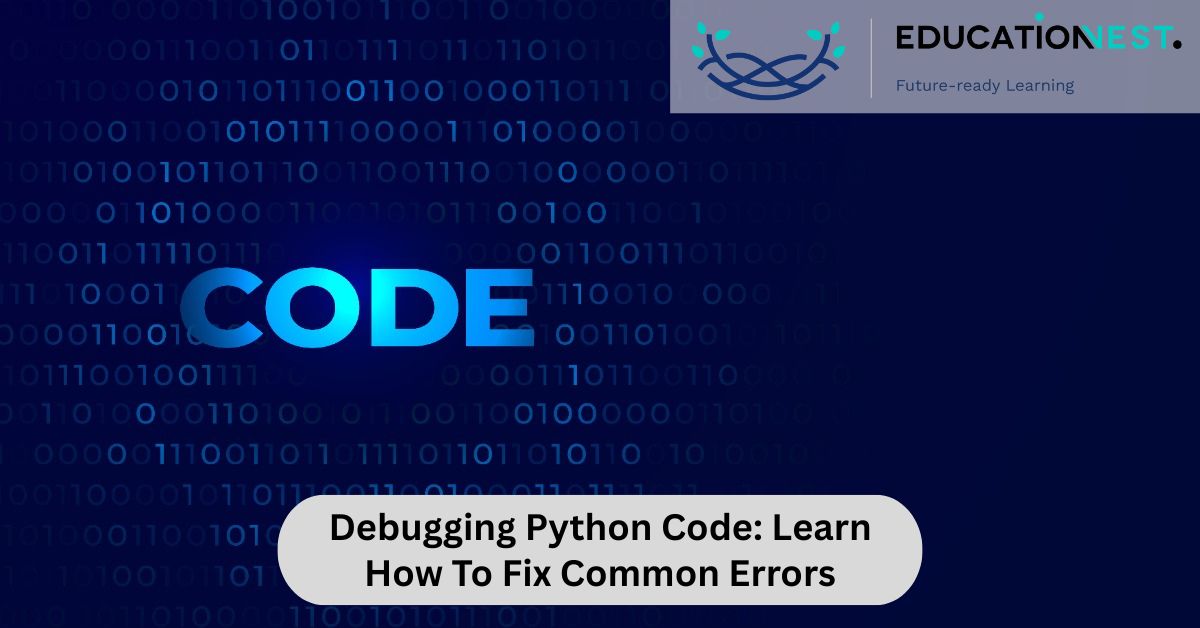
Python’s simplicity and readability make it one of the best programming languages. But its dynamic typing and lack of strict compile-time checks are the culprits. These can lead to unexpected runtime errors. No matter how experienced you are with Python, you will make errors.
Whether you are a beginner writing your first script or a seasoned developer working on a complex application, bugs and exceptions will always be part of the journey. However, understanding why these errors occur is the only thing that makes debugging easier. It is also the only way you learn to write cleaner, more efficient code in the future.
This article talks about the most common Python errors and their solutions.
- Syntax Errors
Python is known for its clean and straightforward syntax. But that does not mean it is immune to errors. You will run into syntax issues at some point. Syntax errors occur when the code does not follow Python’s grammatical rules. These mistakes prevent the program from being executed, as the interpreter cannot convert the code into a runnable form. There are tools that highlight Python syntax errors. These are VS Code, PyCharm, or Jupyter Notebook.
How to Fix Syntax Errors in Python:
- Python shows clear error messages that indicate what went wrong. Read it carefully.
- Check for missing punctuation. Ensure colons (:) are present in loops, conditionals, and function definitions. Verify all kinds of brackets are correctly paired.
- Python relies on indentation for code structure. Correct indentation issues. Your code should follow consistent spacing (usually 4 spaces per indentation level).
- Fix misspelled or missing keywords. Double-check def, if, else, for, while, and print.
- Verify proper use of operators. For comparisons, we use == and not = (assignment).
- Ensure logical (and, or, not) and arithmetic operators are correctly used.
- Avoid illegal characters in variable names. They should start with a letter or underscore and not include spaces or special symbols (except _).
- Use Python auto-linter tools like Black, Flake8, or Pylint. These automatically detect and fix many syntax errors.
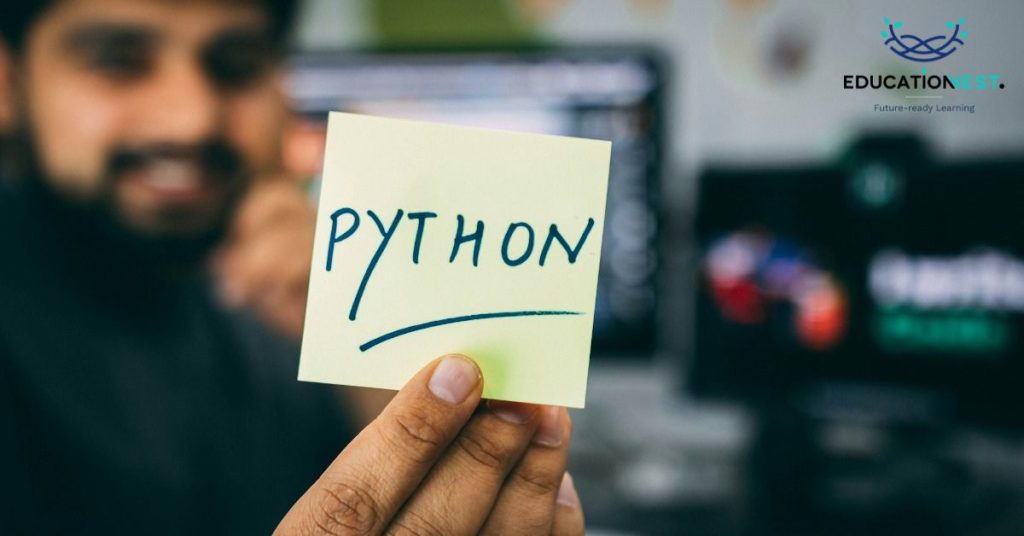
- Logical Errors in Python
Logical errors are the hardest to detect. That’s because they do not cause the program to crash. Neither do they generate error messages. Instead, the program runs but produces incorrect or unexpected results due to flawed logic in the code. The best way to debug logical Python errors is to carefully review, test, and analyze the program’s behavior.
- Print intermediate values to check if variables are holding the expected data throughout the program.
- Use debugging tools (like PDB) to step through code execution and inspect values in real-time.
- Implement tests with Unittest or Pytest to verify the expected output for different inputs. This can catch logic flaws early.
- Break down the code into smaller parts. Test individual blocks of code separately to isolate the issue.
- Using static code analysis tools for Python is another great fix.
- Use assertions to verify conditions that must be true at specific points in the program.
- Check that your loops iterate the expected number of times.
- Double-check that formulas, calculations, and logic are correctly applied.
If you are running a company, it is important to make sure your Python programmers are equipped with all these skills. Everyone should be familiar with common issues and how to fix them. If you are looking for such comprehensive corporate Python training for employees, look no further than EducationNest. They are one of India’s leading corporate training providers, with expert-led courses in various topics that deliver the highest ROIs.
- Runtime Errors in Python
Runtime errors occur when an unexpected condition prevents a program from executing successfully. Syntax errors are detected before the program runs. But runtime errors appear while the code is being executed. These errors can be tricky to debug. Because they often depend on specific input or program state. To fix runtime errors in Python, we need to find the root cause. Then, we need to adjust the code to prevent or handle them effectively.
NameError
A NameError occurs when Python cannot recognize a variable or function because it has not been:
- Defined
- Is misspelled
- is used outside its valid scope.
So you already know how to fix or prevent this error. Just avoid these three mistakes. Use Python debugger tools, such as print statements or PDB, to trace where the issue occurs.
IndexError
An IndexError occurs when attempting to access an element at an invalid index in a list, tuple, or string – typically when the index is out of range. Here’s how to fix it:
- Always check that index values are within the valid range before accessing elements.
- Use Python’s len() function to determine the length of a sequence before indexing.
- Implement try-except blocks to handle potential index-related errors gracefully.
Comprehensive online/offline corporate training programs will teach you all these and more in-depth.
TypeError
A TypeError arises when an operation is performed on an incompatible data type. This can happen when using arithmetic or logical operations on mismatched types or passing incorrect arguments to a function. Here’s how to fix a TypeError in Python:
- Use type annotations to specify the expected data types for variables and function parameters.
- Utilize built-in type-checking functions like instance() to verify types before performing operations.
- Implement unit tests to ensure functions receive and process the correct data types.
AttributeError
An AttributeError is raised when attempting to access an attribute or method that does not exist for a given object. This happens due to misspellings or using the wrong object type. So avoid this mistake. Also, verify that the object type supports the attribute/method before accessing it. You can use hasattr() to check if an attribute exists before attempting to access it.
Read More
How to Master Python Queue: A Comprehensive Guide
Python Variable Types and Their Uses: Everything You Need to Know
How to Create Tuples in Python: A Beginner’s Guide
What is File Handling in Python: The Ultimate Guide
Conclusion
Every error presents a learning opportunity. While Python provides a wealth of built-in tools to identify and handle mistakes, you should also know how to use them. The first step to fixing common Python issues is to know how to interpret error messages. Then, you must know the multiple ways to resolve an issue. More importantly, effective error handling is not just about fixing immediate problems. It is also about preventing future ones to improve overall code reliability.
The ability to troubleshoot and debug effectively is the only way to strengthen your programming teams. If you are looking for comprehensive corporate training in Noida, EducationNest is the best provider for you. They have an enormous number of such programs delivered by the best in the industry. Their courses come with lifetime access and can also be customized as per your team’s specific needs.