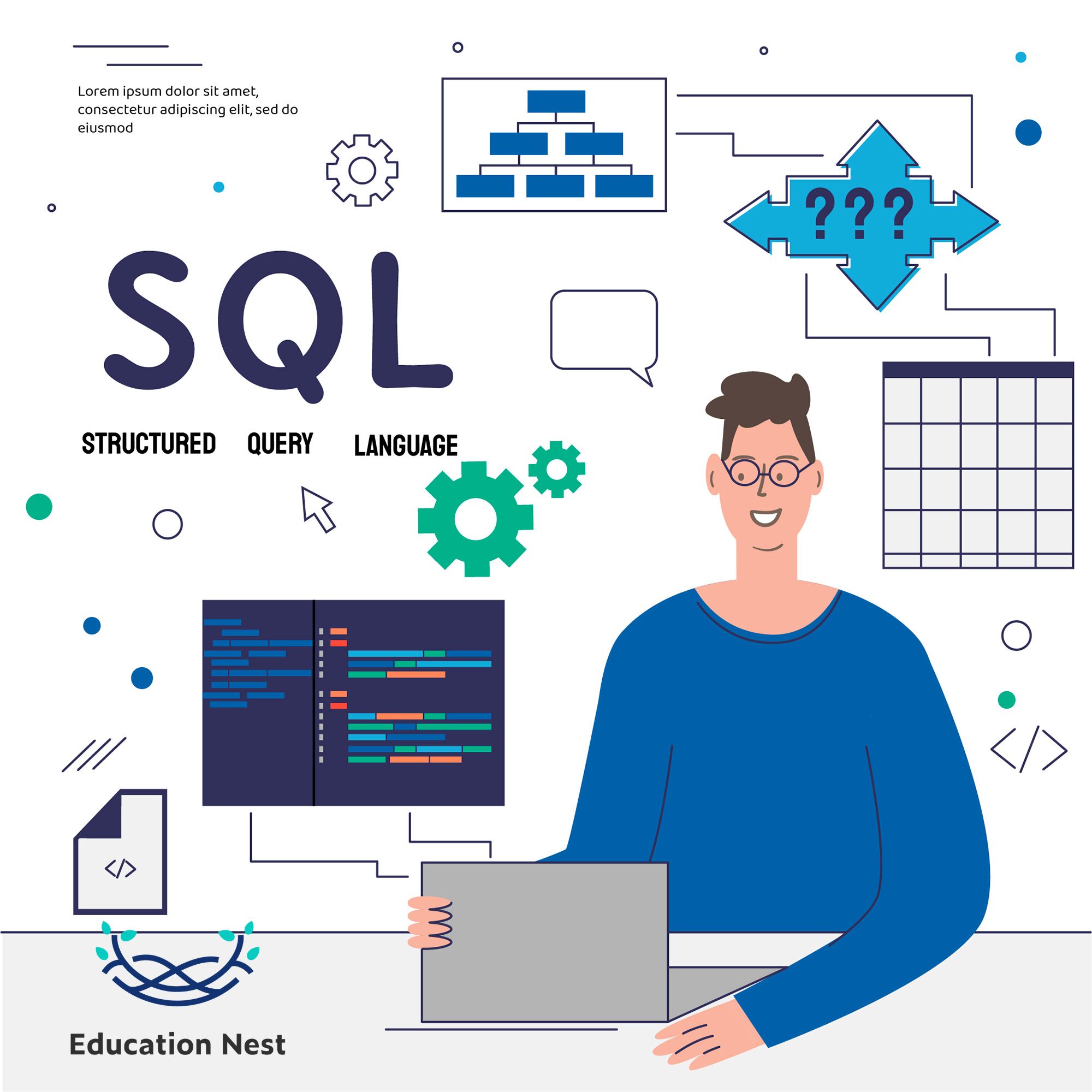
Structured query language (SQL) is a programming language used to store and process information in a relational database. A relational database stores information in table format, with rows and columns representing different data attributes and the different relationships between the data values. There are also a lot of free SQL tutorials online that can help you get better at SQL.
You can use these SQL commands to create a table in a database, add and change a lot of information at once, search through it to find something quickly, or delete a table completely. And that’s how SQL helps you do everything in one place.
In this blog we’ll explain to beginners what SQL commands are and how to use them to ask a database for information or query it. Let’s move forward and start.
MySQL Tutorial: A step-by-step example
MySQL is a popular and free system for managing relational databases. Here is a MySQL tutorial that is easy to understand:
1.Installing MySQL
Before you can use MySQL, you must first install it. You can get MySQL from its official website and set it up by following the steps for your operating system.
2. How to Set Up a Database
To create a database, you can use the following command:
CREATE DATABASE mydatabase;
3. How to Create a Table
To make a table in your database, you can use the following command:
CREATE TABLE mytable (
ID: INT PRIMARY KEY,
name VARCHAR(50),
age INT,
email VARCHAR(50)
4. Inserting Data
You can add information to your table with the following command:
INSERT INTO mytable (id, name, age, email) VALUES (1, ‘John Doe’, 30, ‘john@example.com’);
5.Retrieving Data
You can get information from your table by using the SELECT command.
SELECT * FROM mytable;
This will retrieve all of your table’s rows and columns.
6.Updating Data
You can change the information in your table with the UPDATE command:
UPDATE mytable SET age = 31 WHERE id = 1;
7.Deleting Data
You can get rid of data from your table by using the DELETE command:
DELETE FROM mytable WHERE id = 1;
8.Filtering Data
You can sort data by using the WHERE clause in your SELECT command:
SELECT * FROM mytable WHERE age > 25;
9.Sorting Data
You can use the ORDER BY clause in your SELECT command to sort the data:
SELECT * FROM mytable ORDER BY name;
10.Joining Tables
You can join tables by using the JOIN clause in your SELECT command:
SELECT *
FROM mytable
JOIN myothertable ON mytable.id = myothertable.id;
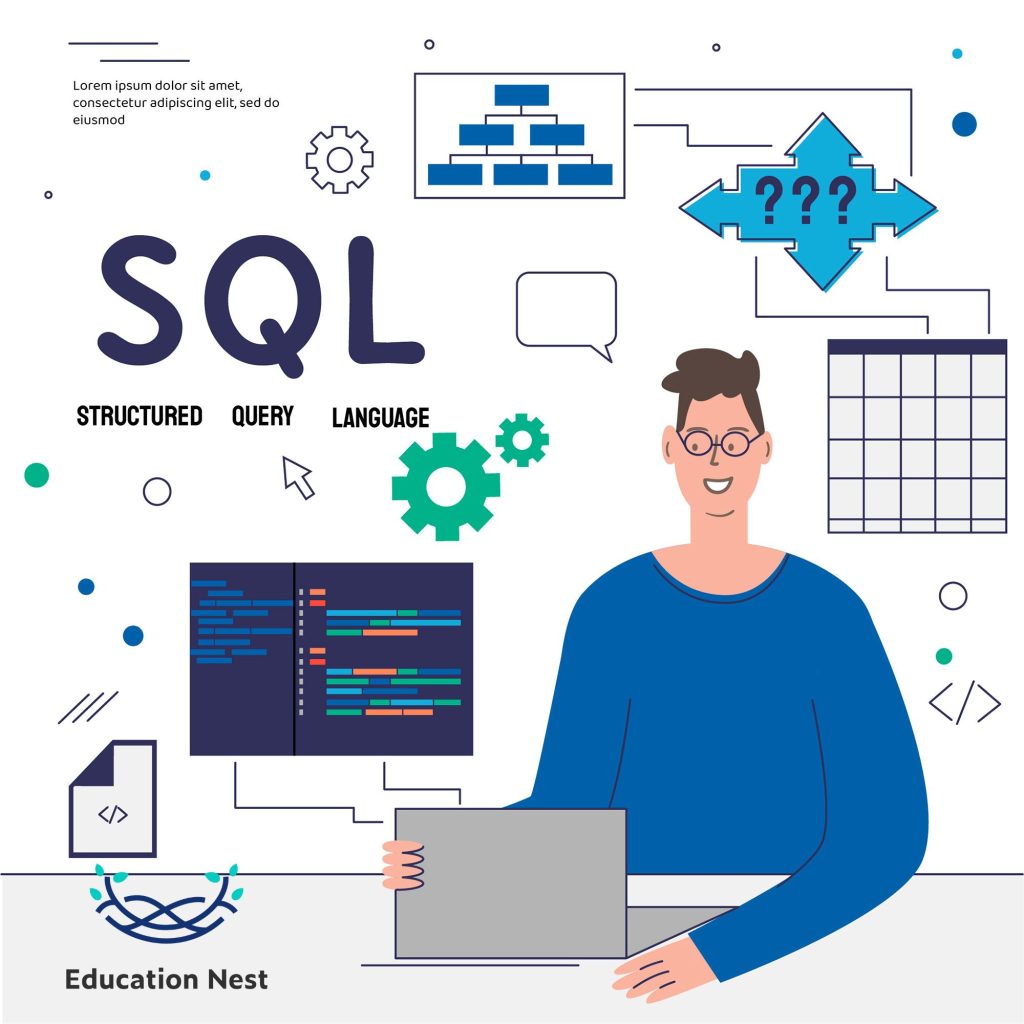
Advanced SQL techniques you can use to improve your skills:
- Subqueries
A subquery is a question that is part of a bigger question. It can be used to find information in one table based on information in another table.
- Joins
Joins are used to combine the results from two or more tables into a single set. There are different kinds of joins, like inner join, left join, right join, and full outer join. This will get the names and phone numbers of everyone in both tables whose ID number is the same.
- Indexing
Indexes are used to make database queries go faster by giving the data a structure that makes it easier to find. An index can be made from one or more columns in a table. This will make an index for the age column in the mytable table.
- Transactions
A transaction is a group of database operations that are done at the same time. Transactions make sure that all actions are either done or undone if something goes wrong.
- Views
The results of a SELECT statement are used to make a view, which is a virtual table. Views can make it easier to understand complicated queries and limit who can see sensitive data.
As you continue to work with databases, you’ll find more ways to use SQL to improve how you manage and look at data.
Also Read: A Practical Guide to Using Order BY and Group BY in SQL for Data Analysis
SQL Queries
The CRUD acronym is used to describe all data operations.
CRUD stands for the four main things we do when we query a database: create, read, update, and delete.
We put information in the database, read or get it out of the database, change or add to it, and, if we want to, get rid of it.
Below, we’ll look at some basic SQL queries and their syntax to get you started.
- SQL CREATE DATABASE statement to create a database named engineering.
- This query creates a new table inside the database.
- It gives the table a name, and the different columns we want our table to have are also passed in.
Some of the most common types are: INT, DECIMAL, DATETIME, VARCHAR, NVARCHAR, FLOAT, and BIT.
- SQL’s ALTER TABLE statement
We can change the table by adding another column to it after we’ve made it.
- The SQL INSERT statement
This is how we add information to tables and create new rows. It’s the C part in CRUD.
In the INSERT “INTO” part, we can choose which columns we want to fill.
The information we want to store goes inside values. This makes a new record in the table, which is a new row.
String values are put in between single quotes when they are inserted.
- SQL SELECT statement
This statement fetches data from the database. It is the R part of CRUD.
The SELECT statement points to the specific column we want to fetch data from that we want shown in the results.
The FROM part determines the table itself.
- SQL WHERE Statement
which allows us to get more specific with our queries.
If we wanted to filter through our engineering table to search for employees that have a specific salary, we would use “where.”
- SQL AND, OR, and BETWEEN operators
These operators allow you to make the query even more specific by adding more criteria to the WHERE statement.
- The AND operator takes two conditions, and both of them must be true for the row to show up in the result.
- The OR operator takes in two conditions, and either one must be true for the row to show up in the result.
- The BETWEEN operator filters out numbers or text that are in a certain range.
We can also use these operators in combination with each other.
A quick review of the key SQL commands will now be given.
- SELECT is a database query that extracts data.
- Update: A database operation that modifies data
- The DELETE command removes data from a database.
- INSERT INTO is a database operation that adds new data.
- The CREATE DATABASE command creates a new database.
- “ALTER DATABASE” modifies a database.
- CREATE TABLE: This command creates a new table.
- ALTER TABLE: changes a table.
- The DROP TABLE command deletes a table from the database.
- CREATE INDEX: This command creates an index (search key).
- Dropping an index eliminates an index.
Conclusion
SQL is a necessary language for anyone who wants to work with data. SQL can help you manage and analyze data well, no matter if you are a data analyst, a database administrator, or a software developer.
With the help of the many free SQL tutorials you can find online, you can learn the basics of SQL syntax and slowly build up your skills to handle more advanced concepts like joins, subqueries, and transactions. By learning SQL, you can make it easier to manage data and find new jobs.