors.
Let’s see how this works by adding a new item to a hashtable and a vector.
// Java program to demonstrate
// why collection framework was needed
import java.io.*;
import java. Util.*;
class CollectionDemo {
public static void main(String[] args)
{
// Creating instances of the Array,
//Vector and hashtable
int arr[] = new int[] { 1, 2, 3, 4 };
Vector<Integer> v = new Vector();
Hashtable<Integer, String> h = new Hashtable();
// Adding the elements into the
// vector
v.addElement(1);
v.addElement(2);
// Adding the element into the
// hashtable
h.put(1, “geeks”);
h.put(2, “4geeks”);
//Array instance creation requires [],
// while Vector and hashtable require ()
// Vector element insertion requires addElement(),
// but hashtable element insertion requires put()
// Accessing the first element of the
//Array, Vector and hashtable
System.out.println(arr[0]);
System.out.println(v.elementAt(0));
System.out.println(h.get(1));
// Array elements are accessed using [],
// vector elements using elementAt()
// and hashtable elements using get()
}
}
Output:
1
1
Geek
Advantages of Java Collection:
There are many reasons to use Java collections, such as:
- Giving valuable data structures and techniques to reduce the time and effort needed to write code.
- Java’s collections make it possible to create data structures and algorithms that work well and are of high quality. This improves both speed and quality.
- APIs that have nothing to do with each other could share collection interfaces.
- helps people learn about new application programming interfaces more quickly (APIs)
- Lets you use the same data structures and algorithmic building blocks over and over again
How data are set up in a collection’s hierarchy
The collection framework uses several classes and interfaces in the Java.util package. The collection framework has an iterable interface that gives you the iterator to go through all collections in a loop. This interface is built on the primary collection interface, which is the framework’s backbone. The collections that add to this collection interface add to what the iterator can do and what the interface’s methods can do.
First, let’s talk about a class and an interface and how they work. Then, we’ll get into the details of the framework.
A class is a blueprint or prototype that an object is built from. It is a symbol for the Set of properties and operations that all things of a particular type have in common.
An interface is like a class in that it can have its own Set of methods and variables, but the ways in an interface are abstract instead of concrete (only method signature, nobody). Interfaces only tell classes what they should be able to do. In a way, it’s like an outline of the course.
- Collection interface in Java with examples
After getting a general idea of how Java Collections can be used to change data, the next step is to learn more about the interfaces and classes that make up Java Collections, including detailed definitions and examples.
All of the classes in the Collection Framework use the subinterfaces of the Collection Interface. All of the methods in the Collection interface are also in its derived interfaces. These subinterfaces are also called collection types or subtypes. In this group, we have:
List: The Collection interface is the parent of the List interface. List data, which can be any ordered Collection of items, has its unique interface. This also means that it can have information that is already known. This list interface is used by several classes, such as ArrayList, Vector, Stack, etc. Since the List’s implementation is the same for all these subclasses, we can make a list object from any of them. As an example,
List <T> al = new ArrayList<> ();
List <T> ll = new LinkedList<> ();
List <T> v = new Vector<> ();
Where T is the type of the object
Set: A set is a group of things that can’t store the same value more than once because they are not in order. We can use this Collection since we only need to keep track of one copy of each object. Many other classes, such as HashSet, TreeSet, LinkedHashSet, etc., use this set interface. Any derived class can be used to make a set object because they all share the Set’s implementation. As an example,
Set<T> hs = new HashSet<> ();
Set<T> lhs = new LinkedHashSet<> ();
Set<T> ts = new TreeSet<> ();
Where T is the type of object.
Queue: Like a physical queue line, a queue interface uses First In, First Out (FIFO) prioritization to handle requests. This storage interface is for things that must be put away in order. For example, when you book a flight, seats are often given out based on who gets there first. Since this is the case, the ticket will be given to the first person who asks for it. Some examples of these classes are PriorityQueue, Deque, ArrayDeque, etc. Any of these subclasses can be used to make a queue object because they all follow the queue protocol. as an example.
Queue <T> pq = new PriorityQueue<> ();
Queue <T> ad = new ArrayDeque<> ();
Where T is the type of object.
Declaration:
public interface Collection<E> extends Iterable<E>
Here, E is the type of element stored in the Collection.
Example:
// Java program to illustrate Collection interface
import java.io.*;
import java.util.*;
public class CollectionDemo {
public static void main(String args[])
{
// creating an empty LinkedList
Collection<String> list = new LinkedList<String>();
// use add a () method to add elements in the List
list.add(“Geeks”);
list.add(“for”);
list.add(“Geeks”);
// Output the present List
System.out.println(“The list is: ” + list);
// Adding new elements to the end
list.add(“Last”);
list.add(“Element”);
// printing the new List
System.out.println(“The new List is: ” + list);
}
}
Output
The List is: [Geeks, for, Geeks]
The new List is: [Geeks, for, Geeks, Last, Element]
Java Collection Interview Questions
When discussing Java, you can’t leave out the Collection Framework. It’s one of the essential ideas in the language. If you want to be a Java developer, you should know these ideas well before you go to an interview. We’ve reviewed the most common Java Collections interview questions and their answers, which you can use to do well in your following interview.
How do you understand the term “Collection Framework” in Java?
Java’s Collection framework has everything you need to store and manage groups of objects. It makes it easy
for programmers to use data structures and methods for working with already set-up data.
The collection framework is made up of the following parts:
-Interfaces
-Classes
-Algorithm
With all of these classes and interfaces at your disposal, you can search, sort, add, and remove data quickly
and easily.
Give a reason why Collection does not extend the cloneable and serializable interfaces.
The Collection interface in Java tells us what an element is. A part is a group of objects. The order of
pieces in each Collection determines how easily tracking them is. Since this is the case, there is a
reason to improve the cloneable and serializable interfaces.
Write down some essential things the Generic Collection can do for you.
The following are some benefits of Java’s generic Collection:
- Type-checking during compilation is made better.
- Makes it less critical to cast in broad categories
- Allows generic algorithms, which make code easier to read, maintain, and use in other places.
Why is using the properties file a good idea?
The main benefit of using a Java properties file is that you don’t have to recompile the Java class if you change
the values in the file. Because of this, it is mainly used to store things like usernames and passwords that are
likely to change. The application can now be managed quickly and easily.
What does “iterator” mean to you regarding the Java Collection Framework?
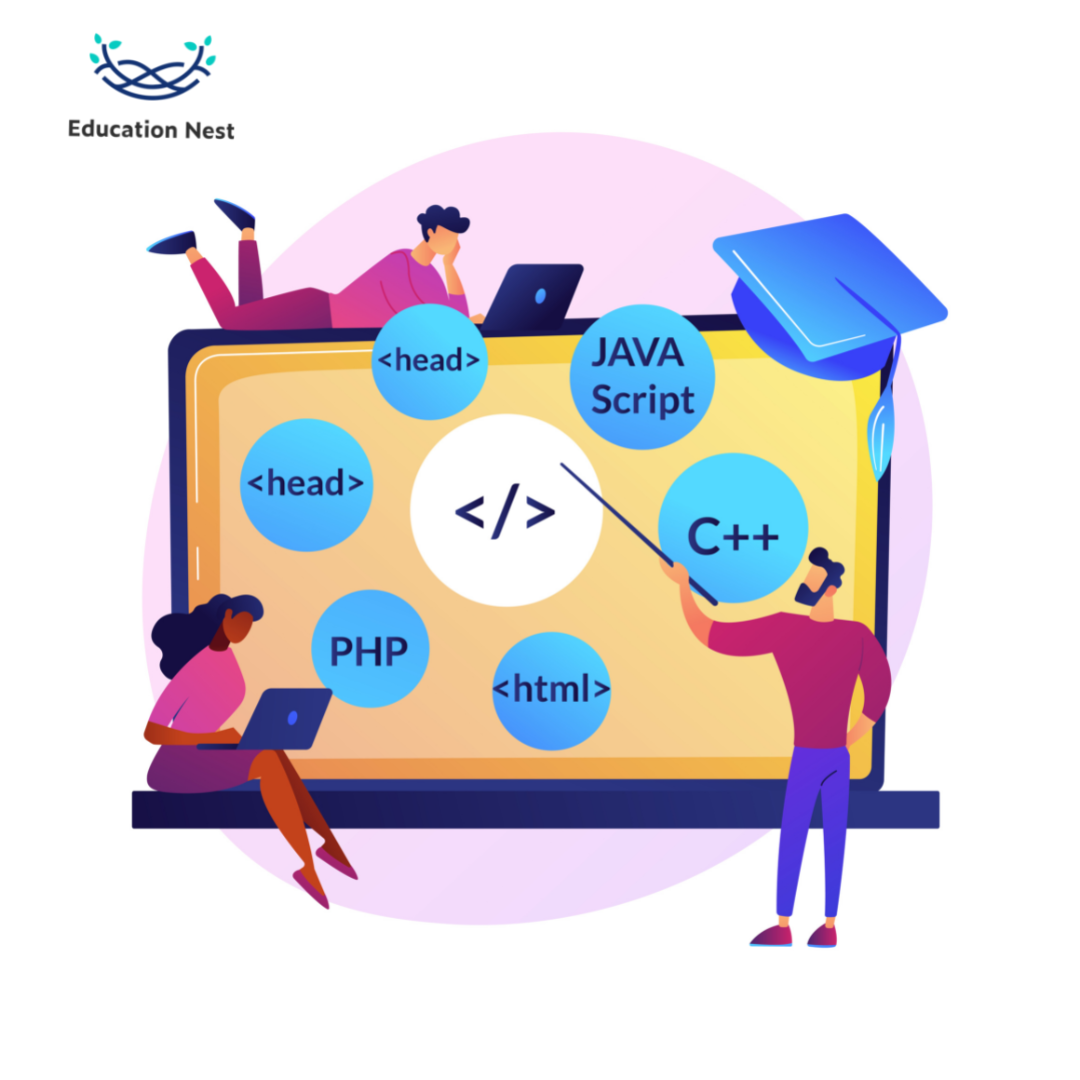
The Iterator interface is part of the Collection framework. It is part of the Java.util package. It’s called a Java
cursor, and its job are to move through a collection.
Some other essential things about the Iterator interface are:
- You can iterate over the items in a collection object, which is why it’s called the “Universal Java Cursor.”
- It can be read and erased.
- Iterator method names that are easy to use
Why do we have to make our own Java equals() function?
One way to tell if two objects are the same is to look at how they first use the equals method. But you must override this method if you want to compare two things based on their properties.
How can we tell in Java what order the objects in a collection are in?
Sorting is accomplished in Java Collections by utilizing the Comparable and Comparator interfaces. The collections are informed by the compareTo() method. The sort() function determines how the elements should be arranged. On the other hand, when the collections are used, the compare() function of the Comparator interface is used to sort the items. The method “sort” (Comparator) is called.
Explain the Set concept and how it is used in the Java Collections framework.
Duplicates are not allowed in a set, which is how it got its name. The primary way that this tool is used is to model
the idea of a mathematical set. There are three different ways to use Set in Java, and each one has its own
Use case.
HashSet’s tree set is the same as LinkedHashSet.
How does the Java HashSet class store its elements?
Java. util. HashSet is part of the Java collection system. It extends AbstractSet and implements the Set interface. A hash table is used by default to create and store unique items. A hashtable is an instance of the HashMap class that uses a hashing process to store data in a hash set. Hashing is the process of converting data into a unique number, which is sometimes called a “hash code.” The hashcode is then used to organize the data related to the key. No one else is needed to change the information key to the hashcode; it all happens independently.
Conclusion
In short, the Java collection framework gives the developer ready-made data structures and algorithms for working with those structures.
After reading this, you should understand the hierarchy, interface, List, queue, and sets, essential parts of the Java collections framework.
We hope you’ve learned much about Java Interview Questions and Answers that will make you stand out from the other candidates.